Question
Using Java In this lab, you will be working with Business Objects and GUIs. A.) Recall the Student class that you built in Lab #8(Student.java).
Using Java
In this lab, you will be working with Business Objects and GUIs.
A.) Recall the Student class that you built in Lab #8(Student.java). You will be using this class to access the Database. Also recall the StudentGUI class that you built in Lab #1 or #2(StudentGUI.java).
B.) You may want to create a Lab9 folder, and copy the above 2 files and all related files(the StudentGUI may have related Panel files, like BottomPanel.java) into the Lab10 folder.
C.) Your task for this lab is to make a students data(name, email, gpa) show up in the StudentGUI.
D.) The user will run the StudentGUI class, and the GUI will show up. The User will then enter a student ID number in the ID textfield and click the Find button. Your event code will read the ID number the user entered, use that ID number and the Student Business Object to retrieve that one students data from the DB(selectDB()). Lastly, pull out the individual data elements(i.e firstName) from the Student Business Object and put them into the appropriate textfields in the StudentGUI.
E.) Also make the Insert, Delete and Update buttons work. Have fun.
//Class Name:StudentData
import java.awt.event.WindowAdapter;
import javax.swing.JFrame;
public class StudentData extends javax.swing.JFrame { public StudentData() { initComponents(); this.setDefaultCloseOperation(javax.swing.JFrame.DO_NOTHING_ON_CLOSE); this.addWindowListener(new WindowAdapter(){ public void windowClosing(java.awt.event.WindowEvent e){ int x=javax.swing.JOptionPane.showConfirmDialog(null,"Do you want to exit","close",javax.swing.JOptionPane.YES_NO_OPTION,javax.swing.JOptionPane.INFORMATION_MESSAGE); if(x==javax.swing.JOptionPane.YES_OPTION) { e.getWindow().dispose(); System.exit(0); } } }); } @SuppressWarnings("unchecked") //
jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jLabel4 = new javax.swing.JLabel(); jLabel5 = new javax.swing.JLabel(); jLabel6 = new javax.swing.JLabel(); jTextField1 = new javax.swing.JTextField(); jTextField2 = new javax.swing.JTextField(); jTextField3 = new javax.swing.JTextField(); jTextField4 = new javax.swing.JTextField(); jTextField5 = new javax.swing.JTextField(); jButton1 = new javax.swing.JButton(); jButton2 = new javax.swing.JButton(); jButton3 = new javax.swing.JButton(); jButton4 = new javax.swing.JButton(); jButton5 = new javax.swing.JButton();
setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N jLabel1.setForeground(new java.awt.Color(0, 153, 153)); jLabel1.setText("Student Information");
jLabel2.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N jLabel2.setForeground(new java.awt.Color(255, 0, 51)); jLabel2.setText("LastName");
jLabel3.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N jLabel3.setForeground(new java.awt.Color(255, 0, 51)); jLabel3.setText("ID");
jLabel4.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N jLabel4.setForeground(new java.awt.Color(255, 0, 51)); jLabel4.setText("FirstName");
jLabel5.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N jLabel5.setForeground(new java.awt.Color(255, 0, 51)); jLabel5.setText("Email");
jLabel6.setFont(new java.awt.Font("Tahoma", 0, 18)); // NOI18N jLabel6.setForeground(new java.awt.Color(255, 0, 0)); jLabel6.setText("GPA");
jTextField1.setToolTipText("enter Id"); jTextField1.addFocusListener(new java.awt.event.FocusAdapter() { public void focusGained(java.awt.event.FocusEvent evt) { none(evt); } }); jTextField1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jTextField1ActionPerformed(evt); } });
jTextField2.setToolTipText("Enter LastName"); jTextField2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jTextField2ActionPerformed(evt); } });
jTextField3.setToolTipText("Enter FirstName"); jTextField3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jTextField3ActionPerformed(evt); } });
jTextField4.setToolTipText("Enter Emial "); jTextField4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jTextField4ActionPerformed(evt); } });
jTextField5.setToolTipText("Enter GPA"); jTextField5.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jTextField5ActionPerformed(evt); } });
jButton1.setText("Find"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } });
jButton2.setText("Insert"); jButton2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton2ActionPerformed(evt); } });
jButton3.setText("Delete"); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } });
jButton4.setText("Update"); jButton4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton4ActionPerformed(evt); } });
jButton5.setText("Exit"); jButton5.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton5ActionPerformed(evt); } });
