Question
Using the classes provided below (they are in paste bin links, so you can copy paste them into a project), make a JUnit Test case
Using the classes provided below (they are in paste bin links, so you can copy paste them into a project), make a JUnit Test case for the method:
public int findPackageOrder(String trackingNumber)
The method is in ShippingStore.java, please make the test case in the class testShippingStore.java
https://pastebin.com/3RMVD8Xe - MainApp.java
package cs3354.kcg27;
import java.io.FileReader; import java.io.PrintWriter; import java.util.Scanner;
/** * This is the main class of the ShippingStore database manager. It provides a * console for a user to use the 5 main commands. * * @author Junye Wen */ public class MainApp {
/** * This method will begin the user interface console. Main uses a loop to * continue doing commands until the user types '6'. A lot of user input * validation is done in the loop. At least enough to allow the interface * with ShippingStore to be safe. * * @param args this program expects no command line arguments * @throws Exception */ public static void main(String[] args) throws Exception { Scanner in = new Scanner(System.in);
// Read database from file. ShippingStore shippingstore = new ShippingStore(); FileReader dataReader = new FileReader(shippingstore.getDataFile()); shippingstore.read(dataReader);
// Console user intererface String welcomeMessage = " Welcome to the Shipping Store database. Choose one of the following functions: " + "\t1. Show all existing package orders in the database " + "\t2. Add a new package order to the database. " + "\t3. Delete a package order from a database. " + "\t4. Search for a package order (given its Tracking #). " + "\t5. Show a list of orders within a given weight range. " + "\t6. Exit program. ";
System.out.println(welcomeMessage);
int selection = in.next().charAt(0); in.nextLine();
while (selection != '6') {
switch (selection) { case '1': shippingstore.showPackageOrders(); break; case '2': System.out.println(" Please type description of package with the following pattern: " + " TRACKING # TYPE SPECIFICATION CLASS WEIGHT VOLUME " + "example: GFR23 Box Books Retail 9500.00 45 "); String inTemp = in.nextLine();
String temp[] = inTemp.split(" ");
if (temp.length != 6) { System.out.println("Not correct number of fields to process."); break; }
shippingstore.addOrder(temp[0], temp[1], temp[2], temp[3], temp[4], temp[5]); break; case '3': shippingstore.showPackageOrders();
System.out.println(" Please enter the tracking # of the package order to delete from the database. "); String orderToDelete = in.nextLine(); shippingstore.removeOrder(orderToDelete); break; case '4': System.out.println(" Enter the Tracking # of the order you wish to see. "); String trackingNum = in.next(); in.nextLine(); shippingstore.searchPackageOrder(trackingNum); break; case '5': float high = 0; float low = 0; System.out.println(" Enter lower-bound weight. "); low = in.nextFloat(); System.out.println(" Enter upper-bound weight. "); high = in.nextFloat(); in.nextLine(); shippingstore.showPackageOrdersRange(low, high); break; case 'h': System.out.println(welcomeMessage); break; default: System.out.println("That is not a recognized command. Please enter another command or 'h' to list the commands."); break;
}
System.out.println("Please enter another command or 'h' to list the commands. "); selection = in.next().charAt(0);
in.nextLine(); }
in.close(); // Before exiting the program, save the data from the main memory to the // data file. PrintWriter pw = new PrintWriter("PackageOrderDB.txt"); shippingstore.flush(pw); System.out.println("Done!");
} }
https://pastebin.com/xKQdZdNM - PackageOrder.java
package cs3354.kcg27;
/**
* This class is a very simple representation of a package order. There are only getter
* methods and no setter methods and as a result a package order cannot be mutated once
* initialized. A package order object can also call the two override methods
* toString()
and equals()
*
* @author Junye Wen
*/
public class PackageOrder {
private final String trackingnumber;
private final String type;
private final String specification;
private final String mailingclass;
private final float weight;
private final int volume;
/**
* This constructor initializes the package order object. The constructor provides no
* user input validation. That should be handled by the class that creates a
* package order object.
*
* @param trackingnumber a String
that represents the tracking number
*
* @param type a String
that represents the type.
* Types: Postcard, Letter, Envelope, Packet, Box, Crate, Drum, Roll, Tube.
*
* @param specification a String
that represents the specification.
* Specification: Fragile, Books, Catalogs, Do-not-Bend, N/A - one per package
*
* @param mailingclass a String
that represents the mailing class
* Mailing class: First-Class, Priority, Retail, Ground, Metro.
