Question
Using the constructors and in the File IO in c++ forms. This small project is geared to test your understanding of File IO. File IO
Using the constructors and in the File IO in c++ forms.
This small project is geared to test your understanding of File IO.
File IO
We have been working on a simple address book. The major problem with it at this point is that we lose all of our information when the program ends. It would be nice to store all of this information to a file. That way, the data will always be there for us.
Your mission is to write all of the entries of your address book to a text file. When you write the file you should write one person per line comma separated. For example
Glenn,Stevenson,1313 Mockingbird Lane,951-639-5532 Jane,Smith,30333 South Street,951-555-5325
This way you can use the comma as a delimiter for each field of the record.
In your constructors, you should have the functionality to load the address book from file and put the contents into your vector. This means you will have to parse out each field for each record. You should also have a save method that will save the entire contents of the vector to the file formatted.
If you are writing this project in C++ you may want to investigate the idea of writing the contents of the address book to file inside a destructor. This is not required here, only something to think about.
In any event, you must have functionality that will write the contents of your address book to file and have a methodology of saving it.
here are some steps to help you
You should not be creating a person array. You should use your constructor to initialize the address book with input from a file. I haven't started the assignment yet, but from reading over the instructions, this will likely be my approach:
- Create an overloaded AddressBook constructor with a string parameter for the file name
- Create an AddressBook destructor for writing the vector to the file
- Create in the address book class a method for reading files
- Create in the address book class a method for writing to files
- Have my new constructor call the read file method, passing the file name
- Have the read file, well read the file, and call setPerson to add names to the vector using a while loop
- To test functionality, I will mostly use the code from previous assignments and add a couple more Person/Player to the vector
- Destructor will call my write to file method when AddressBook object goes out of scope.
- I will then confirm that the file includes the original names plus the additions I made in main
Note: 1) In the the method for reading the file, the method will have to parse inputs from the file to sub strings. Dr. Stevenson recommended using a comma to aid in this. These sub strings should represent the first name, last name, and address. You can then pass the strings to the setPerson method for adding to vector.
2) Make sure you are testing that the file was opened; and, make sure you class the file before returning from the function.
-----Addressing your code above--------
You created an array of Person objects. You only wrote the first element of the array to the Book.txt file. You would need to use a loop to advance through the array...The reason that that each string is on its own line is because the outfile breaks at white space. And, you have inserted a blank space between each string.
I just finished reading the lecture and I haven't started the assignment, but I believe everything that I provided is accurate. If I am off, I am sure Dr. Steveson or Chad will catch it...
Hope this helps.
My person coding from assigmen 1
Person.h
#include
//declare class person class Person { //declare data members of class private: string firstName; string lastName; string address; //declare member functions of class public: void help(); Person(); Person(string first, string last); Person(string first, string last, string address); void setFirstName(string first); void setLastName (string last); void setAddress (string address); string getFirstName(); string getLastName(); string getAddress();
};
Person.cpp
#include "Person.h" #include
//function to reduce redundancy void Person::help() { //sets address to empty string address=""; } //default constructor Person::Person() { //sets first and last name to empty string and calls help function to set address to empty string firstName=""; lastName=""; help(); } //parameterized constructor Person::Person(string first, string last) { //set values of data members of the class firstName=first; lastName=last; help(); } //another parameterized constructor Person::Person(string first, string last, string address) { //set values of data members of the class firstName=first; lastName=last; address=address; } void Person::setFirstName(string first) { //set firstname firstName=first; } void Person::setLastName(string last) { //set lastname lastName=last; } void Person::setAddress(string address) { //set address this->address=address; } string Person::getFirstName() { //return first name return firstName; } string Person::getLastName() { //return lastname return lastName; } string Person::getAddress() { //return address return address; }
main.cpp
#include "Person.h" #include
void getPeople(Person a[]) { int i; string f,l,add; //for every Person for(int i=0;i<5;i++) { cout<<"Enter details of Person "<<(i+1)< void printPeople(Person a[]) { int i; cout<<"FirstName\tLastName\tAddress "< int main() { //declare array of class person of size 5 Person p[5]; //call function to get inputs getPeople(p); //call function to print array of class Person printPeople(p); } address book but I need addressbook coding into an address.cpp and address.h of c++ form because I don't understand java.Turn Java coding into c++ use the person coding. java please tur it into c++ //class AddressBook class AddressBook{ //Array list of persons private ArrayList //class to test AddressBook class public class AddressBookExample{ //main method public static void main(String ar[]){ //create AddressBook object AddressBook addressBook = new AddressBook("John", "William"); //set persons to the address book addressBook.setPerson("Mark", "Brown", "Lewisberg"); addressBook.setPerson("Kane", "Richardson", "New zealand"); //print the address book addressBook.print(); //find the person with last name Brown System.out.println(" Person with last name - Brown: "+ addressBook.findPerson("Brown")); //find the person with name Tim Cook System.out.println(" Person with name - Tim Cook: "+ addressBook.findPerson("Tim", "Cook")); //find the person with name John William System.out.println(" Person with name - John William: "+ addressBook.findPerson("John", "William")); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
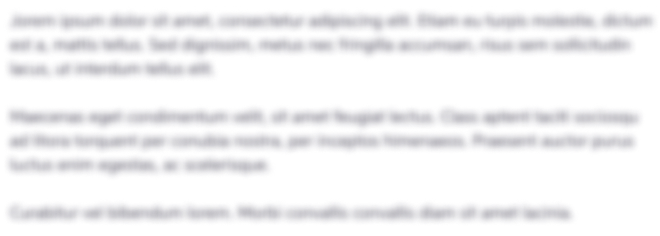
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started