Question
USING C ONLY PLEASE In this assignment you will work with structs and fileIO to load in employee records from a file, add/delete/modify them, then
USING C ONLY PLEASE
In this assignment you will work with structs and fileIO to load in employee records from a file, add/delete/modify them, then save them back to the file at the end of the session. While this is not a true database setup, it is a way to persist data from session to session.
Main function
For this assignment you will use the following main method. Please do not change this method at all.
int main() { // Create an array of empty structures with space for at least 20 int max = 20; employee employeeList[max]; // Load in list from file and display // You may assume the file contains not more than max entries printf("Loading... "); int numEmp = loadEmployees(employeeList, "../employees.txt"); displayEmployees(employeeList, numEmp); // Add an employee to end and display printf("Adding... "); numEmp = addEmployee(employeeList, numEmp, max, 56789, "Vaughn", "Susan", "123-456-7890"); displayEmployees(employeeList, numEmp); // Modify an existing employee with a new phone number printf("Modifying... "); modifyEmployee(employeeList, numEmp, 34567, "012-345-6789"); displayEmployees(employeeList, numEmp); // Remove an employee // Note that it should return the new number of employees - either the same or 1 less printf("Removing... "); numEmp = removeEmployee(employeeList, numEmp, 23456); displayEmployees(employeeList, numEmp); // Write the results back to file printf("Saving... "); saveEmployees(employeeList, numEmp, "../employees2.txt"); return 0; }
I would start with the fileIO and display functions. That is loadEmployees, saveEmployees, and displayEmployees. After that you could work on the other functions. I would comment out the parts of the main that don't have completed functions for testing.
FileIO functions
We will be storing our employee info into a .txt file in the following format:
EmpID Last First Phone 12345 Steven Daniel 111-111-1111 23456 Vaughn Alyssa 222-222-2222 34567 Reed Nolan 333-333-3333 45678 Spratt Riley 444-444-4444
So the first thing you need to do is to create this file. The main code above expects the file to be called employees.txt. You can either create this txt file through a program like NotePad or through CLion. This link contains information on how to create a new txt file from CLion (Links to an external site.)Links to an external site.. You should place this txt file in the same folder as your main.c.
Please note that there should be 1 employee record stored per line. And the first line is just header info. So you will need to read in the 4 header strings and just throw them away before you get into the actual employee information. For the employee information, EmpID should be read as an integer and the others (including the phone number with dashes) should be read in as strings. I would suggest using a single fscanf line that reads in all 4 pieces of information at once. Also note that there should not be a newline ( ) at the end of the last employee entry. If there is, it often messes up trying to determine if you have read in all the entries.
The reverse is true when writing back out the information. You should write the header information once at the start and then not include a after the last entry.
A quick refresh on FileIO:
You need to make a variable to hold the file handle:
FILE* inFile = NULL;
You then need to open the file for either reading "r" or writing "w". Note that "w" will wipe out the existing file with that name and replace it completely with what you write. That is why in the main above I saved it to a different filename. Ideally, saving it back to the same name makes the most sense, but during debugging it can get annoying since it keeps replacing your initial file.
inFile = fopen(filename, "r"); if (inFile == NULL) { printf("Could not open file "); }
When reading you use the f version of scanf or printf:
fscanf(inFile, "%d", &temp);
To see if there is an more information in the file you can use the end of file function which returns a boolean:
feof(inFile)
And finally, don't forget to close the file once you are all done reading/writing:
fclose(inFile);
Structs
Obviously we will need a place to store all the employee information in memory once it is read in from the file. We will do this be creating a struct to hold the information for a single employee. This will include fields for id, last name, first name, and phone number. Many of these pieces of information are strings (i.e. char arrays) so make them big enough to hold the info. I would suggest 30 for names.
Note that in the given main, an array of these structures has been created for you. This array is then passed to each of the functions you will be writing and it is your job to fill-in/use/modify this array. Note that the struct must be typedefed to be employee in order for it to work with my given main. Please do not create another array of structures - there should be only the one created in the main and everyone else should just use it.
Other functions
As you can see from the main, the other functions are for displaying, adding, removing and modifying employees.
Displaying the employee list should be obvious.
Adding should add the employee to the end of the list, assuming there is still room in the array. If there is no room then it will just not add the employee. It returns the number of employees because it may or may not add 1 employee depending on space.
Removal should first search for the employee given their id number. If found it should remove them by bumping up all the other entries. If the id can't be found then it just doesn't remove anyone. It also returns the new number of employees now in the list.
Modifying is only currently set up to change an employee's phone number given the id.
Please note that for removal and modification, the first step is to find the slot of the employee given the id. I would highly suggest that you create another function to do this for you. We often call this a helper function. It isn't called directly by the main, but called by the other functions to do parts of their job. I would call this function findEmployeeIndex. It should take the list, number on the list, and target as parameters and simply return the index of the target... or -1 if not found. This will save you from having to write basically the same code in both remove and modify.
Output
If you run the given main on the given employee.txt file, you should get the following output:
--------------------------------------------------
Loading... Employees: 12345 Steven Daniel 111-111-1111 23456 Vaughn Alyssa 222-222-2222 34567 Reed Nolan 333-333-3333 45678 Spratt Riley 444-444-4444 Adding... Employees: 12345 Steven Daniel 111-111-1111 23456 Vaughn Alyssa 222-222-2222 34567 Reed Nolan 333-333-3333 45678 Spratt Riley 444-444-4444 56789 Vaughn Susan 123-456-7890 Modifying... Employees: 12345 Steven Daniel 111-111-1111 23456 Vaughn Alyssa 222-222-2222 34567 Reed Nolan 012-345-6789 45678 Spratt Riley 444-444-4444 56789 Vaughn Susan 123-456-7890 Removing... Employees: 12345 Steven Daniel 111-111-1111 34567 Reed Nolan 012-345-6789 45678 Spratt Riley 444-444-4444 56789 Vaughn Susan 123-456-7890 Saving...
Process finished with exit code 0
--------------------------------------------------
And then obviously a file named employee2.txt should be created that contains all the final employee information.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
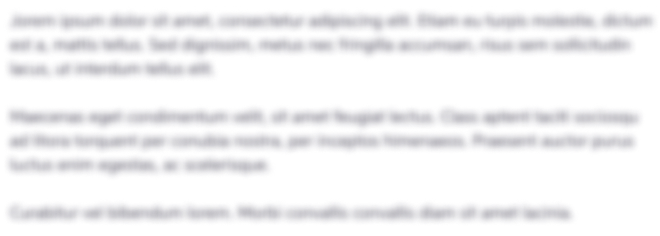
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started