*
* @param weight a float
that represents the weight of the package in oz
*
* @param volume an int
that represents the volume of the package in
* cubic inches, calculated as Width x Length x Height
*
*/
public PackageOrder(String trackingnumber, String type, String specification, String mailingclass, float weight, int volume) {
this.trackingnumber = trackingnumber;
this.type = type;
this.specification = specification;
this.mailingclass = mailingclass;
this.weight = weight;
this.volume = volume;
}
/**
* This method returns the package order's tracking number.
*
* @return a String
that is the tracking number of the package order.
*/
public String getTrackingNumber() {
return trackingnumber;
}
/**
* This method returns the package order's type.
*
* @return a String
that is the package order's type.
*/
public String getType() {
return type;
}
/**
* This method returns the package order's specification.
*
* @return a String
that is the package order's specification.
*/
public String getSpecification() {
return specification;
}
/**
* This method returns the package order's mailing class.
*
* @return a string
that is the package order's mailing class
*/
public String getMailingClass() {
return mailingclass;
}
/**
* This method returns the package's weight.
*
* @return a float
that is the package's weight
*/
public float getWeight() {
return weight;
}
/**
* This method returns the package's volume.
*
* @return an int
that is the package's volume
*/
public int getVolume() {
return volume;
}
/**
* This method returns the package order's fields as a string representation.
*
* @return a String
that lists the fields of the package order
* object delineated by a space and in the same order as the constructor
*/
@Override
public String toString() {
return trackingnumber + " " + type + " " + specification + " " + mailingclass + " "
+ String.format("%.2f", weight) + " " + volume + " ";
}
/**
* This method provides a way to compare two package order objects.
*
* @param c a PackageOrder
object that is used to compare to
* this
package order. Two orders are equal if their TrackingNumber is the
* same.
* @return the boolean
value of the comparison.
*/
public boolean equals(PackageOrder c) {
return c.getTrackingNumber().equals(this.trackingnumber);
}
}
https://pastebin.com/G5BhFz5h - ShippingStore.java
package cs3354.kcg27;
import java.io.IOException;
import java.io.FileReader;
import java.io.PrintWriter;
import java.io.File;
import java.io.Reader;
import java.io.Writer;
import java.util.ArrayList;
import java.util.Scanner;
/**
* This class is used to represent a database interface for a list of
* Package Order
's. It using a plain-text file "PackageOrderDB.txt"
* to store and write package order objects in readable text form. It contains
* an ArrayList
called packageOrerList
to store the
* database in a runtime friendly data structure. The packageOrerList
* is written to "PackageOrderDB.txt" at the end of the ShippingStore
object's
* life by calling flush()
. This class also provides methods for
* adding, remove, and searching for shipping orders from the list.
*
* @author Junye Wen
*/
public class ShippingStore {
private final File dataFile;
private ArrayList
/**
* This constructor is hard-coded to open "PackageOrderDB.txt
" and
* initialize the packageOrerList
with its contents. If no such file
* exists, then one is created. The contents of the file are "loaded" into
* the packageOrerList ArrayList in no particular order. The file is then closed
* during the duration of the program until flush()
is called.
* @throws IOException
*/
public ShippingStore() throws IOException {
dataFile = new File("PackageOrderDB.txt");
packageOrderList = new ArrayList<>();
// If data file does not exist, create it.
if (!dataFile.exists()) {
System.out.println("Data file does not exist, creating one now . . .");
//if the file doesn't exists, create it
PrintWriter pw = new PrintWriter(dataFile);
//close newly created file so we can reopen it
pw.close();
}
}
/**
* Method that returns a reference to the data file.
* @return dataFile
*/
public File getDataFile() {
return dataFile;
}
/**
* Method showPackageOrer displays the current list of package orders in the Arraylist in no
* particular order.
*
*/
public void showPackageOrders() {
showPackageOrders(packageOrderList);
}
/**
* Private method used as an auxiliary method to display a given ArrayList
* of package orders in a formatted manner.
*
* @param orders the package order list to be displayed.
