Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using Design Patterns in the Gourmet Coffee System Prerequisites, Goals, and Outcomes Prerequisites: Before you begin this exercise, you need mastery of the following: Object
Using Design Patterns in the Gourmet Coffee System Prerequisites, Goals, and Outcomes Prerequisites: Before you begin this exercise, you need mastery of the following: Objectoriented Programming How to define interfaces How to implement interfaces Design Patterns: Knowledge of the singleton pattern Knowledge of the strategy pattern Goals: Reinforce your ability to use the singleton and strategy patterns Outcomes: You will demonstrate mastery in the following: Producing applications that use the singleton pattern Producing applications that use the strategy pattern Background In this assignment, you will create another version of the Gourmet Coffee System. This version will present the user with four choices: Quit Display sales Plain Text Display sales HTML Display sales XML choice The user will be able to display the sales information in three formats: plain text, HTML or XML Part of the work has been done for you and is provided in the student archive. You will implement the code that formats the sales information. This code will use the singleton and strategy patterns. Description The following class diagram shows how the singleton and strategy pattern will be used in your implementation: Figure Portion of Gourmet Coffee System class diagram Figure Portion of Gourmet Coffee System class diagram The elements of the pattern are: Interface SalesFormatter declares a method called formatSales that produces a string representation of the sales information. Class PlainTextSalesFormatter implements formatSales. Its version returns the sales information in a plaintext format. Class HTMLSalesFormatter implements formatSales. Its version returns the sales information in an HTML format. Class XMLSalesFormatter implements formatSales. Its version returns the sales information in an XML format. Class GourmetCoffee is the context class. It also contains client code. The client code calls: Method GourmetCoffee.setSalesFormatter to change the current formatter Method GourmetCoffee.displaySales to display the sales information using the current formatter In this assignment, you should implement the following interface and classes: SalesFormatter PlainTextSalesFormatter HTMLSalesFormatter XMLSalesFormatter GourmetCoffee a partial implementation is provided in the student archive Complete implementations of the following classes are provided in the student archive: Coffee CoffeeBrewer Product Catalog OrderItem Order Sales Interface SalesFormatter Interface SalesFormatter declares the method that every "Formatter" class will implement. Method: public String formatSalesSales sales Produces a string representation of the sales information. Class PlainTextSalesFormatter Class PlainTextSalesFormatter implements the interface SalesFormatter. This class is implemented as a singleton so a new object will not be created every time the plaintext format is used. Static variable: singletonInstance The single instance of class PlainTextSalesFormatter. Constructor and methods: static public PlainTextSalesFormatter getSingletonInstance Static method that obtains the single instance of class PlainTextsalesFormatter. private PlainTextSalesFormatter Constructor that is declared private so it is inaccessible to other classes. A private constructor makes it impossible for any other class to create an instance of class PlainTextSalesFormatter. public String formatSalesSales sales Produces a string that contains the specified sales information in a plaintext format. Each order in the sales information has the following format: Order number quantity code price quantity code price quantityN codeN priceN Total totalCost where number is the order number. quantityX is the quantity of the product. codeX is the code of the product. priceX is the price of the product. totalCost is the total cost of the order. Each order should begin with a dashed line. The first order in the sales information should be given an order number of the second should be given an order number of and so on Class HTMLSalesFormatter Class HTMLSalesFormatter implements the interface SalesFormatter. This class is implemented as a singleton so a new object will not be created every time the HTML format is used. Static variable: singletonInstance The single instance of class HTMLSalesFormatter. Constructor and methods: static public HTMLSalesFormatter getSingletonInstance Static method that obtains the single instance of class HTMLSalesFormatter. private HTMLSalesFormatter Constructor that is declared private so it is inaccessible to other classes. A private constructor makes it impossible for any other class to create an instance of class HTMLSalesFormatter. public String formatSalesSales sales Produces a string that contains the specified sales information in an HTML format. The string should begin with the following HTML: code: code quantity: quantity price: price code: codeN quantity: quantityN price: priceN code: C quantity: price: code: C quantity: price: code: A quantity: price: code: B quantity: price:
Using Design Patterns in the Gourmet Coffee System
Prerequisites, Goals, and Outcomes
Prerequisites: Before you begin this exercise, you need mastery of the following:
Objectoriented Programming
How to define interfaces
How to implement interfaces
Design Patterns:
Knowledge of the singleton pattern
Knowledge of the strategy pattern
Goals: Reinforce your ability to use the singleton and strategy patterns
Outcomes: You will demonstrate mastery in the following:
Producing applications that use the singleton pattern
Producing applications that use the strategy pattern
Background
In this assignment, you will create another version of the Gourmet Coffee System. This version will present the user with four choices:
Quit
Display sales Plain Text
Display sales HTML
Display sales XML
choice
The user will be able to display the sales information in three formats: plain text, HTML or XML Part of the work has been done for you and is provided in the student archive. You will implement the code that formats the sales information. This code will use the singleton and strategy patterns.
