Question
Using the driver program below answer the following questions: Question 1: Write a union method for the ResizableArrayBag class. The union of two bags is
Using the driver program below answer the following questions:
Question 1:
Write a union method for the ResizableArrayBag class.
The union of two bags is the combined contents of both bags. Unions are explained in more detail in Chapter 1, #5.
A union might contain duplicates.
The method should not alter either bag. The current bag and the bag sent in as a parameter should be the same when the method ends.
The method header is:
public BagInterface
Example:
bag1 contains (1, 2, 3)
bag2 contains (2, 2, 4, 5)
bag1.union(bag2) will return a new bag that contains (1, 2, 3, 2, 2, 4, 5).
The contents of bag1 and bag2 would be the same.
(The order of the union bag might not match the above, since bags are unordered.)
Question 2:
Write an intersection method for the ResizableArrayBag class.
The intersection of two bags is the overlapping content of the bags. Intersections are explained in more detail in Chapter 1, #6.
An intersecion might contain duplicates.
The method should not alter either bag. The current bag and the bag sent in as a parameter should be the same when the method ends.
The method header is:
public BagInterface
Example:
bag1 contains (1, 2, 2, 3)
bag2 contains (2, 2, 2, 4, 5)
then bag1.intersection(bag2) would return a new bag that contains (2, 2)
The contents of bag1 and bag2 would be the same.
(The order of the intersection bag might not match the above, since bags are unordered.)
Driver program:
import java.util.Arrays;
public class Homework04Driver {
public static void main(String[] args) {
// setting up an ArrayBag to test
System.out.println("TESTING BAG");
ArrayBag
numbersBag.add(1);
numbersBag.add(2);
numbersBag.add(1);
numbersBag.add(4);
numbersBag.add(3);
System.out.println("The bag contains[1, 2, 1, 4, 3] \t\t"
+ Arrays.toString(numbersBag.toArray()));
// Q3 replace method
// NOTE: The description does not specify that you must replace the last
// element in the array, so your output might be different- that's okay
System.out.println(" ***Q3***");
numbersBag.replace(6);
System.out.println("The bag contains[1, 2, 1, 4, 6] \t\t"
+ Arrays.toString(numbersBag.toArray()));
numbersBag.replace(8);
System.out.println("The bag contains[1, 2, 1, 4, 8] \t\t"
+ Arrays.toString(numbersBag.toArray()));
// Q4 removeEvery method
// NOTE: bags are unordered, so the order of your array might be
// different- that's okay as long as the contents match what is listed below
System.out.println(" ***Q4***");
numbersBag.add(1);
numbersBag.removeEvery(1);
System.out.println("The bag contains[8, 2, 4] (any order) \t\t"
+ Arrays.toString(numbersBag.toArray()));
numbersBag.removeEvery(3);
System.out.println("The bag contains[8, 2, 4] (any order) \t\t"
+ Arrays.toString(numbersBag.toArray()));
// Q5: union
System.out.println(" ***Q5***");
ResizableArrayBag
firstResizableBag.add(8);
firstResizableBag.add(2);
firstResizableBag.add(4);
firstResizableBag.add(5);
firstResizableBag.add(6);
firstResizableBag.add(2);
ResizableArrayBag
secondResizableBag.add(3);
secondResizableBag.add(1);
secondResizableBag.add(2);
BagInterface
.union(secondResizableBag);
System.out.println("Bag1 contains [8, 2, 4, 5, 6, 2] \t\t"
+ Arrays.toString(firstResizableBag.toArray()));
System.out.println("Bag2 contains [3, 1, 2] \t\t"
+ Arrays.toString(secondResizableBag.toArray()));
System.out.println("Union Bag contains [8, 2, 4, 5, 6, 2, 3, 1, 2] (any order) \t\t "
+ Arrays.toString(unionBag.toArray()));
// Q6: intersection
System.out.println(" ***Q6***");
firstResizableBag.add(2);
secondResizableBag.add(2);
secondResizableBag.add(4);
BagInterface
System.out.println("Bag1 contains [8, 2, 4, 5, 6, 2, 2] \t\t"
+ Arrays.toString(firstResizableBag.toArray()));
System.out.println("Bag2 contains [3, 1, 2, 2, 4] \t\t"