*/
private void showPackageOrders(ArrayList
System.out.println(" -------------------------------------------------------------------------- ");
System.out.println("| Tracking # | Type | Specification | Class | Weight(oz) | Volume |");
System.out.println(" -------------------------------------------------------------------------- ");
for (int i = 0; i < orders.size(); i++) {
System.out.println(String.format("| %-11s| %-8s| %-14s| %-12s| %-11s| %-7s|",
orders.get(i).getTrackingNumber(),
orders.get(i).getType(),
orders.get(i).getSpecification(),
orders.get(i).getMailingClass(),
String.format("%.2f", orders.get(i).getWeight()),
Integer.toString(orders.get(i).getVolume())
));
}
System.out.println(" -------------------------------------------------------------------------- ");
}
/**
* This method displays package orders that have a weight within the range of
* low
to high
.
*
* @param low a float that is the lower bound weight.
* @param high a float that is the upper bound weight.
*/
public void showPackageOrdersRange(float low, float high) {
ArrayList
for (PackageOrder order : packageOrderList) {
if ((low <= order.getWeight()) && (order.getWeight() <= high)) {
orders.add(order);
}
}
if (orders.isEmpty())
System.out.println("No packages found with weight within the given range. ");
else
showPackageOrders(orders);
}
/**
* This method can be used to find a package order in the Arraylist of orders.
*
* @param trackingNumber a String
that represents the tracking number
* of the order that to be searched for.
* @return the int
index of the package orders in the Arraylist of orders,
* or -1 if the search failed.
*/
public int findPackageOrder(String trackingNumber) {
int index = -1;
for (int i = 0; i < packageOrderList.size(); i++) {
String temp = packageOrderList.get(i).getTrackingNumber();
if (trackingNumber.equalsIgnoreCase(temp)) {
index = i;
break;
}
}
return index;
}
/**
* This method can be used to search for a package order in the Arraylist of orders.
*
* @param trackingNumber a String
that represents the tracking number
* of the order that to be searched for.
*/
public void searchPackageOrder(String trackingNumber) {
int index = findPackageOrder(trackingNumber);
if (index != -1) {
ArrayList
order.add(getPackageOrder(index));
System.out.println(" Here is the order that matched: ");
showPackageOrders(order);
} else {
System.out.println(" Search did not find a match. ");
}
}
/**
* This method is used to add a package order to the orderList ArrayList. In order for a
* package order to be added to the ArrayList it must comply with the following:
*
* 1. The order is not already in the ArrayList according to the tracking number
* as the unique key.
*
* 2. The TrackningNumber string matches the following regular expression:
* "[A-Za-z0-9]{5}"
or in other words: it
* is 5 alphanumeric characters.
*
* 3. The Type of the order can be only one of the following:
* Postcard, Letter, Envelope, Packet, Box, Crate, Drum, Roll, Tube.
*
* 4. The Specification of the order can be only one of the following:
* Fragile, Books, Catalogs, Do-not-Bend, N/A.
*
* 5. The Mailing Class of the order can be only one of the following:
* First-Class, Priority, Retail, Ground, Metro.
*
* 6. The Weight must be non-negative.
*
* 7. The Volume must be non-negative.
* @param toAdd the PackageOrder
object to add to the
* packageOrerList
*/
public void addOrder(String trackingnumber, String type, String specification, String mailingclass, String weight, String volume) {
if (this.findPackageOrder(trackingnumber) != -1) {
System.out.println("Package Order already exists in database. ");
return;
}
if (!trackingnumber.matches("[A-Za-z0-9]{5}")) {
System.out.println("Invalid Tracking Number: not proper format."
+ "Tracking Number must be at least 5 alphanumeric characters.");
return;
}
if (!(type.equals("Postcard") || type.equals("Letter") || type.equals("Envelope")
|| type.equals("Packet") || type.equals("Box")|| type.equals("Crate")
|| type.equals("Drum")|| type.equals("Roll")|| type.equals("Tube"))) {
System.out.println("Invalid type: "
+ "Type must be one of following: "
+ "Postcard, Letter, Envelope, Packet, Box, Crate, Drum, Roll, Tube.");
return;
}
if (!(specification.equals("Fragile") || specification.equals("Books") || specification.equals("Catalogs")
|| specification.equals("Do-not-Bend") || specification.toUpperCase().equals("N/A"))) {
System.out.println("Invalid specification: "
+ "Specification must be one of following: "
+ "Fragile, Books, Catalogs, Do-not-Bend, N/A.");
return;
}
if (!(mailingclass.equals("First-Class") || mailingclass.equals("Priority") || mailingclass.equals("Retail")
|| mailingclass.equals("Ground") || mailingclass.equals("Metro")) ) {
System.out.println("Invalid Mailing Class: "
+ "Mailing Class must be one of following: "
+ "First-Class, Priority, Retail, Ground, Metro.");
return;
}
if (Float.parseFloat(weight) < 0) {
System.out.println("The weight of package cannot be negative.");
return;
}
if (!volume.matches("[0-9]{1,6}")) {
System.out.println("Invalid volume: "
+ "The package's volume has to be an integer number between 0 and 999999. ");
return;
}
//If passed all the checks, add the order to the list
packageOrderList.add(new PackageOrder(trackingnumber, type, specification, mailingclass,
Float.parseFloat(weight), Integer.parseInt(volume)));
System.out.println("Package Order has been added. ");
}
/**
* This method will remove an order from the packageOrerList
ArrayList. It
* will remove the instance of an order that matches tracking number that was
* passed to this method. If no such order exists, it will produce an error message.
