Question
using java An GYM center asked you to help them in storing, deleting and retrieving their current customers information. Where each customer in the GYM
using java
An GYM center asked you to help them in storing, deleting and retrieving their current customers information. Where each customer in the GYM has a special id where first 4 digits are representing the year the customer joined, and the other 4 digits are presenting the customer unique id.
Based on your knowledge in data structure, you decided to use trees, and singly linked lists to get advantages of those structures together in building this system.
Where each tree node key will be the year of joining the GYM, and the data of the tree node will be the singly linked list that contains all the customers who joined the GYM at that year.
Each customer has the following information: id, name, and an arraylist of all GYM_courses he/she joined.
Each GYM_course has its id, name, price, and level (easy-medium-hard).
You need to create the classes Customer, and GYM_Course
You need to use the singly linked list and Tree classes that were covered in the lab, and build a new class called, GYM that has the following methods:
a-insert(Customer) to insert a new customer. It should be inserted based on his id in a tree node that has singly linked list represents customers joined the GYM in that year, if no node created yet for that year (i.e. this is the first customer) then a new node should be created, and inserted in the list.
b- search(id) it returns a customer with that id if the customer is found, otherwise the method should return null.
c- delete(id ): it deletes the customer information with the passed id and returns true. Otherwise, returns false if there is no customer with that id. Note that if that customer is the only one in that tree node, then the tree node should be also deleted.
d- addGYM_course (id, GYM_course) the method will receive customers id and a GYM course, it adds that course to the customers arraylist of courses and returns true, if the customer does not exist, then the method returns false.
e- TotalBenefit (year): which returns the total benefit the GYM center received from all customers joined the center in a certain year. The total benefit will the total of all prices of GYM courses for all customers joined in that year. If no customers joined the GYM center in that year, then the method should return zero.
f- PrintCustomersPerGYMCourse (GYM_Course_id):which prints all the customers (ids and names) who registered in the passed GYM_Course based on its id. if the none of the were registered in the passed GYM_Course based on its id then inform the user about that.
g- PirntCustomers(): which prints all customers (ids and names), the customers should be printed based on their years of joining the center in an descending order, for example first print all customers who joined the center in 2021, then 2020,2019, etc.
Test your structure in a test application (contains main method).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
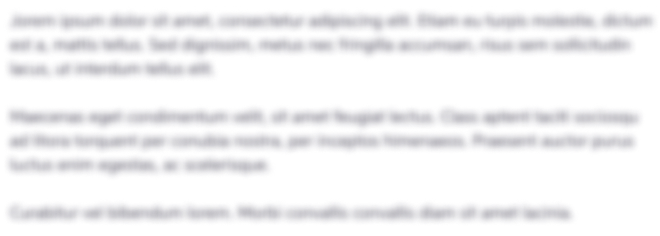
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started