Question
Using priority queues to implement a loan approval system for a financial institute that funds startups. Problem: Our financial institute receives many loan applications and
Using priority queues to implement a loan approval system for a financial institute that funds startups.
Problem:
Our financial institute receives many loan applications and rejects them only if the applicant doesn't qualify (so generous!) otherwise the applications will be added to a list (called active list) and will be approved if the budget is available. The loan approval system allows the following
commands:
- Load the applications: Reads the information of the applications from a file and store them as active applications if they qualify (if an application does not meet the requirements it will be added to the rejected application list)
- Set the budget: Update the current budget (the input for this command is some amount of budget that will be added to the current budget of the institute. At the beginning the current budget should be initialized as 0).
- Make a decision: given the current budget, make a decision about active applications (approve as many applications as possible: i.e remove them from the active application list and add them to the approved list).
- Print: print the list of active applications (no decision made so far), approved applications and rejected applications in three separate log files (approved.txt, rejected.txt, active.txt).
- Update an application: Applicants can update their application later by providing more documents. This may only affect active applications. For this command you can assume the information is given in a file (exactly the same format as input applications for command Load)
Application:
New applications are stored in a file which is the input of load or update command. Each application contains different fields (separated by tab 't') and will be stored as a separate line in the input file. The following are the application's fields:
- Applicant's full name (can be stored as a string)
- Loan amount that is requested
- Years of relevant education
- Years of relevant experience
- Estimated annual profit: it is a list of estimated profit for the financial institute from the
customers' payback in each year (for up to 30 years). Note that different applicants may
differ in the length of estimated annual profit.
Approval process:
The financial institute will keep applications in three lists. Once the applications are read from
the input file, they will be checked to see if they meet the requirements or not and based on that
added to the active application list or rejected list. If the sum of education and experience years is
smaller than 10, the application will be rejected. Otherwise the application will get some score
and will be added to the active list. The following formula is used to calculate the score of active
applications:
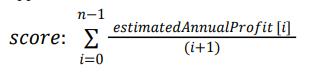
where es
timatedAnnualProfit[i] is the estimation of annual profit for year i after approval (it is a
part of the application form).
Note that in the case of update command, the score of the application (if in active list) will be
calculated again and it will be updated in the active list (you do not need to search in the rejected
list to update an application. if the application was rejected before it is like a new application).
Sample Input
The following is an example of an input file:
Each line is one application and different fields of each application are separated by tab
("t"). For example, in the first application (the first line), the applicant's full name is "Maryam
Siahbani", the requested loan is 150000$, years of relevant education is 10, and years of relevant
experience is 3, and estimated annual profit is [0, 0, 24000, 40000, 50000, 24000]. So for this
applicant the score will be calculated as 0*1/1 + 0* 1/2 + 24000 * 1/3 + 40000* 1/4 + 50000 *
1/5 + 24000* 1/6 = 32000.
A sample input file will be posted to Blackboard as an example, make sure you follow the input
format.
Small hint on reading file: When you read each line of the file you can split it based on "t" to
get all the tokens as a list.
Note that the approval of the applications depends on their priority scores and the available
budget. For example in the above example, assume that the institute budget is set to 300000, then
the input file is given to the loan-approval application and then command "make decision" is
requested and finally command print. The result in the active.txt, approved.txt and
rejected.txt files will be as follows:
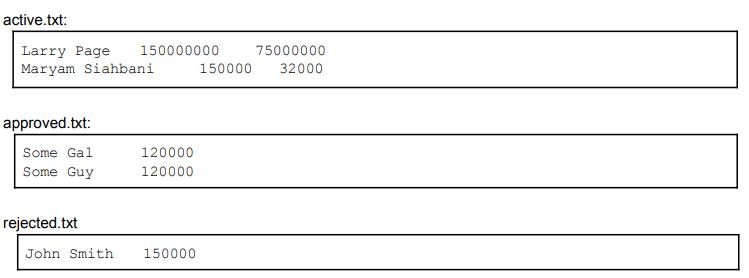
Note that although the priority score of "Larry Page" was higher than others it was not approved,
due to lack of budget. The applications in the active list will be printed based on the priority
score (sorted in decreasing order). The last field in each line of active.txt is the priority score.
