Question
using node.js Use NPM to download a free module called colors. Modify app.js file so that the customers feedback text will be shown as green
using node.js
Use NPM to download a free module called colors. Modify app.js file so that the customers feedback text will be shown as green in the black console.
I already installed colors.js but it still not change the text color.
app.js:
/**
app.js
This is a simple web site that hosts
a fake Wake Tech Credit Union web site.
It is used to demonstrate how easy it is to
create and deploy a web sever using Node.js.
*/
var express = require('express');
var colors = require('./node_modules/colors');
var fs = require('fs');
/**
* Define the sample application.
*/
var SampleApp = function() {
// Scope.
var self = this;
/* ================================================================ */
/* Helper functions. */
/* ================================================================ */
/**
* Set up server IP address and port # using env variables/defaults.
*/
self.setupVariables = function() {
// Set the environment variables we need.
//self.ipaddress = process.env.IP;
//self.port = process.env.PORT || 5000;
//if (typeof self.ipaddress === "undefined") {
// self.ipaddress = "127.0.0.1";
//};
};
/**
* Populate the cache.
*/
self.populateCache = function() {
if (typeof self.zcache === "undefined") {
self.zcache = { 'index.html': '' };
}
// Local cache for static content.
self.zcache['index.html'] = fs.readFileSync('./index.html');
};
/**
* Retrieve entry (content) from cache.
* @param {string} key Key identifying content to retrieve from cache.
*/
self.cache_get = function(key) { return self.zcache[key]; };
/**
* terminator === the termination handler
* Terminate server on receipt of the specified signal.
* @param {string} sig Signal to terminate on.
*/
self.terminator = function(sig){
if (typeof sig === "string") {
console.log('%s: Received %s - terminating sample app ...',
Date(Date.now()), sig);
process.exit(1);
}
console.log('%s: Node server stopped.', Date(Date.now()) );
};
/**
* Setup termination handlers (for exit and a list of signals).
*/
self.setupTerminationHandlers = function(){
// Process on exit and signals.
process.on('exit', function() { self.terminator(); });
// Removed 'SIGPIPE' from the list - bugz 852598.
['SIGHUP', 'SIGINT', 'SIGQUIT', 'SIGILL', 'SIGTRAP', 'SIGABRT',
'SIGBUS', 'SIGFPE', 'SIGUSR1', 'SIGSEGV', 'SIGUSR2', 'SIGTERM'
].forEach(function(element, index, array) {
process.on(element, function() { self.terminator(element); });
});
};
/* ================================================================ */
/* App server functions (main app logic here). */
/* ================================================================ */
/**
* Create the routing table entries + handlers for the application.
*/
self.createRoutes = function() {
self.routes = { };
self.routes['/feedback'] = function(req, res) {
console.log("-- Received a customer feedback: [" + req.body.feedback.colors.red + "]");
res.send("
WTCU Feedback
Thanks for your feedback!
");
};
self.routes['/'] = function(req, res) {
res.setHeader('Content-Type', 'text/html');
res.send(self.cache_get('index.html') );
};
};
/**
* Initialize the server (express) and create the routes and register
* the handlers.
*/
self.initializeServer = function() {
self.createRoutes();
//self.app = express.createServer();
self.app = express();
self.app.set('port', process.env.PORT || 3333);
self.app.set('ip', process.env.IP || "127.0.0.1");
self.app.use(express.static(__dirname));
//self.app.use(express.bodyParser());
self.app.use(express.json());
self.app.use(express.urlencoded());
// Add handlers for the app (from the routes).
for (var r in self.routes) {
self.app.get(r, self.routes[r]); // maps the HTTP GET request
self.app.post(r, self.routes[r]); // maps the HTTP POST request
}
};
/**
* Initializes the sample application.
*/
self.initialize = function() {
self.setupVariables();
self.populateCache();
self.setupTerminationHandlers();
// Create the express server and routes.
self.initializeServer();
};
/**
* Start the server (starts up the sample application).
*/
self.start = function() {
// Start the app on the specific interface (and port).
self.app.listen(self.app.get('port'), function() {
console.log('%s: Node server started on %s:%d ...',
Date(Date.now() ), self.app.get('ip'), self.app.get('port'));
});
};
}; /* Sample Application. */
/**
* main(): Main code.