Description
The following class diagram shows how the singleton and strategy pattern will be used in your implementation:
Figure Portion of Gourmet Coffee System class diagram
Figure Portion of Gourmet Coffee System class diagram
The elements of the pattern are:
Interface SalesFormatter declares a method called formatSales that produces a string representation of the sales information.
Class PlainTextSalesFormatter implements formatSales. Its version returns the sales information in a plaintext format.
Class HTMLSalesFormatter implements formatSales. Its version returns the sales information in an HTML format.
Class XMLSalesFormatter implements formatSales. Its version returns the sales information in an XML format.
Class GourmetCoffee is the context class. It also contains client code. The client code calls:
Method GourmetCoffee.setSalesFormatter to change the current formatter
Method GourmetCoffee.displaySales to display the sales information using the current formatter
In this assignment, you should implement the following interface and classes:
SalesFormatter
PlainTextSalesFormatter
HTMLSalesFormatter
XMLSalesFormatter
GourmetCoffee a partial implementation is provided in the student archive
Complete implementations of the following classes are provided in the student archive:
Coffee
CoffeeBrewer
Product
Catalog
OrderItem
Order
Sales
Interface SalesFormatter
Interface SalesFormatter declares the method that every "Formatter" class will implement.
Method:
public String formatSalesSales sales Produces a string representation of the sales information.
Class PlainTextSalesFormatter
Class PlainTextSalesFormatter implements the interface SalesFormatter. This class is implemented as a singleton so a new object will not be created every time the plaintext format is used.
Static variable:
singletonInstance The single instance of class PlainTextSalesFormatter.
Constructor and methods:
static public PlainTextSalesFormatter getSingletonInstance Static method that obtains the single instance of class PlainTextsalesFormatter.
private PlainTextSalesFormatter Constructor that is declared private so it is inaccessible to other classes. A private constructor makes it impossible for any other class to create an instance of class PlainTextSalesFormatter.
public String formatSalesSales sales Produces a string that contains the specified sales information in a plaintext format. Each order in the sales information has the following format:
Order number
quantity code price
quantity code price
quantityN codeN priceN
Total totalCost
where
number is the order number.
quantityX is the quantity of the product.
codeX is the code of the product.
priceX is the price of the product.
totalCost is the total cost of the order.
Each order should begin with a dashed line. The first order in the sales information should be given an order number of the second should be given an order number of and so on
Class HTMLSalesFormatter
Class HTMLSalesFormatter implements the interface SalesFormatter. This class is implemented as a singleton so a new object will not be created every time the HTML format is used.
Static variable:
singletonInstance The single instance of class HTMLSalesFormatter.
Constructor and methods:
static public HTMLSalesFormatter getSingletonInstance Static method that obtains the single instance of class HTMLSalesFormatter.
private HTMLSalesFormatter Constructor that is declared private so it is inaccessible to other classes. A private constructor makes it impossible for any other class to create an instance of class HTMLSalesFormatter.
public String formatSalesSales sales Produces a string that contains the specified sales information in an HTML format.
The string should begin with the following HTML:
code: code
quantity: quantity
price: price
code: codeN
quantity: quantityN
price: priceN
code: C
quantity:
price:
code: C
quantity:
price:
code: A
quantity:
price:
code: B
quantity:
price:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
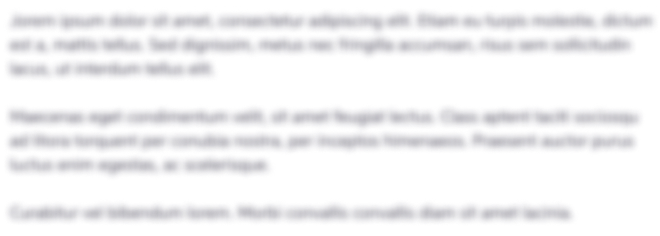
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started