Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using only DrRacket In this part you will implement your own limited version of eval. Your version will only understand a very limited subset of
Using only DrRacket
In this part you will implement your own limited version of eval. Your version will only understand a very limited subset of Racket, with expressions of the following kind:
expr ::lambda var expr ; lambda function definition
expr expr ; function application
var ; variable reference
Note that a lambda may only have one parameter.
Your evaluation function will be passed sexpressions of the above form the base form will always be a lambda and should return corresponding Racket values. Because your evaluator will use Racket features to represent and implement this subset of the Racket language, it is a socalled metacircular evaluator.
To implement this, you will need to take apart the input to your evaluator and determine which form it is before building the corresponding Racket form. In order to implement variables which are just symbols in the input you will also need to keep track of their bindings in an environment we recommend using the associative list which you implemented above.
Here's the evaluator in action:
myeval lambda x x
myeval lambda flambda xf f x sqr
We've stubbed out portions of the evaluator for you as a guide. Feel free to deletekeep what you wish.
define myeval rexp
let myevalenv rexp rexp
env ; environment assoc list
cond symbol rexp ; variable
void
eqfirst rexp 'lambda ; lambda expression
void
else ; function application
void
Here are the test cases DO NOT CHANGE THESE, and please make sure they pass:
testcase "testmyeval
define rexp lambda x x
define val myeval rexp
define res val
checkequal? res
testcase "testmyeval
define rexp lambda x xlambda y y
define val myeval rexp
define res val
checkequal? res
testcase "testmyeval
define exp lambda f
lambda x
f f x
define val myeval exp
define res val lambda x x x
checkequal? res
Step by Step Solution
There are 3 Steps involved in it
Step: 1
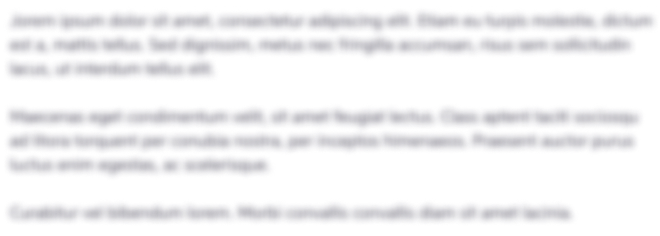
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started