Question
using c++ Stack #ifndef ORDEREDCONTAINER_H #define ORDEREDCONTAINER_H #include linearStructure.h template class OrderedContainer { public: /* The constructor initializes the Container with the structure passed in
using c++
Stack
#ifndef ORDEREDCONTAINER_H
#define ORDEREDCONTAINER_H
#include "linearStructure.h"
template
class OrderedContainer
{
public:
/*
The constructor initializes the Container with the
structure passed in as a parameter. You will have to
make a copy of the LinearStructure as parties outside
of this class may have pointers to it still. The
elements added to the container must be stored in the
object to which dataStructure points.
*/
OrderedContainer(LinearStructure
/*
Copy constructor
*/
OrderedContainer(const OrderedContainer
/*
Overloaded assignment operator.
*/
virtual OrderedContainer
/*
Destructor.
*/
virtual ~OrderedContainer();
/*
Removes an element from the container. See subclasses
for more details.
*/
virtual T remove() = 0;
/*
Returns an element from the container. See subclasses
for more details.
*/
virtual T next() = 0;
/*
Inserts an element into the container. See subclasses
for more details.
*/
virtual void insert(T el) = 0;
/*
Returns true if the container is empty, and false
if the container contains elements.
*/
virtual bool isEmpty();
/*
This function reverses the order of the elements
in the container.
*/
virtual void reverse() = 0;
/*
Returns a pointer to the linear structure in which the elements
are contained.
*/
virtual LinearStructure
protected:
/*
A pointer to an object of type LinearStructure. This
structure should contain the elements inserted into
the container. This member should be initialized
in the constructor.
*/
LinearStructure
};
#include "orderedContainer.cpp"
#endif
#ifndef LINEARSTRUCTURE_H
#define LINEARSTRUCTURE_H
#include
using namespace std;
template
class LinearStructure;
template
ostream& operator<<(ostream&,LinearStructure
template
class LinearStructure
{
public:
/*
Calls print() function to print the data
*/
friend ostream& operator<<
/*
Creates a dynamically allocated deep copy of the object and returns
a pointer to it.
*/
virtual LinearStructure
/*
Virtual destructor
*/
virtual ~LinearStructure(){}
/*
Inserts an element at the given index.
See subclasses for more details.
*/
virtual void insert(int index, T element) = 0;
/*
Removes and returns an element from the index passed
as a parameter. See subclasses for more details.
*/
virtual T remove(int index) = 0;
/*
Returns an element from the index passed
as a parameter. See subclasses for more details.
*/
virtual T get(int index) const = 0;
/*
Returns the first element from the structure.
See subclasses for more details.
*/
virtual const T& getFirst() const = 0;
/*
Returns the last element from the structure.
See subclasses for more details.
*/
virtual const T& getLast() const = 0;
/*
Returns the index of the first element in the structure.
See subclasses for more details.
*/
virtual int getIndexFirst() const = 0;
/*
Returns the index of the last element in the structure.
See subclasses for more details.
*/
virtual int getIndexLast() const = 0;
/*
Returns true if the structure is empty, and false
otherwise.
*/
virtual bool isEmpty() = 0;
/*
Empties out the structure. See subclasses for more details.
*/
virtual void clear() = 0;
protected:
/*
Stream operators are not members of a class and are
therefore not inherited and cannot be overridden as
subclass members. See the implementation of this
class' stream operator below. The print function
in each subclass should be implemented as you would
an overloaded stream operator.
*/
virtual ostream& print(ostream& os) = 0;
};
template
ostream& operator<<(ostream& os,LinearStructure
{l.print(os); return os;}
#endif
Question:
Implement the given functions in the .h file
#ifndef STACK_H
#define STACK_H
#include "orderedContainer.h"
#include "linearStructure.h"
template
class Stack : public OrderedContainer
{
public:
/*
The constructor initializes the stack with the
structure which will contain the elements. Remember to
make a deep copy of the input structure.
*/
Stack(LinearStructure
/*
Copy constructor
*/
Stack(const Stack
/*
Overloaded assignment operator.
*/
Stack
/*
Destructor.
*/
virtual ~Stack();
/*
This function pops and returns the element
on the top of the Stack.
*/
virtual T remove();
/*
This function returns, but does NOT remove, the
element at the top of the stack.
*/
virtual T next();
/*
This function pushes the element sent in as a
parameter onto the top of the Stack.
*/
virtual void insert(T el);
/*
This function reverses the order of the elements
in the stack. For example, if the stack contains the
elements w, x, y and z, where w is at the top of the
stack, then the stack should be z, y, x, w after the
reverse with z now being at the top of the stack.
*/
virtual void reverse();
};
#include "stack.cpp"
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
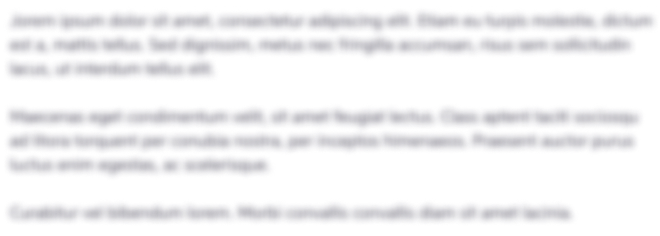
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started