Question
Using Java, create a random number game program with a menu that lets you play two different versions of the game, asks and answers a
Using Java, create a random number game program with a menu that lets you play two different versions of the game, asks and answers a question, and has an exit option. Refer to the UML diagram to create this program.
For MainClass, you should only have the 2 class level attributes listed and 2 instances (check the class diagram above for association). You shouldnt have to create any more variables or instances in this class. You should initialize your class level attributes and instances before the dowhile loop in this class. In the loop, you should present the user with a menu and ask them to select an option.
The options are:
1. Random Game Version 1 a. This should execute the checkWhile function from RandomGame
2. Random Game Version 2 a. This should execute the checkFor function from RandomGame
3. While vs DoWhile vs For a. This should print out a quick summary of the difference between a While Loop, a DoWhile Loop, and a For Loop.
4. Quit a. This should exit the program by setting the while condition, loopStatus, to false. Make sure to handle the user entering input that is not supported! You should print out an exit message after the loop has been completed. (DoWhile Loop)
For the RandomGame, you should only have the 4 class level attributes listed and 1 instance (again, check the class diagram above for association). You shouldnt have to create any more variables (except to increment the for loop) or instances in this class. You should set the value of your Scanner instance in the constructor. (We are setting the value of the Scanner in RandomGame to the scanner from MainClass so we dont have to worry about closing the System.in in RandomGame.)
The other three methods are explained as follows (Functions):
checkWhile o This method will call the newRandom function to generate a new random number. Then, the game will start by telling the user to guess a number between 1 and 10. A while loop will continuously have the user guess a number. If the number is correctly guessed, the game should let the user know that they have won and the user will return to the main menu by setting the while condition, guessed, to true. If the guess was incorrect, the user should be prompted to try guessing again. (While Loop)
checkFor o This method will call the newRandom function to generate a new random number. Then, the game will start by telling the user to guess a number between 1 and 10. A for loop will continuously have the user guess a number, but they are limited to guesses number of guesses. If the number is correctly guessed, the game should let the user know that they have won and the user will return to the main menu by setting the incrementing variable to guesses. If the guess was incorrect and still has available guesses, the user should be prompted to try guessing again. If the guess was incorrect and has run out of available guesses, the user should be given a game over message and sent back to the main menu. (For Loop)
newRandom o This method sets the randomNumber equal to a number from 1 to 10. (int)(Math.floor(Math.random()*10)+1)
RandomGame guessed: boolean= false +randomNumber: int tuserNumber: int +guesses: int +RandomGame (newInput:scanner) +chechile ( ) : void +checkFor void +newRandom ): void MainClass +menuoption: int +1oopStatus: boolean= true +main(): void Scanne Do notmake the Scanner dass. ImportitStep by Step Solution
There are 3 Steps involved in it
Step: 1
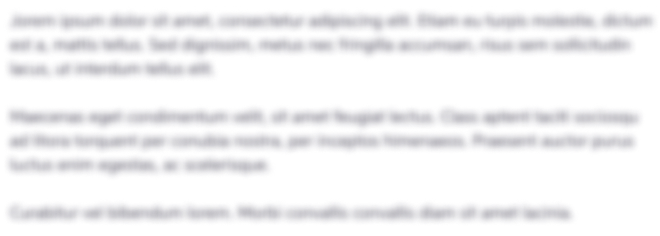
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started