Question
USING JAVA CODE THE FOLLOWING TO WORK WITH EMPLOYEE.JAVA; Part 1: Code Hourly Employee Create a new java file called HourlyEmployee.java Reference the following UML
USING JAVA CODE THE FOLLOWING TO WORK WITH EMPLOYEE.JAVA;
Part 1: Code Hourly Employee
Create a new java file called HourlyEmployee.java
Reference the following UML Diagram for what to code:
Notes on Methods:
Constructor - The constructor accepts all that an employee requires as well as a double for wage, and sets hoursWorked to 0.0.
toString - Overrides the Employee toString, but calls the super.toString and adding "Wage: " + wage and "Hours Worked: " + hoursWorked at the end each on their new line. (This is basically what we had setup for last iteration of the Employee class). Use the currency NumberFormat object on the wage variable.
Name: [firstName] [lastName] ID: [employeeNum] Department: [department] Title: [jobTitle] Wage: [wage] Hours Worked: [hoursWorked]
increaseHours() - This method adds 1 hour to hoursWorked variable
increaseHours(double h) - this method adds the value sent(h) to hoursWorked variable. This should also check so that we don't add negative hours to the employee.
Note: this was our addHours() methods from before
resetWeek() - sets hoursWorked to 0.0
annualRaise() - give a wage increase of 5%
holidayBonus() return the amount of pay for working 40 hours (40 * wage)
calculateWeeklyPay() - Return amount earned in the week using wage and hoursWorked. However, any hours worked over 40 is considered overtime, so you need to calculate those extra hours as one and a half of the wage (1.5 * wage) * (hours - 40). You will probably need an if statement or do some math with modulus to calculate the hours over 40.
if hours > 40: pay = 40 * payrate + (1.5 * payrate) * (hours-40) else: pay = payrate * hours
setPay(double pay) - should change the wage variable to the amount sent.
Part 2: Code Salary Employee:
Create a new java file called SalaryEmployee.java
Reference the following UML Diagram for what to code:
Notes on Methods:
Constructor - The constructor accepts all that an employee requires as well as a double for salary.
toString - Overrides the Employee toString, but calls the super.toString and adding "Salary: " + salary at the end of each on their own new line. Use the NumberFormat currency object on the salary variable.
Name: [firstName] [lastName] ID: [employeeNum] Department: [department] Title: [jobTitle] Salary: [salary]
calculateWeeklyPay() - returns the amount earned in the week by taking the salary and dividing it by 52 (the number of weeks in a year)
annualRaise() - Salary increased by 6.25%
holidayBonus() - Returns 3.365% of salary
resetWeek() - does nothing, but you must define this so leave it blank.
setPay(double pay) - should change the salary variable to the amount sent.
Part 3: Code Commission Employee
Create a new java file called CommissionEmployee.java
Reference the following UML Diagram for what to code:
Notes on Methods:
Constructor - The constructor accepts all that an employee requires as well as a double for rate (in decimal form so 3.5% would be sent as .035). This also sets the sales to 0.0
toString - Overrides the Employee toString, but calls the super.toString, adding "Rate: " + rate and "Sales: " + sales each on a new line. Use the percent and currency NumberFormat objects on rate and sales respectively.
Name: [firstName] [lastName] ID: [employeeNum] Department: [department] Title: [jobTitle] Rate: [rate] Sales: [sales]
calculateWeeklyPay() - Retursn the rate * sales
annualRaise() - Rate percentage increased by .002. example if rates is 3.5% it would be .035 + .002 so .037 or 3.7%
holidayBonus() - no bonus, return 0.
resetWeek() - reset sales to 0.0
increaseSales() - adds 100 to sales
increaseSales(double s) - adds the value sent (s) to sales. Again we shouldn't have negative sales add so make sure that the value sent is positive before adding.
