Question
Using Dr Java Objective: Write a class that represents a person in a simple way First download the driver and put it in your project
Using Dr Java
Objective:
Write a class that represents a person in a simple way
First download the driver and put it in your project
DO NOT ALTER THE DRIVER!
Write a class file called Vehicle
Some of the attributes are
Manufacturers name Number of Cylinders (must be greater than 0) Owners name
Create the following Constructors
Default sets everything to default values Parameterized Constructor
Check for valid values
Accessors and Mutators for each variable
MAKE SURE THE MUTATORS CHECK FOR VALID VALUES!
Create the following Methods
equals takes in another instance of a Vehicle and returns true only if the names and the number of cylinders are equal toString returns a String that contains the Manufacturers name, number of cylinders, and the owners name
Write another class Truck which is a Vehicle
Some of the attributes of Truck are
Load capacity: a nonnegative number of tons represented by a decimal number Towing capacity: a nonnegative number of tons represented by a decimal
Create the following constructors
Default sets everything to default values
This includes calling the Vehicles default constructor
Parameterized Constructor
This must also take in via parameter the manufacturers name, number of cylinders, and the owners name in addition to the load and towing capacity.
Accessors and Mutators for each variable
MAKE SURE THE MUTATORS CHECK FOR VALID VALUES!
Create the following methods
equals This should override the vehicles equals method return true if all the properties of vehicle and truck are equal toString This should also override the vehicles toString method and also return the Vehicles toString along with the towing and load capacity
Write another class Car which is a Vehicle
Some of the attributes of Truck are
Gas Mileage: a nonnegative number of gallons represented by a decimal number Number of passengers: a nonnegative number of passengers represented by a whole number
Create the following constructors
Default sets everything to default values
This includes calling the Vehicles default constructor
Parameterized Constructor
This must also take in via parameter the manufacturers name, number of cylinders, and the owners name in addition to the load and towing capacity.
Accessors and Mutators for each variable
MAKE SURE THE MUTATORS CHECK FOR VALID VALUES!
Create the following methods
equals This should override the vehicles equals method return true if all the properties of vehicle and car are equal toString This should also override the vehicles toString method and also return the Vehicles toString along with the gas mileage and number of passengers
The Driver
import java.util.Scanner;
//A class that keeps a fleet of different types of vehicles
public class FleetOfVehicles {
//An array of vehicles
private Vehicle[] fleet;
public static final int MAX_VEHICLES = 100;
public FleetOfVehicles()
{
fleet = new Vehicle[MAX_VEHICLES];
}
public Vehicle[] getFleet()
{
return this.fleet;
}
//Adds a new vehicle to the first empty spot in the fleet array
public void addVehicle(Vehicle aV)
{
for(int i=0;i { if(fleet[i] == null) { fleet[i] = aV; return; } } //If it reaches here the array is full System.out.println("The fleet of vehicles is full!"); } //Removes a vehicle. It searches through the array for a vehicle that is equal to //the parameter and once it is found that value is set to null public void removeVehicle(Vehicle aV) { for(int i=0;i { if(fleet[i] != null && fleet[i].equals(aV)) { fleet[i] = null; return; } } //If it reaches here then the vehicle was not found System.out.println("The vehicle was not found"); } //A static keyboard to be used throughout the frontend static Scanner keyboard; //Entry point of the program public static void main(String[] args) { keyboard = new Scanner(System.in);//Construct the keyboard System.out.println("Welcome to the fleet manager"); FleetOfVehicles fOfV = new FleetOfVehicles();//Creates a new instance of the FleetOfVehicles to be used boolean quit = false; while(!quit)//Runs until the user quits { printOptions(); int pick = keyboard.nextInt(); keyboard.nextLine(); switch(pick) { case 1: //Add vehicle fOfV.addVehicle(makeAVehicleDialog()); break; case 2: //Remove vehicle fOfV.removeVehicle(makeAVehicleDialog()); break; case 9: quit = true; break; default: System.out.println("Invalid