Question
Using Python #1 You have been given a stepladder and a small robot that can climb up and down that ladder, one step at a
Using Python
#1
You have been given a stepladder and a small robot that can climb up and down that ladder, one step at a time. The robot can be programmed using a language that contains exactly three commands, which are always given using uppercase letters:
C (climb) causes the robot to move one step up the ladder. If the robot attempts to climb up from the top step of the ladder, it falls off the ladder and is destroyed.
D (descend) causes the robot to move one step down the ladder. If the robot is already on the first step of the ladder, nothing happens (it stays in place).
R (reset) causes the robot to immediately return to the first step of the ladder.
Given a size (total number of steps) for the ladder and a sequence of commands for the robot, your task is to determine how many instructions will be executed before the robot falls to its doom from the top of the ladder. You may assume that every instruction sequence always leads to the destruction of the unfortunate robot. You may also assume that every instruction sequence only contains the three commands listed above. Put your solution into the ladder() function that we have provided for you.
The robot always begins on the first step (step 1) of the ladder.
For example, suppose that you have a 4-step ladder and the instruction sequence CDDCCRCCCCCCCDDDD. In this case:
1. The robot first moves up one step to step 2. 2. The robot moves down one step to step 1. 3. The robot attempts to move down another step. It is already on the first step, so nothing happens. 4. The robot moves up one step to step 2. 5. The robot moves up one step to step 3. 6. The robot resets and moves back to step 1. 7. The robot moves up one step to step 2. 8. The robot moves up one step to step 3. 9. The robot moves up one step to step 4. Note that this is the top step of the ladder.
10. The robot attempts to move up one step and falls off the ladder.
Thus, the robot self-destructs after 10 instructions. The rest of the instruction sequence is ignored.
Function Call
ladder(4, "CDDCCCCCRCCCCDDDD") : (return value) = 7
ladder(1, "DDRCDCC") : (return value) = 4
ladder(1, "C") : (return value) = 1
#2
Pac-Man is a video game character who normally eats dots, power pellets, and the occasional ghost. Recently, hes fallen on hard times and has been reduced to (mainly) eating letters of the alphabet. Pac-Man can eat most letters of the alphabet, but he is unable to digest any of the characters in the word GHOST (uppercase or lowercase). When he reaches one of these characters in a string, he loses his appetite and stops eating.
Your job is to complete the pacman() function, which traces Pac-Mans progress through a string of uppercase and lowercase letters (with no spaces, digits, or symbols) and prints out the final state of the string. Use under- scores ( ) to represent consumed characters and a less-than sign (<) to represent Pac-Mans final position (either at the very beginning or end of the string, or right before the character that stopped him). Finally, your output should include any uneaten part of the string.
For example, consider the string cat. Pac-Man can eat the c and the a, but stops when he reaches t (because it is one of the letters in GHOST). Thus, the final string will be Hint #1: Use two while loops to solve this problem: one for the characters before Pac-Man stops, and one for any letters that remain in the input after he stops eating. Hint #2: If Pac-Man stops eating in the middle of the input, you will need to print him instead of the last character that he successfully consumed. Depending on how you approach this problem, you may find that string slicing is helpful to remove the final character of a string. To slice a string, follow the string variables name with square brackets; inside the brackets, write the starting index, followed by a colon (:), followed by the ending index. The slice will contain all of the characters from the starting index up to but not including the ending index. For example, "helloworld"[3:7] will return the string lowo. Here, your ending index should probably be something like length of string - 1. Function Call pacman(pacmancanbeawinner) = (return value) '_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _<' #1 test case print('Testing ladder() for steps = 4 and commands = "CDDCCCCCRCCCCDDDD": ' + str(ladder(4, "CDDCCCCCRCCCCDDDD"))) print('Testing ladder() for steps = 1 and commands = "DDRCDCC": ' + str(ladder(1, "DDRCDCC"))) print('Testing ladder() for steps = 1 and commands = "C": ' + str(ladder(1, "C"))) #2 test case print('Testing pacman() for text="pacmancanbeawinner": ' + str(pacman("pacmancanbeawinner"))) print('Testing pacman() for text="pacmanloses": ' + str(pacman("pacmanloses"))) print('Testing pacman() for text="Helloworld": ' + str(pacman("Helloworld")))pacman(pacmanloses) = (return value) '_ _ _ _ _ _
pacman(Helloworld) = (return value) '
Step by Step Solution
There are 3 Steps involved in it
Step: 1
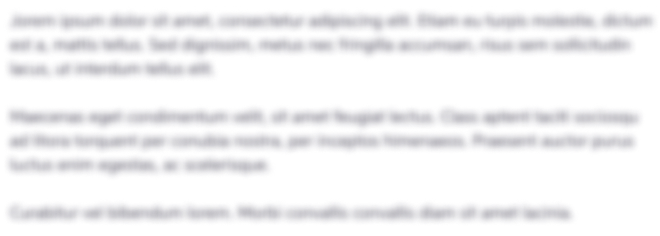
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started