Question
Using Python 8.20 DevelopaclassBankAccountthatsupportsthesemethods: __init__(): Initializes the bank account balance to the value of the input argument, or to 0 if no input argument is
Using Python
8.20 DevelopaclassBankAccountthatsupportsthesemethods:
__init__(): Initializes the bank account balance to the value of the input argument, or to 0 if no input argument is given
withdraw(): Takes an amount as input and withdraws it from the balance
deposit(): Takes an amount as input and adds it to the balance
balance(): Returns the balance on the account
>>> x = BankAccount(700) >>> x.balance() 700.00 >>> x.withdraw(70)
>>> x.balance() 630.00 >>> x.deposit(7) >>> x.balance() 637.00
8.40 InProblem8.20,therearesomeproblemswiththeimplementationoftheclassBankAccount, and they are illustrated here:
>>> x = BankAccount(-700) >>> x.balance() -700 >>> x.withdraw(70)
>>> x.balance() -770 >>> x.deposit(-7) >>> x.balance() Balance: -777
The problems are: (1) a bank account with a negative balance can be created, (2) the with- drawal amount is greater than the balance, and (3) the deposit amount is negative. Modify the code for the BankAccount class so that a ValueError exception is thrown for any of these violations, together with an appropriate message: 'Illegal balance', 'Overdraft', or 'Negative deposit'.
>>> x = BankAccount2(-700) Traceback (most recent call last): ... ValueError: Illegal balance
8.41 InProblem8.40, a generic Value Error exception is raised if any of the three violations occur. It would be more useful if a more specific, user-defined exception is raised instead. Define new exception classes NegativeBalanceError, OverdraftError, and DepositError that would be raised instead. In addition, the informal string representa- tion of the exception object should contain the balance that would result from the negative balance account creation, the overdraft, or the negative deposit.
For example, when trying to create a bank account with a negative balance, the error message should include the balance that would result if the bank account creation was al- lowed:
>>> x = BankAccount3(-5)
Traceback (most recent call last):
...
NegativeBalanceError: Account created with negative balance -5
When a withdrawal results in a negative balance, the error message should also include the balance that would result if the withdrawal was allowed:
>>> x = BankAccount3(5)
>>> x.withdraw(7)
Traceback (most recent call last):
...
OverdraftError: Operation would result in negative balance -2
If a negative deposit is attempted, the negative deposit amount should be included in the error message:
>>> x.deposit(-3)
Traceback (most recent call last):
...
DepositError: Negative deposit -3
Finally, reimplement the class BankAccount to use these new exception classes instead of ValueError.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
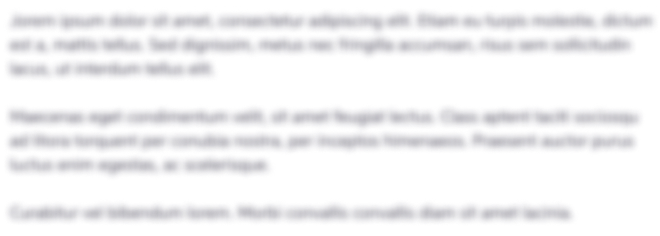
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started