Question
Using Python: *************I already have the code, i just need help with part 3 of the code.**************** Code: #Assignment - 1 # Part 1 def
Using Python:
*************I already have the code, i just need help with part 3 of the code.****************
Code:
#Assignment - 1
# Part 1 def intersect (list1, list2): result=[] for i in range(0,len(list1)): for j in range (0,len(list2)): if list1[i]==list2[j]: result.append(list1[i]) break return result #output [1, 9, 25] print(intersect([1,3,5,7,9,11,13,15,17,19,21,23,25],[1,4,9,16,25]))
#Part 2 def gregoryLiebniz(n): sum = 0.0 unit = 1.0 for i in range (1,n+1): lower = 2*i-1 sum += unit/lower unit = -unit return 4*sum
a=gregoryLiebniz(100000) print a #output 3.14159255359
#Part 3 krogg=Character('Krogg',180,0,20,20) glinda=Character('Glinda',120,0,5,20,5) Geoffrey=Character('Geoffrey',150,0,15,15) party=[krogg,glinda,geoffrey] enemy1=Character('Spider 1',50,100,10,1) enemy2=Character('Spider 2',50,100,10,1) enemy3=Character('Wolf 1',100,250,15,5) enemy4=Character('Wolf 2',100,250,15,5) enemy5=Character('Bear 1',200,350,20,10) enemy6=Character('Bear 2',200,350,20,10) enemy7=Character('Red Dragon',300,800,30,20) enemy8=Character('Blue Dragon',400,1000,35,20) enemies=[enemy1,enemy2,enemy3,enemy4,enemy5,enemy6,enemy7,enemy8]
party(bossHP >= 0):
print'Round',round
def party (bossHP >= 0):
print 'Round', round
# Krogg Attack if partyDead[0] == False: bossDamage = partyAttack[0] - bossDefense bossHP -= bossDamage print 'Krogg does ',bossDamage ,'points of damage to Spider' else: print 'Krogg cannot attack because he is dead'
# Geoffrey Attack if partyDead[2] == False: bossDamage = partyAttack[2] - bossDefense bossHP -= bossDamage print 'Geoffrey does ',bossDamage ,'points of damage to Spyder' else: print 'Geoffrey cannot attack because he is dead'
# Glinda Heal if partyDead[1] == False: for i in range(len(partyHP)): if partyDead[i] == False: partyHP[i] = partyHP[i] + 5 else: print 'Glinda cannot heal, because',partyNames[i] ,'is dead' else: print 'Glinda cannot heal, because she is dead'
# Boss Attack for i in range(len(partyHP)): if partyDead[i] == False: partyDamage = bossAttack - partyDefense[i]] partyHP[i] = partyHP[i] - partyDamage print 'The Boss cannot attack, because',partyNames[i] ,'is dead' else: print 'The Party is dead, you lose.'
round += 1
********************************************************************
Part 1:
The intersection () of two sets (s1, s2) is the set of all elements that are in s1 and are also in s2. Write a function (intersect) that takes two lists as input (you can assume they have no duplicate elements), and returns the intersection of those two sets (as a list) without using the in operator or any built-in functions, except for range() and len(). Write some code to test your function, as well.
Note: Do not use the in operator for this assignment. The key word in used in for loops (e.g. for x in list) is allowed, as that is not the same as using the in operator. The following code, which uses the in operator, would not be permitted, since it makes this question too easy:
if elem in set2: # do something
Sample Output for Part 1:
>>> intersect([1,3,5,7,9,11,13,15,17,19,21,23,25], [1,4,9,16,25]) [1, 9, 25]
Part 2
For the second part of this lab, we will write a function (gregoryLiebniz) that will estimate , using a di erent approach to that used in the labs. The Gregory-Liebniz series, developed by James Gregory and Gottfried Liebniz, provides an approximation to that gets better, the more terms you consider. While ine cient, it is quite simple. The Gregory-Liebniz series is given by the following formula:
Your function will take one argument (numIterations), which determines how many steps to take (i.e. how many terms to sum), and returns the approximation for .
Try to nd a value for numIterations that gives a reasonable approximation (e.g. 6 correct decimal places), yet takes only a few seconds to execute.
Sample Output for Part 2:
>>> gregoryLiebniz(10000000) 3.14159255359
Note: You may wish to disable this code when testing the other parts of this assignment, as many iterations of this algorithm can take a very long time to execute.
Part 3
For this part of the assignment, we will add a level up system to our RPG characters. Use the code for the Character class from the labs as a starting point.
Add an xpGained attribute to the Character class. This is how much XP would be gained by defeating this character in a battle. The value of xpGained will be passed into the constructor.
Add two more attributes, xp and level, to the Character class. The attribute xp is how much XP has been collected so far, and will be initialized to 0. The attribute level is the current level of the character, and will be initialized to 1.
