Question
Using Java modify the code below to Write a static method totalDurations that is passed the parallel arrays from the main program. It creates a
Using Java modify the code below to
Write a static method totalDurations that is passed the parallel arrays from the main program. It creates a new pair of parallel arrays (phone numbers and durations, again) where each different incoming phone number is stored exactly once, and the duration is the total duration of all the calls from that phone number. It then prints these arrays. Nothing is returned.
For example, if the arrays contain 555-1234 (duration 10), 555-4321 (duration 20), and 555-1234 (duration 30), then the output would show 555-1234 (duration 40) and 555-4321 (duration 20).
To help you solve the problem, you can call the find method. Use it to check if a phone number has already been placed in the new arrays, and if so, to determine where that number is. Also note that the size of the new arrays will never be greater than the current list.
public class Activity0D { public static void main(String[] args) { String[] phoneNumbers = new String[100]; int[] callDurations = new int[phoneNumbers.length]; int size = 0;
size = addCall(phoneNumbers, callDurations, size, "555-555-5555", 137); size = addCall(phoneNumbers, callDurations, size, "555-555-0000", 12); size = addCall(phoneNumbers, callDurations, size, "555-555-1234", 26); size = addCall(phoneNumbers, callDurations, size, "555-555-9876", 382);
System.out.println("Phone numbers (initially):"); printList(phoneNumbers, callDurations, size);
size = removeCall(phoneNumbers, callDurations, size, 1); // middle size = removeCall(phoneNumbers, callDurations, size, size - 1); // last size = removeCall(phoneNumbers, callDurations, size, 0); // first
System.out.println(" Phone numbers (after):"); printList(phoneNumbers, callDurations, size);
size = removeCall(phoneNumbers, callDurations, size, 0);
System.out.println(" Phone numbers (none left):"); printList(phoneNumbers, callDurations, size);
System.out.println(" End of processing."); }
public static int addCall(String[] phoneNumbers, int[] callDurations, int size, String newNumber, int newDuration) { if (size >= phoneNumbers.length) { System.out.println("Error adding " + newNumber + ": array capacity exceeded."); } else { phoneNumbers[size] = newNumber; callDurations[size] = newDuration; size++; }
return size; }
public static void printList(String[] phoneNumbers, int[] callDurations, int size) { for (int i = 0; i < size; i++) { System.out.println(phoneNumbers[i] + " duration: " + callDurations[i] + "s"); } }
public static int find(String[] list, int size, int start, String target) { int pos = start;
while (pos < size && !target.equals(list[pos])) { pos++; }
if (pos == size) pos = -1;
return pos; }
public static void findAllCalls(String[] phoneNumbers, int[] callDurations, int size, String targetNumber) { int matchPos;
System.out.println("Calls from " + targetNumber + ":"); matchPos = find(phoneNumbers, size, 0, targetNumber); while (matchPos >= 0) { System.out.println(phoneNumbers[matchPos] + " duration: " + callDurations[matchPos] + "s");
// Find the next match, starting after the last one matchPos = find(phoneNumbers, size, matchPos + 1, targetNumber); } }
public static int removeCall(String[] phoneNumbers, int[] callDurations, int size, int posToRemove) { for (int i = posToRemove + 1; i < size; i++) { phoneNumbers[i - 1] = phoneNumbers[i]; callDurations[i - 1] = callDurations[i]; } size--;
return size; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
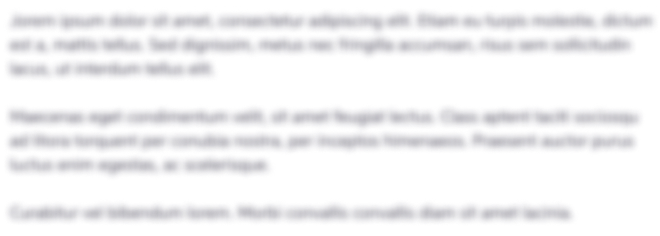
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started