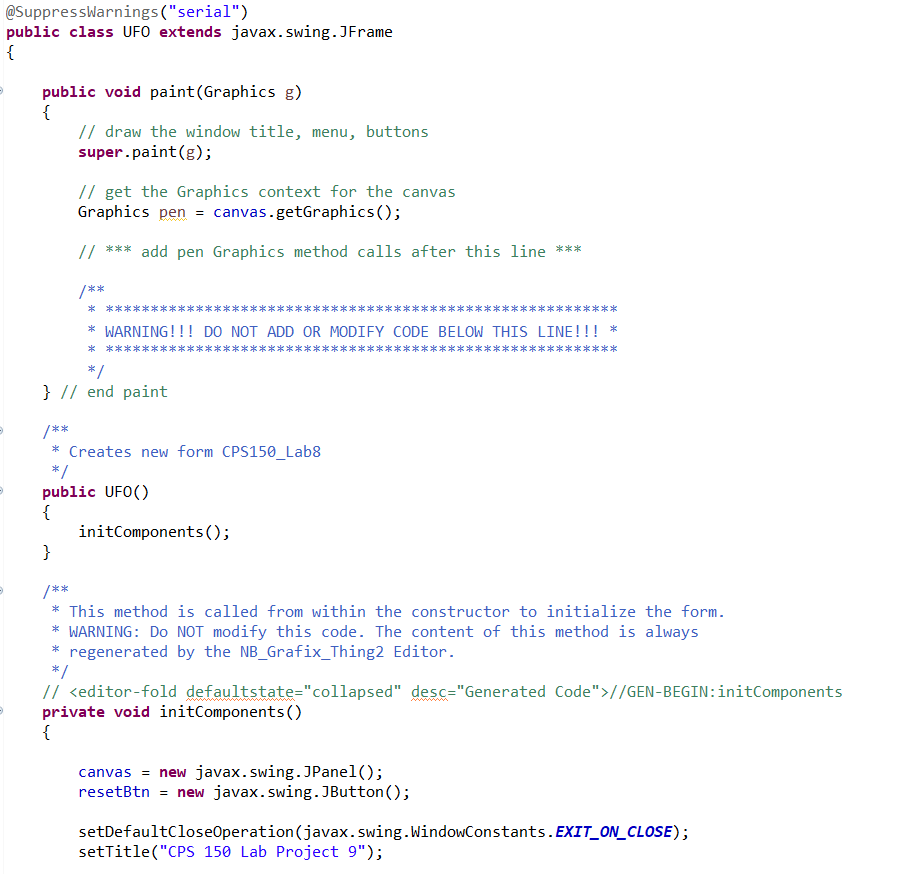
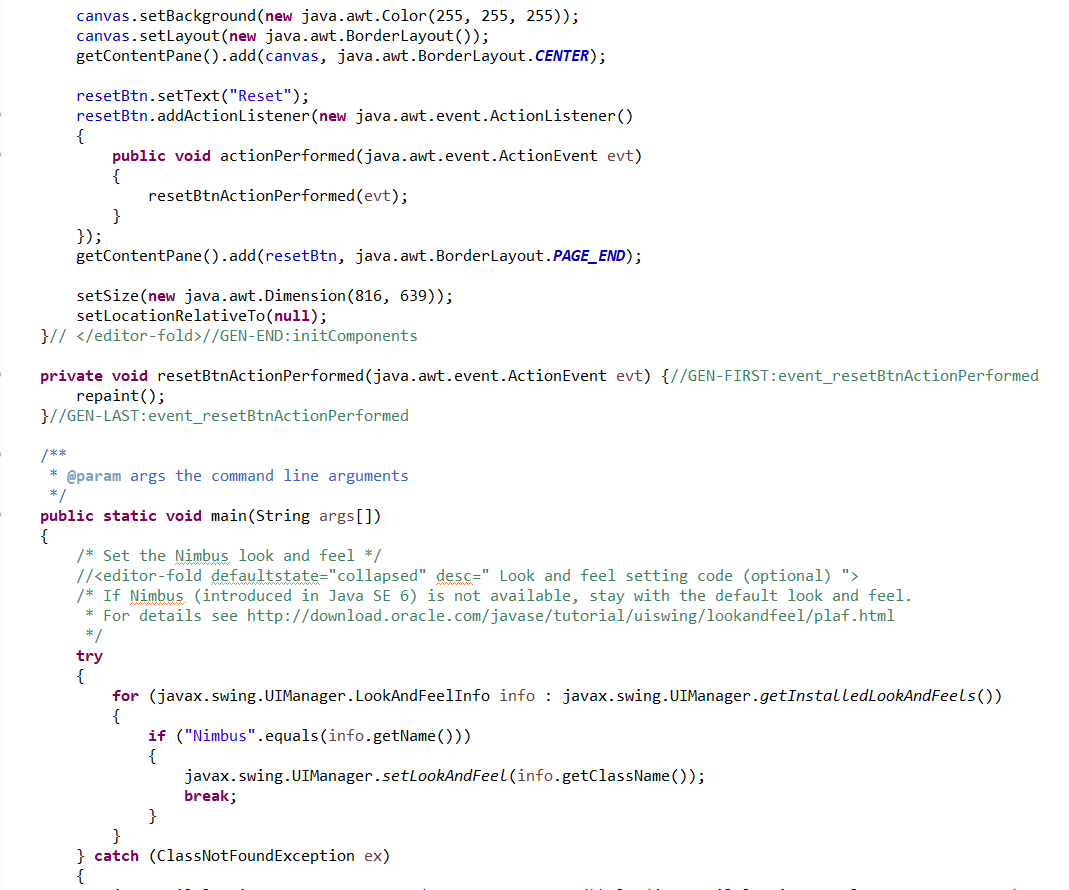
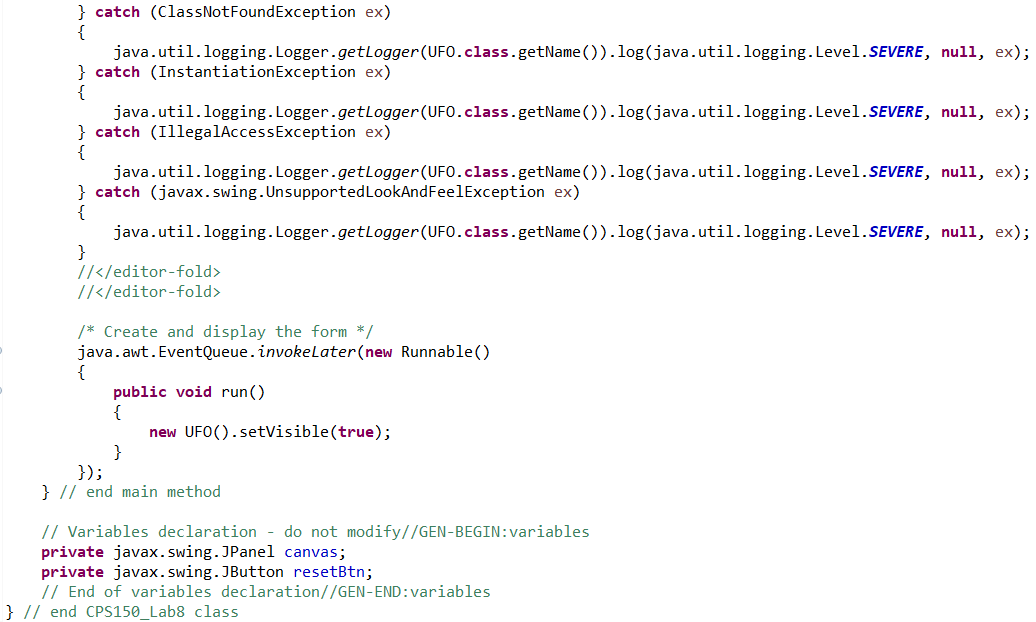
Using the documentation, design and write a program (in the Grafix_Thing's paint method, using the pen Graphics object) to draw a UFO similar to the following one.
