Question
Using Java coding conventionsWrite a program that writes user supplied data to a text file and can perform a few statistical calculations on the data.
Using Java coding conventionsWrite a program that writes user supplied data to a text file and can perform a few statistical calculations on the data.
Constraints
The file directory will be C:/W12Assignment. Mac and Linux distro users can modify this location
Only one file will be created, used, and updated while the program runs
Requirements
Use exception handling and validation where appropriate
Use PrintWriter and Scanner for writing and reading data
Use a separate (external to the main class) subclass to write data
Use a separate (external to the main class) subclass to read data
Use a separate (external to the main class) subclass to validate entries
Use set and get methods in the subclasses
Find the average of all the numbers in the file
Find the smallest number in the file
Find the largest number in the file
Find the standard deviation (StdDev) of the number set (Follow the Basic Example as a model for your StdDev algorithm located here (Links to an external site.)Links to an external site..)
Implement a loop to run the program again if the user wishes (Note: this will only allow updates
or reading of the same file since only one file is used for duration of the program run)
Use the supplied MyNumbersLarge.txt file to make sure you are obtaining the correct information as such:
o All numbers printed (500 numbers) o The average of the numbers in file name is: 502.3212 o The smallest number in the file is: 1.2416 o The largest number in the file is: 999.3116 o The standard deviation for the numbers in this file is: 287.6556
Hints
Consider using an ArrayList of Doubles to store numbers as they are entered
Research "Collections" to assist with minimums and maximums
Considering writing the code to work with all correct entries before implementing validation and exception handling. Once the code works, then try to break it with bad entries. This way, you can separate the requirements (Code in chunks!)
FOR OUTPUT SEE IMAGE
Welcome to My Statistics Calculator Enter the name of your text file (e.g. MyNumbers) The file extension will be added after entry -: MyNumbers You must add the extension .txt in your code. The directory does not exist. Creating directory... Directory created at this location: C:\W12Assignment If either the directory or file do not exist, create them. If they do, notify the user that they exist The file does not exist. Creating file... File created at this location->: C: W12Assignment MyNumbers.txt Please select from the following menu of options 1. Enter Data 2. Read Data 3. Exit The file contains no data. Enter data?n Would you like to run the program again?->: y The user should be able to enter data here if they wish. Please select from the following menu of options: 1. Enter Data 2. Read Data 3. Exit -2 How many numbers would you like to send to the file?->: 6 Enter number 1->89.635 Enter number 2-> 100.3069 Enter number 3-> 101.0009 Enter number 4-95.365 Enter number 5 99.3644 Enter number 6-90.00049 Would you like to read the see the results? y The numbers in this file are: 89.6350 100.3069 101.0009 95.3650 99.3644 90.0005 The average of the numbers in file name is: 95.9455 The smallest number in the file is: 89.6350 The largest number in the file is: 101.0009 The standard deviation for the numbers in this file is: 4.6863 Would you like to run the program again?->:y
Step by Step Solution
There are 3 Steps involved in it
Step: 1
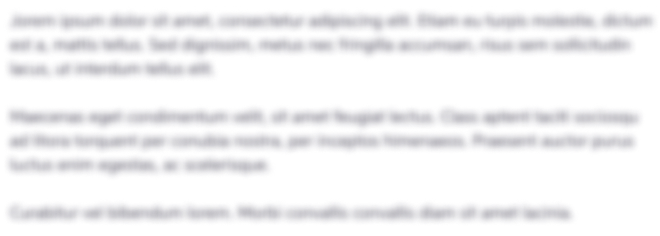
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started