Question
Using the 3 files below, create the C++ function that will manipulate a 600x600 image to... 1. The original image should be resized twice, down
Using the 3 files below, create the C++ function that will manipulate a 600x600 image to...
1. The original image should be resized twice, down to both 400x400 and 200x200 atop the new 600x600 P6 image. The function prototype for the resizings should be: void resizeImage(image_t*, image_t* );
2. The 400x400 image should be negative, have its colors inverted.
***************************************
// driver.c //
#include
#include
#include "ppm_info.h"
int main(int argc, char* argv[])
{
if(argc != 4)
{
printf("USAGE: ");
return -1;
}
FILE* originalImage = fopen(argv[1], "r");
FILE* newImage = fopen(argv[2], "w");
FILE* newImageP3 = fopen(argv[3], "w");
if(!originalImage || !newImage || !newImageP3)
{
printf("ERROR: File(s) could not be opened. ");
return -1;
}
image_t* image = read_ppm(originalImage);
header_t hdr = {"P6", 600, 600, 255};
image_t* imageNew = allocate_memory(hdr);
resizeImage(image, imageNew);
write_p6(newImage, imageNew);
//write_p6(newImage, image);
//write_p3(newImageP3, image);
return 0;
}
******************************************************************
// ppm_info.c //
#include "ppm_info.h"
header_t read_header(FILE* image_file)
{
header_t header;
fscanf(image_file, "%s %d %d %d ",
header.magicNum, &header.width, &header.height, &header.maxVal);
return header;
}
void write_header(FILE* out_file, header_t header)
{
fprintf(out_file, "%s %d %d %d ",
header.magicNum, header.width, header.height, header.maxVal);
}
image_t* read_ppm(FILE* image_file)
{
header_t header = read_header(image_file);
image_t* image = NULL;
image = read_pixels(image_file, header);
return image;
}
image_t* read_pixels(FILE* image_file, header_t header) {
int row = 0;
int col = 0;
unsigned char red,green,blue;
red = green = blue = 0;
image_t* image= allocate_memory(header);
for(row = 0; row < header.height; row++)
{
for(col = 0; col < header.width; col++)
{
fscanf(image_file, "%c%c%c", &red, &green, &blue);
image->pixels[row][col].r = red;
image->pixels[row][col].g = green;
image->pixels[row][col].b = blue;
}
}
return image;
}
image_t* allocate_memory(header_t header)
{
int row;
/*First allocate memory for the image itself. The image has a header and
*a double pointer for the pixels */
image_t* image = (image_t*) malloc(sizeof(image_t));
/*After you have allocated the memory for the image you can now set the header
*equal to the header passed in.*/
image->header = header;
/*Now we need to allocate the memory for the actual pixels. This is a 2d
*array so allocate for the 2D array. */
image->pixels = (pixel_t**) malloc(sizeof(pixel_t*) * header.height);
for(row = 0; row < header.height; row++)
{
image->pixels[row] = (pixel_t*)malloc(sizeof(pixel_t) * header.width);
}
return image;
}
void write_p6(FILE* out_file, image_t* image)
{
int row = 0;
int col = 0;
//unsigned char red,green,blue;
header_t header = image->header;
strcpy(header.magicNum,"P6");
write_header(out_file, header);
//red = green = blue = 0;
/*Loop through the image and print each pixel*/
for(row = 0; row < header.height; row++)
{
for(col = 0; col < header.width; col++)
{
fprintf(out_file, "%c%c%c",
image->pixels[row][col].r,
image->pixels[row][col].g,
image->pixels[row][col].b);
}
}
}
void write_p3(FILE* out_file, image_t* image)
{
int row = 0;
int col = 0;
//unsigned char red,green,blue;
header_t header = image->header;
strcpy(header.magicNum,"P3");
write_header(out_file, header);
//red = green = blue = 0;
/*Loop through the image and print each pixel*/
for(row = 0; row < header.height; row++)
{
for(col = 0; col < header.width; col++)
{
fprintf(out_file, "%d %d %d ",
image->pixels[row][col].r,
image->pixels[row][col].g,
image->pixels[row][col].b);
}
}
}
void resizeImage(image_t* old, image_t* resized)
{
double relW = (double)(old->header.width/resized->header.width);
double relH; //do the same as above with height
int row, col;
for(row = 0; row < resized->header.height; row++)
{
for(col = 0; col < resized->header.width; col++)
{
//calculate newrow and newcol
//set resized->pixels[row][col] = old->pixels[newrow][newcol];
}
}
}
************************************************************************
// ppm_info.h //
#ifndef PPM_INFO
#define PPM_INFO
#include
#include
#include
// First meaningful line of the PPM file
typedef struct header {
char magicNum[3];
int width, height, maxVal;
} header_t;
// Represents an RGB pixel with integer values between 0-255
typedef struct pixel {
unsigned char r, g, b;
} pixel_t;
// PPM Image representation
typedef struct image {
header_t header;
pixel_t** pixels;
} image_t;
header_t read_header(FILE* image_file);
void write_header(FILE* out_file, header_t header);
image_t* read_ppm(FILE* image_file);
image_t* read_pixels(FILE* image_file, header_t header);
image_t* allocate_memory(header_t header);
void write_p6(FILE* out_file, image_t* image);
void write_p3(FILE* out_file, image_t* image);
/*Add resizeImage prototype from specification document*/
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
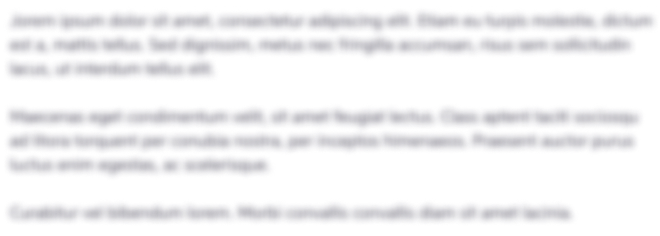
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started