Question
Using JAVA FX how would I combine the two programs below into one interface that allows the user to pick which specific program they would
Using JAVA FX how would I combine the two programs below into one interface that allows the user to pick which specific program they would like to play. They should be able to choose which one they want to play and then switch between them if necesary. All of this must be done in JAVA FX code
Black jack javafx - first game program
package application;
import java.util.Arrays;
import java.util.ArrayList;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.layout.HBox;
import javafx.scene.layout.GridPane;
import javafx.scene.control.Label;
import javafx.scene.control.Labeled;
import javafx.scene.control.Button;
import javafx.scene.control.TextField;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.stage.Stage;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
public class Main extends Application {
public String btHitY;
public int NumberPlayerCards;
public int NumberDealerCards;
public int NUMBER_OF_CARDS;
public int PlayerCards[];
public int DealerCards[];
public Image imagesP[];
public Image imagesD[];
public int deck[];
public String URLBase;
public GridPane pane;
public Main() {
this.btHitY = " ";
this.btHitY = new String();
}
@Override // Override the start method in the Application class
public void start(Stage primaryStage) {
//Create array deck, suit string, and rank string
deck = new int[52];
String[] suits = {"Spades", "Hearts", "Diamonds", "Clubs"};
String[] ranks = {"Ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King"};
int NUMBER_OF_CARDS = 4;
//Initialize the cards
for (int i = 0; i < deck.length; i++)
deck[i] = i;
//Shuffle the cards
for (int i = 0; i < deck.length; i++) {
//Generate an index randomly
int index = (int)(Math.random() * deck.length);
int temp = deck[i];
deck[i] = deck[index];
deck[index] = temp;
}
int NumberPlayerCards = 2;
int NumberDealerCards = 2;
int[] PlayerCards = new int[50];
int[] DealerCards = new int[50];
//Display the first cards
for (int i = 0; i < 8; i++) {
String suit = suits[deck[i] / 13];
String rank = ranks[deck[i] % 13];
System.out.println("Card number " + deck[i] + ": " + rank + " of " + suit);
}
for (int i = 0; i < NumberPlayerCards; i++)
PlayerCards[i] = deck[i * 2];
for (int i = 0; i < NumberDealerCards; i++)
DealerCards[i] = deck[i * 2 + 1];
// Create a pane to hold the image views
GridPane pane = new GridPane();
pane.setAlignment(Pos.CENTER);
pane.setPadding(new Insets(5, 5, 5, 5));
pane.setHgap(5);
pane.setVgap(5);
Image[] imagesP = new Image[50];
Image[] imagesD = new Image[50];
for (int i = 0; i < NumberPlayerCards; i++) {
int cardForPrint = PlayerCards[i] + 1;
System.out.println(URLBase + cardForPrint + ".png");
imagesP[i] = new Image(URLBase + cardForPrint + ".png");
}
for (int i = 0; i < NumberDealerCards; i++) {
int cardForPrint = DealerCards[i] + 1;
System.out.println(URLBase + cardForPrint + ".png");
imagesD[i] = new Image(URLBase + cardForPrint + ".png");
}
//rotate flag image to cover dealer card
Image flag = new Image("http://www.cs.armstrong.edu/liang/common/image/us.gif");
ImageView imageFlag = new ImageView(flag);
imageFlag.setRotate(90);
imageFlag.setFitHeight(75);
imageFlag.setFitWidth(95);
pane.add(new Label("Player Cards"), 0, 0);
pane.add(new ImageView(imagesP[0]), 1, 0);
pane.add((imageFlag), 1, 1);
pane.add(new Label("Dealer Cards"), 0, 1);
