Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using Java, modify the existing code. I t i s three classes. 1 . Replace the ArrayList in your assignment with a LinkedList from the
Using Java, modify the existing code. three classes.
Replace the ArrayList in your assignment with a LinkedList from the Java collections. Do NOT reimplement the linked list like you did in CodeStepByStep, just use the one provided. The algorithm that you implement for your program, will be exactly the same, only the list implementation will change.
Compare the timing for using an ArrayList with the same timing for using a LinkedList. Try to explain what you see and make any recommendations. Also, consider your proposed algorithm from Unit that rearranges Contestants in the same list. Based on your timing estimates, explain whether you think it would take more or less time using a LinkedList rather than an ArrayList.
Contestant Class:
import java.util.ArrayList;
import java.util.List;
public class Contestant extends Person
private int currentPosition;
private List positionHistory;
A list of integers that records the history of all positions the contestant has been in
public ContestantString name
supername;
this.positionHistory new ArrayList;
public int getCurrentPosition
return currentPosition;
Returns the current position of the contestant.
public void setCurrentPositionint position
this.currentPosition position;
this.positionHistory.addposition;
Sets the current position to the given position and adds this position to the positionHistory list.
public double getAveragePosition
Calculates and returns the average of all positions stored in
int sum ;
for int pos : positionHistory
sum pos;
return double sum positionHistory.size;
It sums up all the positions and divides the sum by the number of positions in the list.
ContestDriver Class:
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class ContestSimulation
private static final Random random new Random;
public static void mainString args
int numberOfContestants ;
int numberOfRounds ;
Initializes a list of contestants.
List contestants new ArrayList;
for int i ; i numberOfContestants; i
contestants.addnew ContestantContestant charA i;
for int i ; i contestants.size; i
contestants.getisetCurrentPositioni;
Runs a specified number of rounds.
for int round ; round numberOfRounds; round
simulateRoundcontestants;
printAveragePositionscontestants;
Each round simulates contestants either answering correctly or incorrectly and updates their positions accordingly.
public static void simulateRoundList contestants
for int i ; i contestants.size; i
Contestant contestant contestants.geti;
boolean answeredCorrectly random.nextBoolean;
if answeredCorrectly
contestants.removei;
contestants.add contestant;
else
contestants.removei;
contestants.addcontestant;
for int i ; i contestants.size; i
contestants.getisetCurrentPositioni;
After all rounds, it prints the average position of each contestant.
printContestantscontestants;
public static void printContestantsList contestants
System.out.printlnCurrent positions:";
for Contestant contestant : contestants
System.out.printlncontestantgetName at position contestant.getCurrentPosition;
System.out.println;
public static void printAveragePositionsList contestants
System.out.printlnAverage positions:";
for Contestant contestant : contestants
System.out.printlncontestantgetName average position contestant.getAveragePosition;
Person Class:
public class Person
private String name;
public PersonString name
this.name name;
Creates a Person object with a specific name.
public String getName
return name;
Allows external code to access the value of the private name field. It returns the current value of name.
public void setNameString name
this.name name;
Allows external code to modify the value of the private name field. It takes a String argument and assigns it to the name field.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
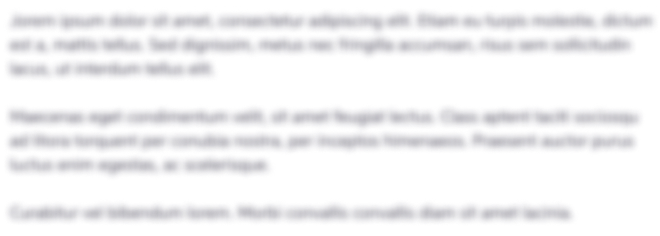
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started