*
* @param toDelete the PackageOrder
object to be removed.
*/
public void removeOrder(String trackingNum) {
int orderID = findPackageOrder(trackingNum);
if (orderID == -1) {
System.out.println(" Action failed. No package order with the given tracking # exist in database. ");
}
else {
packageOrderList.remove(orderID);
System.out.println(" Action successful. Package order has been removed from the database. ");
}
}
/**
* This method is used to retrieve the PackageOrder object from the
* PackageOrderList
at a given index.
*
* @param i the index of the desired PackageOrder
object.
* @return the PackageOrder
object at the index or null if the index is
* invalid.
*/
public PackageOrder getPackageOrder(int i) {
if (i < packageOrderList.size() && i >= 0) {
return packageOrderList.get(i);
} else {
System.out.println("Invalid Index. Please enter another command or 'h' to list the commands.");
return null;
}
}
/**
* This method reads data from the FileReader provided as input and puts them
* in the packageOrderList.
* @param dataReader The input FileReader to read from.
* @throws IOException If any problem occurs with the data input.
*/
public void read(Reader dataReader) throws IOException {
Scanner orderScanner = new Scanner(dataReader);
//Initialize the Array List with package orders from PackageOrderDB.txt
while (orderScanner.hasNextLine()) {
// split values using the space character as separator
String[] temp = orderScanner.nextLine().split(" ");
packageOrderList.add(new PackageOrder(temp[0], temp[1], temp[2], temp[3],
Float.parseFloat(temp[4]), Integer.parseInt(temp[5])));
}
//Package order list is now in the ArrayList completely so we can close the file
orderScanner.close();
}
/**
* This method accepts a Writer
to a file and overwrites it with a text representation of
* all the package orders in the PackageOrderList
.
* This should be the last method to be called before exiting the program.
* @param dataWriter The data to write in the file.
* @throws IOException
*/
public void flush(Writer dataWriter) throws IOException {
for (PackageOrder c : packageOrderList) {
dataWriter.write(c.toString());
}
dataWriter.close();
}
public int multiply (int x) {
return x * x;
}
}
https://pastebin.com/8yzHXJDh - testShippingStore.java
package cs3354.kcg27;
import static org.junit.Assert.assertEquals;
import java.io.File;
import java.io.IOException;
import org.junit.Before;
import org.junit.Test;
public class testShippingStore {
@Before
public void before() throws IOException {
ShippingStore testShippingStore = new ShippingStore();
testShippingStore.addOrder("12121", "Postcard", "Fragile", "Priority", "3.25", "3");
}
@Test
public void testGetDataFile() throws IOException {
ShippingStore testShippingStore = new ShippingStore();
File testFile = testShippingStore.getDataFile();
final File dataFiles;
dataFiles = new File("PackageOrderDB.txt");
assertEquals("The data files are not the same", testFile, dataFiles);
}
@Test
public void testShowPackageOrders() {
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
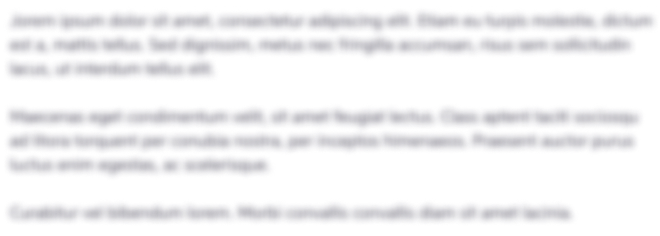
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started