+ Arrays.toString(secondResizableBag.toArray()));
System.out.println("Intersection Bag contains [2, 4, 2] (any order) \t\t "
+ Arrays.toString(intersectionBag.toArray()));
// setting up AList to test
System.out.println(" TESTING LIST");
AList
produceList.add("banana");
produceList.add("date");
produceList.add("grape");
produceList.add("eggplant");
produceList.add("jicama");
produceList.add("grape");
// Q7: getPosition
// Note: this driver assumes you return -1 if an element is not in the list;
// it would also be valid to throw an exception
System.out.println(" ***Q7***");
System.out.println("Position is 1: " + produceList.getPosition("banana"));
System.out.println("Position is 3: " + produceList.getPosition("grape"));
System.out.println("Position is -1: " + produceList.getPosition("mango"));
//Q8: moveToBeginning
System.out.println(" ***Q8***");
System.out.println("List contains [banana, date, grape, eggplant, jicama, grape] \t\t "
+ Arrays.toString(produceList.toArray()));
produceList.moveToBeginning();
System.out.println("List contains [grape, banana, date, grape, eggplant, jicama] \t\t "
+ Arrays.toString(produceList.toArray()));
produceList.clear();
produceList.moveToBeginning();
System.out.println("List contains [] \t\t "
+ Arrays.toString(produceList.toArray()));
// Q9: equals
System.out.println(" ***Q9***");
produceList.add("banana");
produceList.add("potato");
produceList.add("jicama");
produceList.add("grape");
AList
foodList.add("banana");
foodList.add("potato");
foodList.add("jicama");
System.out.println("Result is false: " + produceList.equals(foodList));
foodList.add("grape");
System.out.println("Result is true: " + produceList.equals(foodList));
foodList.add("squash");
System.out.println("Result is false: " + produceList.equals(foodList));
foodList.replace(1, "jicama");
foodList.replace(3, "banana");
System.out.println("Result is false: " + produceList.equals(foodList));
System.out.println("The list contains[banana, potato, jicama, grape] \t\t "
+ Arrays.toString(produceList.toArray()));
System.out.println("The second list contains[jicama, potato, banana, grape, squash] \t\t\t"
+ Arrays.toString(foodList.toArray()));
/* un-comment this section if doing the extra credit */
/*
System.out.println(" TESTING EXTRA CREDIT");
AList
numberListA.add(1);
numberListA.add(5);
numberListA.add(6);
numberListA.add(8);
numberListA.add(7);
numberListA.add(9);
AList
numberListB.add(2);
numberListB.add(4);
numberListB.add(3);
numberListB.add(9);
numberListB.add(7);
System.out.println("List B is smaller (fewer elements), result should be positive: " + numberListA.compareTo(numberListB));
numberListA.remove(1);
numberListA.remove(1);
System.out.println("List A is smaller (fewer elements), result should be negative: " + numberListA.compareTo(numberListB));
numberListA.clear();
numberListA.add(1);
numberListA.add(3);
numberListA.add(5);
numberListA.add(7);
numberListA.add(10);
numberListB.clear();
numberListB.add(2);
numberListB.add(4);
numberListB.add(3);
numberListB.add(9);
numberListB.add(7);
System.out.println("List A is smaller (same number of elements, 1<2), result should be negative: " + numberListA.compareTo(numberListB));
numberListB.remove(1);
numberListB.add(1, -2);
System.out.println("List B is smaller (same number of elements, -2 < 1, result should be positive: " + numberListA.compareTo(numberListB));
numberListA.clear();
numberListA.add(1);
numberListA.add(2);
numberListA.add(3);
numberListA.add(10);
numberListA.add(9);
numberListB.clear();
numberListB.add(1);
numberListB.add(4);
numberListB.add(3);
numberListB.add(9);
numberListB.add(7);
System.out.println("Neither List A nor List B is smaller, result should be zero: " + numberListA.compareTo(numberListB));
*/
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
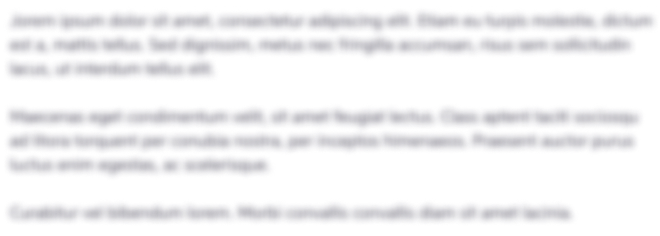
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started