Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using the code from the recitation on a doubly linked circular list shown below modify the code to read integers from STDIN and to walk
Using the code from the recitation on a doubly linked circular list shown below modify the code to read integers from STDIN and to walk backwards from the "head".
All output will be to STDOUT.
Submit the java file and a screen shot (PDF) of the program running the command to walk backwards from the "head".
/****************************************************************************** * Compilation: javac Circ2List.java * Execution: java Circ2List * Data files: not applicable * * Provides methods to insert at the end, the beginning, and after * an integer in the doubly linked circular list. * * This program builds & prints a circular list of integers that are * hardcoded in the source code. * * Sample output * % java Circ2List * Walk from start to end * 4 500 650 70 908 * * Lab #1 goals: * 1. Add ability to read integers from STDIN * 2. Add command to print list backward from "head" * * All output is to STDOUT. * ******************************************************************************/ import java.util.*; class Circ2List { static Node start; // Structure of a Node static class Node { int data; Node next; Node prev; }; // Function to insert at the end static void insertEnd(int value) { // If the list is empty, create a single node // circular and doubly list if (start == null) { Node new_node = new Node(); new_node.data = value; new_node.next = new_node.prev = new_node; start = new_node; return; } // If list is not empty /* Find last node */ Node last = (start).prev; // Create Node dynamically Node new_node = new Node(); new_node.data = value; // Start is going to be next of new_node new_node.next = start; // Make new node previous of start (start).prev = new_node; // Make last preivous of new node new_node.prev = last; // Make new node next of old last last.next = new_node; } // Function to insert Node at the beginning // of the List, static void insertBegin(int value) { // Pointer points to last Node Node last = (start).prev; Node new_node = new Node(); new_node.data = value; // Inserting the data // setting up previous and next of new node new_node.next = start; new_node.prev = last; // Update next and previous pointers of start // and last. last.next = (start).prev = new_node; // Update start pointer start = new_node; } // Function to insert node with value as value1. // The new node is inserted after the node with // with value2 static void insertAfter(int value1, int value2) { Node new_node = new Node(); new_node.data = value1; // Inserting the data // Find node having value2 and next node of it Node temp = start; while (temp.data != value2) temp = temp.next; Node next = temp.next; // insert new_node between temp and next. temp.next = new_node; new_node.prev = temp; new_node.next = next; next.prev = new_node; } static void display() { Node temp = start; System.out.printf(" Walk from start to end "); while (temp.next != start) { System.out.printf("%d ", temp.data); temp = temp.next; } System.out.printf("%d ", temp.data); } /* Driver code*/ public static void main(String[] args) { /* Start with the empty list */ Node start = null; // Insert 500. Linked list becomes 500, .null insertEnd(500); // Insert 4 at the beginning. Linked list becomes 4, 500 insertBegin(4); // Insert 7 at the end. Linked list becomes 4, 500, 70 insertEnd(70); // Insert 908 at the end. Linked list becomes 4, 500, 70, 908 insertEnd(908); // Insert 6, after 5. Linked list becomes 4, 500, 650, 70, 908 insertAfter(650, 500); display(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
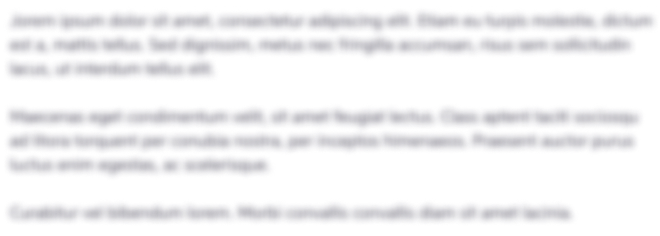
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started