Question
Using only C language For this assignment you will implement and solve a classical computer science problem known as The Towers of Hanoi. The Towers
Using only C language
For this assignment you will implement and solve a classical computer science problem known as The
Towers of Hanoi. The Towers of Hanoi is a simple stacking game that will be introduced at the beginning
of the lab. The rules are as follows:
1. You can only move one disk at a time
2. You can never put a larger disk on top of a smaller disk
Include the following structure definition at the top of your program:
typedef struct disk{
int disk_size;
struct disk* next;
struct disk* prev;
}disk;
Implement the following functions:
void push(disk** head, disk* new_disk)
The push function takes the address of the head of a stack and a new disk to push to the stack as inputs. It
simply pushes the new disk to the stack and returns nothing. Make sure that the head of the stack gets
changed to the new disk.
disk* pop(disk** stack)
The pop function takes the address of the head of a stack and pops the top node off of the stack. It
returns the node that it removed from the top of the stack. It also changes the head of the stack.
void move_stack(int level, disk** from, disk** to, disk** spare)
The move_stack function takes all three stacks and an integer value to keep track of your recursion. When
you call this function in main, the level parameter should be the number of disks. YOU MUST MAKE
THIS FUNCTION RECURSIVE. You can try to make it iterative, but you will be wasting your time.
int main( int argc, char **argv)
The main function takes the number of disks as the single command line argument. You should error
check to ensure that the number of disks is between 3 and 9 inclusive. The 3 to 9 range is important
because less than 3 disks is a trivial case and anything more than 9 disks starts to take way too many
moves. After error checking the command line argument, you should set up three stacks to be the rods
of the game. You will be given a function to initialize the game on one of the rods and a function to
print the game. After the game is set up and printed, you should call your move_stack function to solve
the game. Finally, you should print the game again to confirm it worked. Dont forget to free all of your
memory and error check everything you need to error check.
Sample Outputs
Characters in bold case are inputs from user.
[kpsgf7@tc-m610-login-node623 lab6]$
compile lab6.c
[kpsgf7@tc-m610-login-node623 lab6]$
./a.out
IMPROPER COMMAND LINE ARGUMENTS!
./a.out
[kpsgf7@tc-m610-login-node623 lab6]$
./a.out 15
IMPROPER NUMBER OF DISKS!
DISK NUMBER MUST BE BETWEEN 3 AND 9 INCLUSIVE!
[kpsgf7@tc-m610-login-node623 lab6]$
./a.out 2
IMPROPER NUMBER OF DISKS!
DISK NUMBER MUST BE BETWEEN 3 AND 9 INCLUSIVE!
[kpsgf7@tc-m610-login-node623 lab6]$
./a.out 3
NEW GAME
1 - -
2 - -
3 - -
_______________
SOLVED GAME
- - 1
- - 2
- - 3
_______________
[kpsgf7@tc-m610-login-node623 lab6]$
./a.out 6
NEW GAME
1 - -
2 - -
3 - -
4 - -
5 - -
6 - -
_______________
SOLVED GAME
- - 1
- - 2
- - 3
- - 4
- - 5
- - 6
_______________
[kpsgf7@tc-m610-login-node623 lab6]$
./a.out 9
NEW GAME
1 - -
2 - -
3 - -
4 - -
5 - -
6 - -
7 - -
8 - -
9 - -
_______________
SOLVED GAME
- - 1
- - 2
- - 3
- - 4
- - 5
- - 6
- - 7
- - 8
- - 9
_______________
Step by Step Solution
There are 3 Steps involved in it
Step: 1
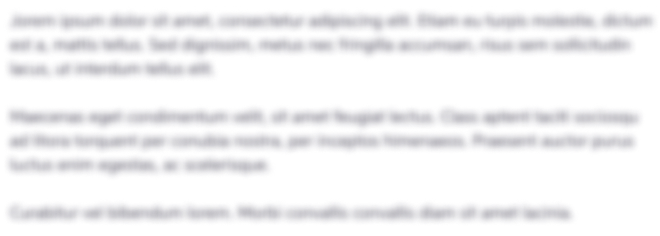
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started