@SuppressWarnings ("serial") public class UFO extends javax.swing.JFrame { public void paint(Graphics g) { // draw the window title, menu, buttons super.paint(g); // get the Graphics context for the canvas Graphics pen = canvas.getGraphics(); // *** add pen Graphics method calls after this line *** * ****** /** ****** * WARNING!!! DO NOT ADD OR MODIFY CODE BELOW THIS LINE!!! * ****** */ } // end paint * ****** /** * Creates new form CPS150_Lab8 */ public UFO() { initComponents(); } /** * * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always regenerated by the NB_Grafix_Thing2 Editor. */ //
//GEN-BEGIN:initComponents private void initComponents() { canvas = new javax.swing.JPanel(); resetBtn = new javax.swing.JButton(); setDefaultCloseOperation (javax.swing. WindowConstants.EXIT_ON_CLOSE); setTitle("CPS 150 Lab Project 9"); canvas.setBackground(new java.awt.Color(255, 255, 255)); canvas.setLayout(new java.awt.BorderLayout()); getContentPane().add(canvas, java.awt.BorderLayout.CENTER); resetBtn.setText("Reset"); resetBtn.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed (java.awt.event. ActionEvent evt) { resetBtnActionPerformed(evt); } }); getContentPane().add(resetBtn, java.awt.BorderLayout.PAGE_END); setSize(new java.awt.Dimension (816, 639)); setLocationRelativeTo(null); }// //GEN-END: initComponents private void resetBtnActionPerformed (java.awt.event.ActionEvent evt) {//GEN-FIRST:event_resetBtnActionPerformed repaint(); }//GEN-LAST:event_resetBtnActionPerformed /** * @param args the command line arguments public static void main(String args[]) { /* Set the Nimbus look and feel */ //
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel. * For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html */ try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalled LookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } catch (ClassNotFoundException ex) } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } catch (IllegalAccessException ex) java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } catch (javax.swing.Unsupported LookAndFeelException ex) { java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } // // /* Create and display the form */ java.awt. EventQueue.invokelater(new Runnable() { public void run() { new UFO().setVisible(true); } }); } // end main method // Variables declaration - do not modify//GEN-BEGIN: variables private javax.swing.JPanel canvas; private javax.swing. JButton resetBtn; // End of variables declaration//GEN-END: variables } // end CPS150_Lab8 class @SuppressWarnings ("serial") public class UFO extends javax.swing.JFrame { public void paint(Graphics g) { // draw the window title, menu, buttons super.paint(g); // get the Graphics context for the canvas Graphics pen = canvas.getGraphics(); // *** add pen Graphics method calls after this line *** * ****** /** ****** * WARNING!!! DO NOT ADD OR MODIFY CODE BELOW THIS LINE!!! * ****** */ } // end paint * ****** /** * Creates new form CPS150_Lab8 */ public UFO() { initComponents(); } /** * * This method is called from within the constructor to initialize the form. * WARNING: Do NOT modify this code. The content of this method is always regenerated by the NB_Grafix_Thing2 Editor. */ //
//GEN-BEGIN:initComponents private void initComponents() { canvas = new javax.swing.JPanel(); resetBtn = new javax.swing.JButton(); setDefaultCloseOperation (javax.swing. WindowConstants.EXIT_ON_CLOSE); setTitle("CPS 150 Lab Project 9"); canvas.setBackground(new java.awt.Color(255, 255, 255)); canvas.setLayout(new java.awt.BorderLayout()); getContentPane().add(canvas, java.awt.BorderLayout.CENTER); resetBtn.setText("Reset"); resetBtn.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed (java.awt.event. ActionEvent evt) { resetBtnActionPerformed(evt); } }); getContentPane().add(resetBtn, java.awt.BorderLayout.PAGE_END); setSize(new java.awt.Dimension (816, 639)); setLocationRelativeTo(null); }// //GEN-END: initComponents private void resetBtnActionPerformed (java.awt.event.ActionEvent evt) {//GEN-FIRST:event_resetBtnActionPerformed repaint(); }//GEN-LAST:event_resetBtnActionPerformed /** * @param args the command line arguments public static void main(String args[]) { /* Set the Nimbus look and feel */ //
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel. * For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html */ try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalled LookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } catch (ClassNotFoundException ex) } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } catch (IllegalAccessException ex) java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } catch (javax.swing.Unsupported LookAndFeelException ex) { java.util.logging.Logger.getLogger(UFO.class.getName()).log(java.util.logging. Level.SEVERE, null, ex); } // // /* Create and display the form */ java.awt. EventQueue.invokelater(new Runnable() { public void run() { new UFO().setVisible(true); } }); } // end main method // Variables declaration - do not modify//GEN-BEGIN: variables private javax.swing.JPanel canvas; private javax.swing. JButton resetBtn; // End of variables declaration//GEN-END: variables } // end CPS150_Lab8 class