input"); } System.out.println("The Fleet currently"); printFleet(fOfV); } System.out.println("Goodbye"); } //Displays the options to the user public static void printOptions() { System.out.println("Enter 1: to add a Vehicle Enter 2: to remove a Vehicle Enter 9 to quit"); } //Returns an instance of a vehicle based on user input public static Vehicle makeAVehicleDialog() { Vehicle retV; int pick = 0; System.out.println("Enter 1: if it is a car Enter 2: if it is a truck Enter 3: if it is unclassified"); pick = keyboard.nextInt(); keyboard.nextLine(); while(pick != 1 && pick != 2 && pick != 3) { System.out.println("Invalid choice pick again"); pick = keyboard.nextInt(); keyboard.nextLine(); } System.out.println("Enter the manufacturer's name"); String manuName = keyboard.nextLine(); System.out.println("Enter the number of cylinders"); int cylinders = keyboard.nextInt(); keyboard.nextLine(); System.out.println("Enter the owner's name"); String ownersName = keyboard.nextLine(); switch(pick) { case 1://Constructing a car System.out.println("Enter the car's gas mileage"); double mileage = keyboard.nextDouble(); keyboard.nextLine(); System.out.println("Enter the number of passengers"); int passengers = keyboard.nextInt(); keyboard.nextLine(); retV = new Car(manuName,cylinders,ownersName,mileage,passengers); break; case 2://Constructing a truck System.out.println("Enter the truck's load capacity"); double loadCap = keyboard.nextDouble(); keyboard.nextLine(); System.out.println("Enter the truck's towing capacity"); double towCap = keyboard.nextDouble(); keyboard.nextLine(); retV = new Truck(manuName,cylinders,ownersName,loadCap,towCap); break; default: retV = new Vehicle(manuName,cylinders,ownersName); } return retV; } public static void printFleet(FleetOfVehicles fV) { for(Vehicle v : fV.getFleet()) { if(v == null) continue; System.out.println(v); System.out.println(); } } } Example Dialog: Welcome to the fleet manager Enter 1: to add a Vehicle Enter 2: to remove a Vehicle Enter 9 to quit 1 Enter 1: if it is a car Enter 2: if it is a truck Enter 3: if it is unclassified 1 Enter the manufacturer's name Nissan Enter the number of cylinders 6 Enter the owner's name JJ Enter the car's gas mileage 29 Enter the number of passengers 5 The Fleet currently Manufacturer's Name: Nissan Number Of Cylinders: 6 Owner's Name: JJ Gas Mileage: 29.0 Number of Passengers: 5 Enter 1: to add a Vehicle Enter 2: to remove a Vehicle Enter 9 to quit 1 Enter 1: if it is a car Enter 2: if it is a truck Enter 3: if it is unclassified 2 Enter the manufacturer's name Chevy Enter the number of cylinders 8 Enter the owner's name Eddie Enter the truck's load capacity 1 Enter the truck's towing capacity 2 The Fleet currently Manufacturer's Name: Nissan Number Of Cylinders: 6 Owner's Name: JJ Gas Mileage: 29.0 Number of Passengers: 5 Manufacturer's Name: Chevy Number Of Cylinders: 8 Owner's Name: Eddie Towing Capacity: 2.0 Load Capacity: 1.0 Enter 1: to add a Vehicle Enter 2: to remove a Vehicle Enter 9 to quit 1 Enter 1: if it is a car Enter 2: if it is a truck Enter 3: if it is unclassified 3 Enter the manufacturer's name Ford Enter the number of cylinders 6 Enter the owner's name Bob The Fleet currently Manufacturer's Name: Nissan Number Of Cylinders: 6 Owner's Name: JJ Gas Mileage: 29.0 Number of Passengers: 5 Manufacturer's Name: Chevy Number Of Cylinders: 8 Owner's Name: Eddie Towing Capacity: 2.0 Load Capacity: 1.0 Manufacturer's Name: Ford Number Of Cylinders: 6 Owner's Name: Bob Enter 1: to add a Vehicle Enter 2: to remove a Vehicle Enter 9 to quit 2 Enter 1: if it is a car Enter 2: if it is a truck Enter 3: if it is unclassified 2 Enter the manufacturer's name Chevy Enter the number of cylinders 8 Enter the owner's name Eddie Enter the truck's load capacity 1 Enter the truck's towing capacity 2 The Fleet currently Manufacturer's Name: Nissan Number Of Cylinders: 6 Owner's Name: JJ Gas Mileage: 29.0 Number of Passengers: 5 Manufacturer's Name: Ford Number Of Cylinders: 6 Owner's Name: Bob Enter 1: to add a Vehicle Enter 2: to remove a Vehicle Enter 9 to quit 9 The Fleet currently Manufacturer's Name: Nissan Number Of Cylinders: 6 Owner's Name: JJ Gas Mileage: 29.0 Number of Passengers: 5 Manufacturer's Name: Ford Number Of Cylinders: 6 Owner's Name: Bob Goodbye
Step by Step Solution
There are 3 Steps involved in it
Step: 1
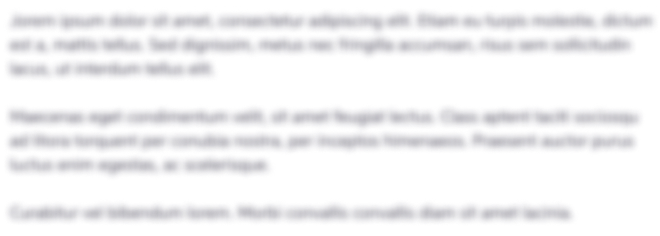
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started