*/
var zapp = new SampleApp();
zapp.initialize();
zapp.start();
nods_modules/colors.js:
var colors = {}; module['exports'] = colors;
colors.themes = {};
var ansiStyles = colors.styles = require('./styles'); var defineProps = Object.defineProperties;
colors.supportsColor = require('./system/supports-colors');
if (typeof colors.enabled === "undefined") { colors.enabled = colors.supportsColor; }
colors.stripColors = colors.strip = function(str){ return ("" + str).replace(/\x1B\[\d+m/g, ''); };
var stylize = colors.stylize = function stylize (str, style) { if (!colors.enabled) { return str+''; }
return ansiStyles[style].open + str + ansiStyles[style].close; }
var matchOperatorsRe = /[|\\{}()[\]^$+*?.]/g; var escapeStringRegexp = function (str) { if (typeof str !== 'string') { throw new TypeError('Expected a string'); } return str.replace(matchOperatorsRe, '\\$&'); }
function build(_styles) { var builder = function builder() { return applyStyle.apply(builder, arguments); }; builder._styles = _styles; // __proto__ is used because we must return a function, but there is // no way to create a function with a different prototype. builder.__proto__ = proto; return builder; }
var styles = (function () { var ret = {}; ansiStyles.grey = ansiStyles.gray; Object.keys(ansiStyles).forEach(function (key) { ansiStyles[key].closeRe = new RegExp(escapeStringRegexp(ansiStyles[key].close), 'g'); ret[key] = { get: function () { return build(this._styles.concat(key)); } }; }); return ret; })();
var proto = defineProps(function colors() {}, styles);
function applyStyle() { var args = arguments; var argsLen = args.length; var str = argsLen !== 0 && String(arguments[0]); if (argsLen > 1) { for (var a = 1; a < argsLen; a++) { str += ' ' + args[a]; } }
if (!colors.enabled || !str) { return str; }
var nestedStyles = this._styles;
var i = nestedStyles.length; while (i--) { var code = ansiStyles[nestedStyles[i]]; str = code.open + str.replace(code.closeRe, code.open) + code.close; }
return str; }
function applyTheme (theme) { for (var style in theme) { (function(style){ colors[style] = function(str){ if (typeof theme[style] === 'object'){ var out = str; for (var i in theme[style]){ out = colors[theme[style][i]](out); } return out; } return colors[theme[style]](str); }; })(style) } }
colors.setTheme = function (theme) { if (typeof theme === 'string') { try { colors.themes[theme] = require(theme); applyTheme(colors.themes[theme]); return colors.themes[theme]; } catch (err) { console.log(err); return err; } } else { applyTheme(theme); } };
function init() { var ret = {}; Object.keys(styles).forEach(function (name) { ret[name] = { get: function () { return build([name]); } }; }); return ret; }
var sequencer = function sequencer (map, str) { var exploded = str.split(""), i = 0; exploded = exploded.map(map); return exploded.join(""); };
// custom formatter methods colors.trap = require('./custom/trap'); colors.zalgo = require('./custom/zalgo');
// maps colors.maps = {}; colors.maps.america = require('./maps/america'); colors.maps.zebra = require('./maps/zebra'); colors.maps.rainbow = require('./maps/rainbow'); colors.maps.random = require('./maps/random')
for (var map in colors.maps) { (function(map){ colors[map] = function (str) { return sequencer(colors.maps[map], str); } })(map) }
defineProps(colors, init());
Step by Step Solution
There are 3 Steps involved in it
Step: 1
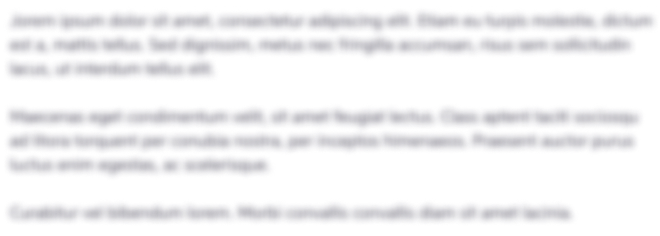
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started