Guideline:
You need to implement and submit at least 4 classes:
- Applicant: this class keeps the information for each applicant, name, education,
experience,... . This class should also have a field called score (see the Approval
process for the details). This class should implement interface Comparable (define
method compareTo() which compares regarding the value of score).
- PriorityQueue: your priority queue should be a max heap (you did this in lab8). I
posted a max heap on Blackboard along with this assignment. You can use that. Study all
the methods in this class properly.
- Loan: This is the main class for loan approval. This class should keep three lists: active
applications, approved applications and rejected applications. The approved and rejected
lists can be a regular ADT List (you can use ArrayList in Java API). The active
application list should be a priority queue (an object of PriorityQueue class). In
class Loan you should have a method called run() which prints the options for the user
and executes them.
- test: This class is just for testing. It will include method main, just create an object of
class Loan and test it. I posted an example test file (You can use it as is - do not change
it).
Requirements:
Make sure your final submission passes all the following requirements to get the full mark:
- Your code should run without error.
- Your loan application properly communicates with the user (print the possible
commands, takes the commands and executes them).
- Your loan application has all the commands mentioned above (properly working).
File-1
public class Applicant implements Comparable {
private String name;
private int score;
// add the rest of fields (education, experience, annual profit)
//Complete this constructor
// you should calculate this.score based on estimated annual profit
public Applicant() {
//Complete this constructor
// you should calculate this.score based on estimated annual profit
}
public int compareTo(Applicant other){
if (this.score > other.score)
return 1;
else if (this.score < other.score)
return -1;
else
return 0;
// you can just write it in one line:
// return this.score - other.score
}
// this method will be used to find an applicant
public boolean equals(Object other) {
if (!(other instanceof Applicant)){
return false;
}
return this.name.equals(((Applicant)other).name);
}
public String toString() {
//complete this method
//it will be useful for print option in class Loan
return "";
}
// add any other method you need
}
File-2
import java.util.ArrayList;
public class Loan {
private PriorityQueue activeList;
private ArrayList approvedList;
private ArrayList rejectedList;
private int budget;
public Loan () {
activeList = new PriorityQueue();
approvedList = new ArrayList();
rejectedList = new ArrayList();
budget = 0;
}
public void run() {
/* print the options for the Loan app and execute them
*
* Possible operations:
* Load applications
* Set the budget
* Make a decision
* Print
* Update an application (there is a method find() in PriorityQueue
* that you can use to find the applicant
* you would like to update)
*/
}
}
FIle-3
Maryam Siahbani 150000 10 3 0 0 24000 40000 50000 24000
John Smith 150000 6 3 0 0 25000 50000 50000 25000
Larry Page 150000000 8 25 0 150000000
Some Guy 120000 4 13 0 60000 90000 40000
Some Gal 120000 7 10 0 60000 90000 40000
File-4
public class test {
public static void main( String [ ] args )
{
Loan testObject = new Loan();
testObject.run();
}
}
n-1 score: i=0 estimated AnnualProfit [i] (i+1)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
Here is the code for the Applicant class Java public class Applicant implements Comparable private String name private int score private int education private int experience private List estimatedAnnu...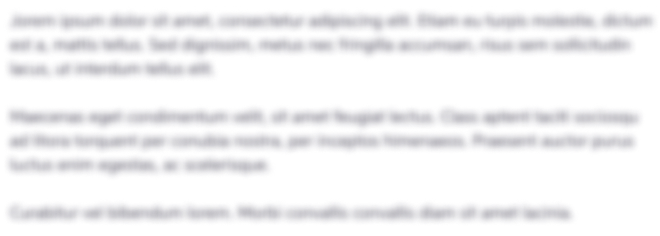
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started