Question
Using matlab. Write the function shiftLetter( ). This function is passed two parameters, a character and an amount by which to shift. It returns a
Using matlab. Write the function shiftLetter( ). This function is passed two parameters, a character and an amount by which to shift. It returns a new character.
Sample usage:
shiftletter('a',3) -> 'd'
shiftletter('y',3) -> 'b'
note that the integer used to represent 'a' is 97, 'A' is 65, 'z' is 122, 'Z' is 90
tip: in the function, convert the character to an integer, add the shift, then convert back to a character
How can you get it to "wrap around"? For example, what if you want to shift 'y' by 3?
if shifting moves a number "out of range", you need to move it back in.one way to do this is to check if a number is out of range, then find out by how much, and "add" that back to 'a':
'y' + 3 -> 124
124 - 'z' -> 2
'a' + 2 -1 = 98 (this accounts for the fact that 'a' is already 1 character into the wrap around
char(98) -> b
what if it is too low?
do the same, but subtracting from z
a "cleaner" way is to use the remainder function
convert the initial letter to an "index" between 0 and 25 by subtracting 'a' or 'A' (you can come up with a way that always uses the same one)
add the shift to that index
use mod( ) to divide by 26, thereby wrapping around
add the new index back to 'a'
convert it back to a character
Step 2
A shift (or rotation, or Caesar) cipher is a kind of encryption which replaces all the characters in a string with a letter several places away. For instance rot('hello',1) shifts every letter in the string 'hello' to the adjacent letter: 'ifmmp'. rot('hello',5) would produce 'mjqqt'. Notice that the alphabet circles around, so that 'z' becomes 'a' if shifted by 1. Notice also that decrypting a shifted string simply involves negating the shift value: rot('ifmmp',-1) -> 'hello'.
Write the function rot(str,shift). This function has two parameters, a string of characters and a shift amount, and returns the new string generated by using the function shiftLetter on every letter in the string. You cannot assume the input string is all lowercase, but your output string should be all lowercase. Do not change characters that are not upper or lowercase letters, just move them into the result string. So rot('Hello!', 1) -> 'ifmmp!' You may use the built-in MATLAB functions ischar(), isletter(), upper(), and lower(). Implement rot( ) by using your above shiftLetter( ) function on every character in an input string.
Step 3
If I tell you the shift key, it's easy to convert an entire message. But if you don't already have the shift key, then you'll need to make a guess at what it might be. You can make this an educated guess by counting letters and computing their frequency!
Write a function LetterCounts( ). This function has one parameter, a string. It will output a 26-element vector corresponding to the frequency of the letters. You can do this by first initializing your output vector to 26 0s. Then, iterate through every character in the string, finding which letter of the alphabet that character is, and incrementing the corresponding index by 1. When you are all done, compute the actual frequency based on the length of the input string. You want the frequency values in percentages. In other words, if I computed frequencies for the string 'aaabb' the initial vector of letter counts would be [3, 2, 0, 0, ..., 0] and the final output vector would be [60, 40, 0, 0, 0, ...., 0] indicating that 'a' appeared 60% of the time, 'b' appeared 40% of the time.
This function will give you the information you need in Step 4 to compare between frequencies of letters in a coded message and standard letter frequencies in order to determine what the shift key would have to be to get high frequency letters in the code to become the usual high frequency letters.
Step 4
How would you decode the following message?
M egnefufgfuaz okbtqd fmwqe fia xuefe, azq ar wqke mzp azq ar egne, mxazs iuft m efduzs fa qzoapq, mzp dqbxmoqe qhqdk otmdmofqd rday ftq efduzs ituot ue uz ftq wqke xuef iuft ftq oaddqebazpuzs hmxgq uz ftq egne xuef. Rad uzefmzoq: wqke = "mqua" egne = "4310" , ftqz egn(wqke,egne,"tqxxa") nqoayqe "t3xx0". Idufq egn() mzp geq uf fa pqoapq ftq yqeemsq raxxaiuzs: qau tlfk pkoapkp htk rgzlj ykiilsk. kxokjjkzh eadw! htgi eli bdlohgok rad htk kxly, g ly iudk qau egjj pa ekjj!
Use letterCounts(string) to determine the letter frequencies in the coded message, and compare them to standard letter frequencies in English. Then you can make an educated guess about what the shift index might be. The Wikipedia table on letter frequencies in English might be helpful. After you have a guess, try decoding the entire message using the functions you have already written.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
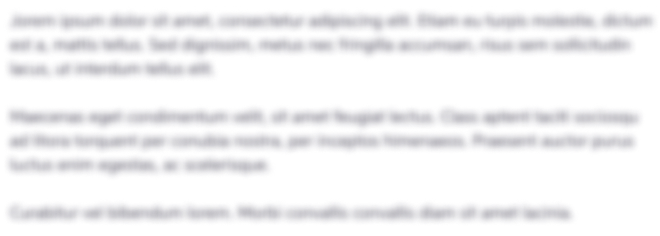
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started