Write a new function, gainXP, which will add some amount of experience to the character, possibly leveling them up as well. Adding the experience is simply a matter of adding it to the instance variable. To determine if the character levels up, however, we'll use a series of lists with values are described in the table below:
If the character's XP exceeds the minimum for a level, their level should be increased, along with their attack, defense, and magic power. All of these values should come from the tables, above.
Add code to the attack() function that will call gainXP with the appropriate arguments, when the attack results in defeating the other character.
Now, we'll simulate a huge battle. We're going to have a list of enemies to ght. Each enemy will ght your party one at a time, meaning only one enemy attacks per round (though your party can still all attack each round). You will need to modify the code of the battle loop to accommodate:
Move onto the next enemy when an enemy is defeated
Check to see if all enemies have been defeated to exit the loop (instead of just
the boss)
This program will be challenging to debug. It is recommended that you implement a better __str__ function and output every character's stats at the end of each round. You should also display messages for everything that happens (e.g. a character gaining XP, a character leveling up).
The following code sets up a pretty close battle, and you should use it for testing:
krogg = Character('Krogg', 180, 0, 20, 20) glinda = Character('Glinda', 120, 0, 5, 20, 5) geoffrey = Character('Geoffrey', 150, 0, 15, 15)
party = [krogg, glinda, geoffrey]
enemy1 = Character('Spider 1', 50, 100, 10, 1) enemy2 = Character('Spider 2', 50, 100, 10, 1) enemy3 = Character('Wolf 1', 100, 250, 15, 5) enemy4 = Character('Wolf 2', 100, 250, 15, 5) enemy5 = Character('Bear 1', 200, 350, 20, 10) enemy6 = Character('Bear 2', 200, 350, 20, 10) enemy7 = Character('Red Dragon', 300, 800, 30, 20) enemy8 = Character('Blue Dragon', 400, 1000, 35, 20)
enemies = [enemy1, enemy2, enemy3, enemy4, enemy5, enemy6, enemy7, enemy8]
Here is some sample output (using the above character stats):
Round 1
Action: Krogg does 19 points of damage to Spider 1 Geoffrey does 14 points of damage to Spider 1 Glinda heals 5 hp for Krogg Glinda heals 5 hp for Glinda Glinda heals 5 hp for Geoffrey Spider 1 does 0 points of damage to Krogg Spider 1 does 0 points of damage to Glinda Spider 1 does 0 points of damage to Geoffrey
Character status: Krogg (HP:180, XP:0, Level:1, Attack:20, Defense:20) Glinda (HP:120, XP:0, Level:1, Attack:5, Defense:20) Geoffrey (HP:150, XP:0, Level:1, Attack:15, Defense:15) Spider 1 (HP:17, XP:0, Level:1, Attack:10, Defense:1) Spider 2 (HP:50, XP:0, Level:1, Attack:10, Defense:1) Wolf 1 (HP:100, XP:0, Level:1, Attack:15, Defense:5) Wolf 2 (HP:100, XP:0, Level:1, Attack:15, Defense:5) Bear 1 (HP:200, XP:0, Level:1, Attack:20, Defense:10) Bear 2 (HP:200, XP:0, Level:1, Attack:20, Defense:10) Red Dragon (HP:300, XP:0, Level:1, Attack:30, Defense:20) Blue Dragon (HP:400, XP:0, Level:1, Attack:35, Defense:20)
The enemies are dead. You are victorious! ... more rounds ... Round 59
Action: Krogg does 12.5 points of damage to Blue Dragon Geoffrey cannot attack because he/she is dead. Glinda cannot heal because he/she is dead.
5
Blue Dragon does 7.5 points of damage to Krogg Blue Dragon does 15 points of damage to Glinda Glinda has been defeated. Blue Dragon gained 0 XP.
Blue Dragon does 12.5 points of damage to Geoffrey Geoffrey has been defeated. Blue Dragon gained 0 XP.
Character status: Krogg [DEAD] Glinda [DEAD] Geoffrey [DEAD] Spider 1 [DEAD] Spider 2 [DEAD] Wolf 1 [DEAD] Wolf 2 [DEAD] Bear 1 [DEAD] Bear 2 [DEAD]
Red Dragon [DEAD] Blue Dragon (HP:5.0, XP:0, Level:1, Attack:35, Defense:20)
Your whole party is dead. You lose.3 5 7 9 11' 3 5 7 9 11
Step by Step Solution
There are 3 Steps involved in it
Step: 1
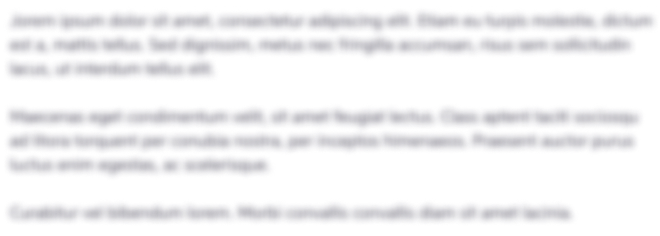
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started