Question
Using ECLIPSE JAVA I an creating a java program where user inputs key words for a song/artist and the program provides songs and artists that
Using ECLIPSE JAVA I an creating a java program where user inputs key words for a song/artist and the program provides songs and artists that correspond (provide the end-user with the functionality to inquire about a song or a singer). Below is my code but I am stuck on the JSONObject and JSONArray part. Do I make two json files within my project in Eclipse? Or can I put the values of the JSON within my code already? If someone can show me how to build these JSON parts...? --HERE--
import java.io.IOException;
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.util.Iterator;
import java.util.Scanner;
import org.json.JSONArray;
import org.json.JSONObject;
import java.net.URLEncoder;
public class MusicGenie {
public static void parseObject(JSONObject json, String key) {
//Here the program is converting the string to filter results
//Several key words create the information, the name itself is not unique here
String result = json.getString(key).toString();
//Demonstration of key words usage to get information
if(result.contains("hub") || result.contains("apple"))
{
}
else
{
System.out.println(json.get(key));
}
}
//we are searching within the JSON file for the words provided
public static void getKey(JSONObject json, String key)
{
boolean exists = json.has(key);
Iterator keys;
String nextKey;
//Loop added for the key that follows
if(!exists) {
keys = json.keys();
while(keys.hasNext())
{
nextKey = (String)keys.next();
try
{
if(json.get(nextKey) instanceof JSONObject)
{
if(exists == false)
{
getKey(json.getJSONObject(nextKey), key);
}
}
else if (json.get(nextKey) instanceof JSONArray)
{
JSONArray jsonarray =
json.getJSONArray(nextKey);
for(int i=0; i
{
String jsonarrayString =
jsonarray.get(i).toString();
JSONObject innerJSON = new
JSONObject(jsonarrayString);
//Using other JSON we loop again object until key is found
if(exists == false) {
getKey(innerJSON, key);
}
}
}
}
catch (Exception e)
{
// Handling exception here
}
}
//Printing output if key is present (ParseObject)
}
else
{
parseObject(json,key);
}
}
public static void main(String[] args) throws IOException,
InterruptedException
{
//Giving the user an option to begin again for wrong input
String restart;
restart = "y";
String end = "";
String option = "";
Scanner input = new Scanner(System.in);
Scanner options = new Scanner(System.in);
Scanner rerun = new Scanner(System.in);
do
{
//Here we are scanning for user input
//while loop to rerun the program until there is a valid selection
while(restart.equals("y"))
{
System.out.println("--------------------------------");
System.out.println("Do you want to search for a Song or Artist? ");
option = options.nextLine();
option = option.toLowerCase();
if(option.contains("artist"))
{
System.out.println("Enter a Artist to search: ");
restart="n";
}
else if(option.contains("song"))
{
System.out.println("Enter a Song to search: ");
restart="n";
}
else
{
System.out.println("Please enter a valid selection!!");
restart = "y";
}
}
String searchInput = input.nextLine();
String search = searchInput.toLowerCase();
//Showing user their choice with the song or artist printed
if(option.toLowerCase().contains("artist"))
{
System.out.println("You entered the artist: " + search);
System.out.println("--------------------------------");
}
else if(option.toLowerCase().contains("song"))
{
System.out.println("You entered the song: " + search);
System.out.println("--------------------------------");
}
//URL search
search = URLEncoder.encode(search, "UTF-8");
//Print test to ensure correct additions
//System.out.println(search);
//Calling the API here
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://shazam.p.rapidapi.com/search?term=" + search + "&locale=en-US&offset=0&limit=5"))
.header("x-rapidapi-key","2293ae3979mshfbd05aef41c719cp17517fjsn3d64074fb333")
.header("x-rapidapi-host", "shazam.p.rapidapi.com")
.method("GET", HttpRequest.BodyPublishers.noBody())
.build();
HttpResponse response =
HttpClient.newHttpClient().send(request, HttpResponse.BodyHandlers.ofString());
//Here we are parsing the data
try
{
String inputJson = response.body();
JSONObject inputJSONObject = new JSONObject(inputJson);
//testing print again to check for parsing to string
// In order to find what keys are left to seek (lack of format)
//System.out.println(inputJson);
if (inputJson.equals("{}"))
{
System.out.println("No information returned for " + search);
}
else if(option.contains("song"))
{ //Outputting tracks/artist
System.out.println("Artist(s): ");
getKey(inputJSONObject, "name");
//searching for name key for artist name to be outputted
//Keeping in mind we cannot directly use name, we filter
}
else if(option.contains("artist"))
{
System.out.println("Top tracks: ");
getKey(inputJSONObject, "title");
//searching for title key for song name to be outputted
}
}
catch (Exception e)
{
System.out.println("Invalid Search! Please start again.");
restart = "y";
continue;
}
//Making sure the choice is valid
do
{
System.out.println("--------------------------------");
System.out.println("Would you like to search again? (y/n):");
end=rerun.nextLine();
if(end.toLowerCase().contains("n"))
{
System.out.println("Thank you for using the Shazam API!");
end = "n";
restart = "n";
System.exit(0);
}
else if(!end.toLowerCase().contains("y") && !
end.toLowerCase().contains("n"))
{
System.out.println("Please enter y/n !");
end = "y";
}
else if(end.toLowerCase().equals("y"))
{
restart = "y";
end = "n";
}
}
while(end.toLowerCase().contains("y"));
}
while(restart.toLowerCase().contains("y"));
//closing scanner
input.close();
options.close();
rerun.close();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
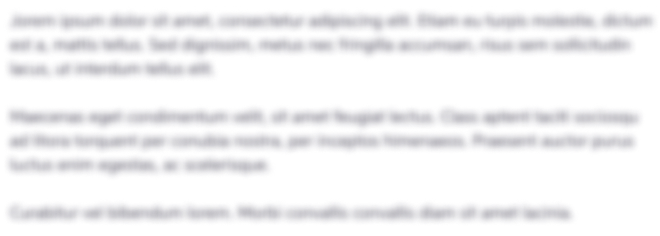
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started