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(128, 128, 128) .addComponent(jLabel1)) .addGroup(layout.createSequentialGroup() .addGap(79, 79, 79) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel3, javax.swing.GroupLayout.PREFERRED_SIZE, 63, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel4, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel2, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel5, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false) .addComponent(jTextField5, javax.swing.GroupLayout.DEFAULT_SIZE, 130, Short.MAX_VALUE) .addComponent(jTextField4) .addComponent(jTextField2) .addComponent(jTextField3) .addComponent(jTextField1)))) .addGap(54, 54, 54)) .addGroup(layout.createSequentialGroup() .addGap(19, 19, 19) .addComponent(jButton1) .addGap(18, 18, 18) .addComponent(jButton2) .addGap(18, 18, 18) .addComponent(jButton3) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addComponent(jButton4) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addComponent(jButton5) .addContainerGap(34, Short.MAX_VALUE)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, 22, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(36, 36, 36) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel3) .addComponent(jTextField1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel4) .addComponent(jTextField3, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel2) .addComponent(jTextField2, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel5) .addComponent(jTextField4, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel6) .addComponent(jTextField5, javax.swing.GroupLayout.PREFERRED_SIZE, 22, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(18, 18, 18) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton1) .addComponent(jButton2) .addComponent(jButton3) .addComponent(jButton4) .addComponent(jButton5)) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) );
pack(); }//
private void jTextField1ActionPerformed(java.awt.event.ActionEvent evt) { //jTextField1.setToolTipText("Enter your username"); }
private void jTextField2ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jTextField3ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jTextField4ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jTextField5ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jButton2ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jButton3ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jButton4ActionPerformed(java.awt.event.ActionEvent evt) { // TODO add your handling code here: }
private void jButton5ActionPerformed(java.awt.event.ActionEvent evt) { System.exit(0); }
private void none(java.awt.event.FocusEvent evt) { // TODO add your handling code here: }
/** * @param args the command line arguments */ public static void main(String args[]) { /* Set the Nimbus look and feel */ //
/* Create and display the form */ java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new StudentData().setVisible(true); } }); }
// Variables declaration - do not modify private javax.swing.JButton jButton1; private javax.swing.JButton jButton2; private javax.swing.JButton jButton3; private javax.swing.JButton jButton4; private javax.swing.JButton jButton5; private javax.swing.JLabel jLabel1; private javax.swing.JLabel jLabel2; private javax.swing.JLabel jLabel3; private javax.swing.JLabel jLabel4; private javax.swing.JLabel jLabel5; private javax.swing.JLabel jLabel6; public javax.swing.JTextField jTextField1; public javax.swing.JTextField jTextField2; public javax.swing.JTextField jTextField3; public javax.swing.JTextField jTextField4; public javax.swing.JTextField jTextField5; // End of variables declaration }
public class Student {
private int id;
private String firstName;
private String street;
private String city;
private String state;
private String zip;
private String email;
double gpa;
private String lastName;
public Student(int id, String firstName, String lastName, String street, String city, String state, String zip,
String email, double gpa) {
super();
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.street = street;
this.city = city;
this.state = state;
this.zip = zip;
this.email = email;
this.gpa = gpa;
}
public Student() {
super();
}
public void display() {
System.out.println("ID "+getId()+" First Name "+getFirstName()+" Last Name "+getLastName()+ " Email "+getEmail()+" City "+getCity()+" Street "+getStreet()+" State "+getState()+" Zip "+getZip()+" GPA "+getGpa());
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getStreet() {
return street;
}
public void setStreet(String street) {
this.street = street;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getZip() {
return zip;
}
public void setZip(String zip) {
this.zip = zip;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public double getGpa() {
return gpa;
}
public void setGpa(double gpa) {
this.gpa = gpa;
}
public static void main(String args[]) {
Student s1 = new Student(1, "rahul", "chopra", "1 india", "nxyz", "abcz", "1313", "abcz@abcz.com", 3.2);
Student s2 = new Student(2, "firstName", "lastName", "street", "city", "state", "zip", "email", 3.2);
s1.display();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
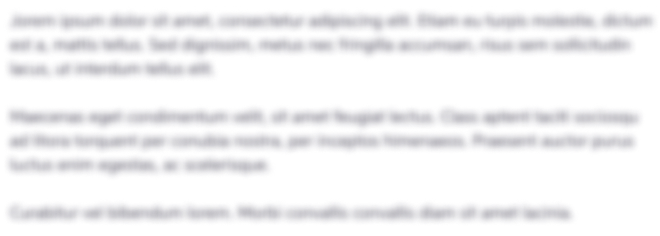
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started