pane.add(new ImageView(imagesP[1]), 2, 0);
pane.add(new ImageView(imagesD[1]), 2, 1);
Button btHit = new Button("Hit");
Button btStay = new Button("Stay");
pane.add(btHit, 1, 2);
pane.add(btStay, 2, 2);
// Create a scene and place it in the stage
Scene scene = new Scene(pane, 1200, 700);
primaryStage.setTitle("Black Jack"); // Set the stage title
primaryStage.setScene(scene); // Place the scene in the stage
primaryStage.show(); // Display the stage
HitHandlerClass handlerHit = new HitHandlerClass();
btHitY = " ";
btHit.setOnAction(handlerHit);
/* if (btHitY.equals("Hit")); {
NumberPlayerCards = NumberPlayerCards + 1;
NUMBER_OF_CARDS = NUMBER_OF_CARDS + 1;
PlayerCards[NumberPlayerCards - 1] = deck[NUMBER_OF_CARDS - 1];
for (int j = 0; j < NumberPlayerCards; j++){
System.out.println(PlayerCards[j]);
}
System.out.println(NumberPlayerCards);
int CardForPrint2 = PlayerCards[NumberPlayerCards - 1] + 1;
imagesP[NumberPlayerCards - 1] = new Image(URLBase + CardForPrint2 + ".png");
pane.add(new ImageView(imagesP[NumberPlayerCards - 1]), NumberPlayerCards, 0);
btHitY = " ";
primaryStage.show();
} */
}
/**
* The main method is only needed for the IDE with limited
* JavaFX support. Not needed for running from the command line.
* @param args
*/
public static void main(String[] args) {
launch(args);
}
class HitHandlerClass implements EventHandler
@Override
public void handle(ActionEvent e) {
NumberPlayerCards = NumberPlayerCards + 1;
NUMBER_OF_CARDS = NUMBER_OF_CARDS + 1;
PlayerCards[NumberPlayerCards - 1] = deck[NUMBER_OF_CARDS - 1];
for (int j = 0; j < NumberPlayerCards; j++){
System.out.println(PlayerCards[j]);
}
System.out.println(NumberPlayerCards);
int CardForPrint2 = PlayerCards[NumberPlayerCards - 1] + 1;
imagesP[NumberPlayerCards - 1] = new Image(URLBase + CardForPrint2 + ".png");
pane.add(new ImageView(imagesP[NumberPlayerCards - 1]), NumberPlayerCards, 0);
btHitY = " ";
}
}
}
Next game
import java.util.Calendar; import java.util.GregorianCalendar; import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.layout.BorderPane; import javafx.scene.layout.GridPane; import javafx.scene.layout.HBox; import javafx.scene.paint.Color; import javafx.scene.text.TextAlignment; import javafx.stage.Stage; public class MyCalendar extends Application { @Override // Override the start method in the Application class public void start(Stage primaryStage) { BorderPane pane = new BorderPane(); CalendarPane calendarPane = new CalendarPane(); pane.setCenter(calendarPane); Button btPrior = new Button("Prior"); Button btNext = new Button("Next"); HBox hBox = new HBox(5); hBox.getChildren().addAll(btPrior, btNext); pane.setBottom(hBox); hBox.setAlignment(Pos.CENTER); // Create a scene and place it in the stage Scene scene = new Scene(pane, 600, 300); primaryStage.setTitle("My Calendar"); // Set the stage title primaryStage.setScene(scene); // Place the scene in the stage primaryStage.show(); // Display the stage btPrior.setOnAction(e -> { int currentMonth = calendarPane.getMonth(); if (currentMonth == 0) { // The previous month is 11 for Dec calendarPane.setYear(calendarPane.getYear() - 1); calendarPane.setMonth(11); } else { calendarPane.setMonth((currentMonth - 1) % 12); } }); btNext.setOnAction(e -> { int currentMonth = calendarPane.getMonth(); if (currentMonth == 11) // The next month is 0 for Jan calendarPane.setYear(calendarPane.getYear() + 1); calendarPane.setMonth((currentMonth + 1) % 12); }); } /** * The main method is only needed for the IDE with limited * JavaFX support. Not needed for running from the command line. */ public static void main(String[] args) { launch(args); } } class CalendarPane extends BorderPane { private String[] monthName = {"January", "Feburary", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"}; // The header label private Label lblHeader = new Label(); // Maximum number of labels to display day names and days private Label[] lblDay = new Label[49]; private Calendar calendar; private int month; // The specified month private int year; // The specified year public CalendarPane() { // Create labels for displaying days for (int i = 0; i < 49; i++) { lblDay[i] = new Label(); lblDay[i].setTextAlignment(TextAlignment.RIGHT); } lblDay[0].setText("Sunday"); lblDay[1].setText("Monday"); lblDay[2].setText("Tuesday"); lblDay[3].setText("Wednesday"); lblDay[4].setText("Thursday"); lblDay[5].setText("Friday"); lblDay[6].setText("Saturday"); GridPane dayPane = new GridPane(); dayPane.setAlignment(Pos.CENTER); dayPane.setHgap(10); dayPane.setVgap(10); for (int i = 0; i < 49; i++) { dayPane.add(lblDay[i], i % 7, i / 7); } // Place header and calendar body in the pane this.setTop(lblHeader); BorderPane.setAlignment(lblHeader, Pos.CENTER); this.setCenter(dayPane); // Set current month and year calendar = new GregorianCalendar(); month = calendar.get(Calendar.MONTH); year = calendar.get(Calendar.YEAR); updateCalendar(); // Show calendar showHeader(); showDays(); } public void showHeader() { lblHeader.setText(monthName[month] + ", " + year); } /** * Display days */ public void showDays() { // Get the day of the first day in a month int startingDayOfMonth = calendar.get(Calendar.DAY_OF_WEEK); // before month dates are printed in red colour Calendar cloneCalendar = (Calendar) calendar.clone(); cloneCalendar.add(Calendar.DATE, -1); // Becomes preceding month int daysInPrecedingMonth = cloneCalendar.getActualMaximum( Calendar.DAY_OF_MONTH); for (int i = 0; i < startingDayOfMonth - 1; i++) { lblDay[i + 7].setTextFill(Color.RED); lblDay[i + 7].setText(daysInPrecedingMonth - startingDayOfMonth + 2 + i + ""); } // current month date is in BLUE colour int daysInCurrentMonth = calendar.getActualMaximum( Calendar.DAY_OF_MONTH); for (int i = 1; i <= daysInCurrentMonth; i++) { lblDay[i - 2 + startingDayOfMonth + 7].setTextFill(Color.BLUE); lblDay[i - 2 + startingDayOfMonth + 7].setText(i + ""); } // after month dates are printed in green colour int j = 1; for (int i = daysInCurrentMonth - 1 + startingDayOfMonth + 7; i < 49; i++) { lblDay[i].setTextFill(Color.GREEN); lblDay[i].setText(j++ + ""); } } /** * Set the calendar to the first day of the specified month and year */ public void updateCalendar() { calendar.set(Calendar.YEAR, year); calendar.set(Calendar.MONTH, month); calendar.set(Calendar.DATE, 1); } /** * Return month */ public int getMonth() { return month; } /** * Set a new month */ public void setMonth(int newMonth) { month = newMonth; updateCalendar(); showHeader(); showDays(); } /** * Return year */ public int getYear() { return year; } /** * Set a new year */ public void setYear(int newYear) { year = newYear; updateCalendar(); showHeader(); showDays(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
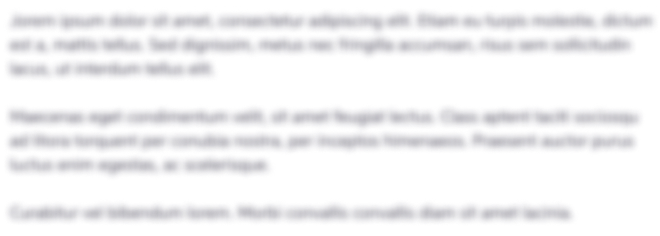
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started