Question
Using Java Write an abstract class called Number. It should have a single abstract method called absoluteValue that returns a double. You will extend this
Using Java
Write an abstract class called Number. It should have a single abstract method called absoluteValue that returns a double. You will extend this class with two additional classes: Rational and Complex.
A Rational number is one that can be expressed as a ratio of integers so a Rational number is defined by the instance variables numerator and denominator. A Complex number is one that contains a real part and an imaginary part, both doubles. In addition to their appropriate instance variables, Rational and Complex should each have a single constructor that fully defines the object. Rational should implement these methods:
double absoluteValue()
int compareTo(Object otherObject) //Should use the absolute value of the number for the comparison
double getValue() //This should evaluate the fraction and return the rational number
String toString()
Complex should implement these methods:
double absoluteValue()
int compareTo(Object otherObject) //Should use the absolute value of the number for the comparison
String toString()
Notes:
Both Rational and Complex should implement Comparable
The string representation of a Rational object should look like this: "numerator/denominator = value" (without the quotes)
The string representation of a Complex object should look like "realPart - imaginaryPart*i" if imaginaryPart is < 0 and "realPart + imaginaryPart*i" otherwise and both without the quotes.
If you don't remember how to calculate the absolute value of a complex number, look it up...
You should write a tester class that creates an array of 5 Rational objects and 5 Complex objects. You should use the Arrays.sort method to sort each of those arrays and display the arrays pre and post-sorting. You should print at least two of the Complex objects and one of the Rational objects to demonstrate that your toString methods are producing output according the the specifications above.
You should submit .java files for Number, Rational, Complex, and your tester class.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
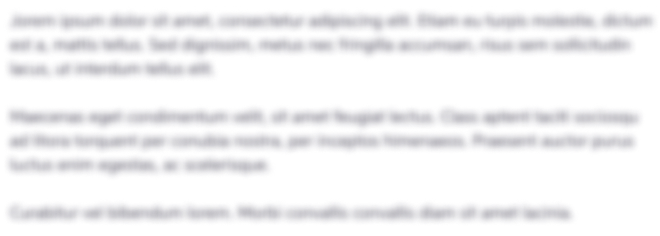
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started