setPay(double pay) - should change the rate variable to the amount sent
MAKE SURE THEY ALL WORK WITH EMPLOYEE.JAVA:
Employee.java:
import java.text.NumberFormat; import java.util.ArrayList; public abstract class Employee { private String firstName; private String lastName; private int employeeNum; private String department; private String jobTitle; private ArrayListemergencyContact; protected static NumberFormat currency = NumberFormat.getCurrencyInstance(); protected static NumberFormat percent = NumberFormat.getPercentInstance(); static { percent.setMaximumFractionDigits(3); } public abstract double calculateWeeklyPay(); public abstract void resetWeek(); public abstract void annualRaise(); public abstract double holidayBonus(); public Employee(String fn, String ln, int en, String dept, String job) { firstName = fn; lastName = ln; employeeNum = en; department = dept; jobTitle = job; emergencyContact = new ArrayList(); } @Override public boolean equals(Object obj) { if (obj == this) { return true; } if (!(obj instanceof Employee)) { return false; } Employee emp = (Employee) obj; return employeeNum == emp.employeeNum; } @Override public String toString() { return "Name: " + firstName + " " + lastName + " " + "ID: " + employeeNum + " " + "Department: " + department + " " + "Title: " + jobTitle; } public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public int getEmployeeNumber() { return employeeNum; } public String getDepartment() { return department; } public String getJobTitle() { return jobTitle; } public void setFirstName(String firstN) { firstName = firstN; } public void setLastName(String lastN) { lastName = lastN; } public void setEmployeeNumber(int empNum) { employeeNum = empNum; } public void setDepartment(String depart) { department = depart; } public void setJobTitle(String jbTitle) { jobTitle = jbTitle; } void printEmployee() { System.out.println("Name: " + firstName + " " + lastName); System.out.println("ID: " + employeeNum); System.out.println("Department: " + department); System.out.println("Title: " + jobTitle) ; } Employee(String firstN, String lastN, int empNum, String depart, String jbTitle, double payRt) { firstName = firstN; lastName = lastN; employeeNum = empNum; department = depart; jobTitle = jbTitle; } }
HERE IS A UML FOR EMPLOYEE.JAVA CODE:
TEST THE CODING WITH THIS:
import java.text.NumberFormat; public class A9EmployeeChecker { public static void main(String[] args) { NumberFormat currency = NumberFormat.getCurrencyInstance(); // add some employees in SalaryEmployee e1 = new SalaryEmployee("Steve", "Rodgers", 3781, "Sales", "Manager", 64325); CommissionEmployee e2 = new CommissionEmployee("Clint", "Barton", 6847, "Sales", "Customer Representative", .0265); HourlyEmployee e3 = new HourlyEmployee("Tony", "Stark", 5749, "Service", "Lead Service Manager", 32.85); // initial employee print System.out.println("*** Initial Employee Printing ***"); System.out.println(e1+" "); System.out.println(e2+" "); System.out.println(e3); // increase employees e2.increaseSales(325); e3.increaseHours(32); e2.increaseSales(-500); // check for negatives e3.increaseHours(-10); // check for negatives // view changes System.out.println(" *** Changed Employee sales/hours ***"); System.out.println(e1+" "); System.out.println(e2+" "); System.out.println(e3); // show weekly pay System.out.println(" *** Calculate Weekly Pay ***"); System.out.println(e1.getFirstName() + " " + e1.getLastName() + " - Weekly Pay: " + currency.format(e1.calculateWeeklyPay())); System.out.println(e2.getFirstName() + " " + e2.getLastName() + " - Weekly Pay: " + currency.format(e2.calculateWeeklyPay())); System.out.println(e3.getFirstName() + " " + e3.getLastName() + " - Weekly Pay: " + currency.format(e3.calculateWeeklyPay())); // reset week e1.resetWeek(); e2.resetWeek(); e3.resetWeek(); // check if reset week worked System.out.println(" *** Reset the Week ***"); e1.printEmployee(); System.out.println(); e2.printEmployee(); System.out.println(); e3.printEmployee(); // give holiday bonus System.out.println(" *** Give Holiday Bonus***"); System.out.println(e1.getFirstName() + " " + e1.getLastName() + " - Bonus: " + currency.format(e1.holidayBonus())); System.out.println(e2.getFirstName() + " " + e2.getLastName() + " - Bonus: " + currency.format(e2.holidayBonus())); System.out.println(e3.getFirstName() + " " + e3.getLastName() + " - Bonus: " + currency.format(e3.holidayBonus())); e1.annualRaise(); e2.annualRaise(); e3.annualRaise(); // check the annual raise System.out.println(" *** Annual Raises ***"); System.out.println(e1+" "); System.out.println(e2+" "); System.out.println(e3+" "); //Check the equals method System.out.println("*** Are employees equal ***"); if (!e1.equals(e2)) { System.out.println("Employee 1 is not Employee 2, Success!!"); } // new employee but with e1 employee number SalaryEmployee e4 = new SalaryEmployee("Joe", "Smith", 3781, "Sales", "Manager", 1000); if(e1.equals(e4)) { System.out.println("Employee 1 is the same as this new employee. SUCCESS!!"); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
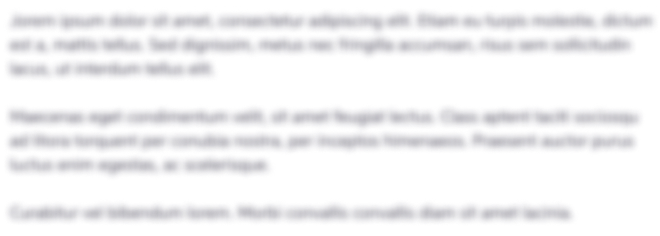
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started