Question
Using Scala perform the followig: Create a hierarchy of classes to represent items to be found in a store or a shopping cart. The top
Using Scala perform the followig: Create a hierarchy of classes to represent items to be found in a store or a shopping cart. The top of this hierarchy consists of the class StoreItem, which implements the characteristics that are common to both its sub-classes: UnitItem and WeightItem.
For this application, we would like to model the items that are sold by the unit and those that are sold by weight. In particular, the number of units must be represented with an integer, whereas the weight is represented with the type double.
All the items must have a unique serial number, a description and a method that calculates the total price for this item.
In your .scala file for this assignment, you must include the following: class StoreItem, object StoreItem, class UnitItem, class WeightItem, and object Test.
StoreItem
Create an abstract class called StoreItem with the following characteristics.
Fields
a val called serialNumber to store the item's serial number.
a val called description of type String to store the item's description.
Constructor
There will be one constructor (the primary constructor), which will take a parameter called description of type String. In the body of the constructor (i.e., in your class definition) the value of the object's serial number is set to the last object's serial number plus one (+1). Implement this functionality using a companion object.
Methods
an abstract method getPrice(), whose implementation depends on the type of item and will thus be overridden in StoreItem's subclasses.
UnitItem
Create a class, called UnitItem, that inherits the characteristics of its superclass StoreItem and has the following ones as well.
Fields
a val of type int to represent the number of units of this item.
a val of type double that represents the price per unit.
Constructor
The constructor of the class has three arguments: the description, the number of units and the price per unit, which are used to initialize the fields of the class.
Methods
a method getPrice() that calculates the price as the product of the number of units and the unit price. This method will return a double.
WeightItem
Create a class, called WeightItem, that inherits the characteristics of its superclass StoreItem and has the following ones as well.
Fields
a val of type double to represent the weight of this item.
a val of type double to represent the price per unit of weight.
Constructor
The constructor of the class has three arguments: the description, the weight and the price per unit of weight, which are used to initialize the fields of the class.
Methods
a method getPrice() that calculates the price as the product of the number of units and the unit price. This method will return a double.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
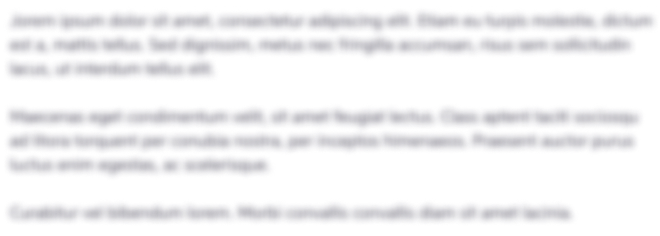
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started