Question
Using the following code Introduction: Pirate Time Your friend has not stopped talking about how cool pirates and how awesome it would be if they
Using the following code
Introduction: Pirate Time
Your friend has not stopped talking about how cool pirates and how awesome it would be if they could be a real-life pirate captain! To amuse your friend, you have decided to create a series of programs about pirates.
Problem: Ocean Voyage (oceanvoyage.c)
Armed with the map from the Old Pirates Treasure Chest, plenty of oranges, and the best landing crew possible, your friend is ready to depart Spain for the Caribbean in search of treasure. The trip will be long and dangerous.
Your friend must decide how they will travel: as a merchant, as a privateer, or as a pirate. Merchant vessels are fairly safe, but slow. Merchant sailors will be given extra gold for their journey from their investors at home. Privateer vessels are well armed and a bit faster. Financed by the government, privateers will also receive a little extra gold for the trip. Pirates have the fastest ships in the seas, but only have the gold they already possess.
As captain, your friend has decided to depart from Port Marin, Spain. They will travel along the coast of Spain and Portugal and make for the Canary Islands. They will be able to buy supplies in both of these ports. From the Canary Islands they can catch the trade winds to Grenada at the edge of the Caribbean Sea. This will be the last opportunity to get supplies before searching for the island on the Old Pirates map.
Between ports there are many dangers. Sailors may fall ill, storms and fog may plague the ship, and parts of the ship may even break down. These will cause delays and your friend must balance the need to get to the treasure with the need to keep their crew healthy. Make sure they purchase plenty of food and extra parts for the journey.
At the end of the journey your friend will note how many of the crew survived the trip and how many shovels they managed to bring to dig up the treasure.
You must fill in the functions that are currently empty. After you write each function, you should test it before moving on. The main function should not be modified for the final submission (you may modify it during testing, as long as you return it to its initial form).
Descriptions of each function are given in the skeleton along with the function Pre- and Post-conditions.
Restrictions
Although you may use other compilers, your program must compile and run using Code::Blocks. Your program should include a header comment with the following information: your name, course number, section number, assignment title, and date. Also, make sure you include comments throughout your code describing the major steps in solving the problem.
Using the code Below
/* Add your own header comment. */
//Included Libraries #include
//Constants for Arrays #define STRLENGTH 30 #define NUMCREW 5 #define NUMSUPPLIES 4
//Constants for Distances (measured in miles) #define CANARY 1261 #define GRENADA 3110 #define FINAL 500 #define DISTANCE 4871
//Function Signatures - do not change these void setup(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int supplies[NUMSUPPLIES], int *captaintype, int *funds, int *distanceperday); int countcrew(int crewstatus[NUMCREW]); void printstatus(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW]); void getsupplies(char supplytypes[NUMSUPPLIES][STRLENGTH], int supplies[NUMSUPPLIES], int *funds); void dailyreport(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int supplies[NUMSUPPLIES], int funds, int traveled); void rest(int supplies[NUMSUPPLIES], char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int *days); int fish(); int max(int a, int b); int min(int a, int b); void event(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int *days, int supplies[NUMSUPPLIES]);
//Main function - This is the final version of main. Any changes you make while //creating the functions should be removed prior to submission. int main(void) { //crewnames and supplytypes are arrays of strings to store the names of the crew members //and the types of supplies that can be purchased and taken on the voyage char crewnames[NUMCREW][STRLENGTH]; char supplytypes[NUMSUPPLIES][STRLENGTH] = {"Food", "Clothes", "Ship Parts", "Shovels"}; //stop indicates whether or not the user would like to stop at a port //crewstatus indicates the status of each crew member, corresponding to the order of names //supplies has a total for each type of supply, corresponding to the order of supplies char stop; int crewstatus[NUMCREW], supplies[NUMSUPPLIES]; //the distanceperday and funds depends on the captaintype the user selects //day is the current day, traveled is the total miles traveled, i is a loop counter //and action stores the intended action of the user for the day int distanceperday, captaintype, funds, traveled=0, day=1; int i, action;
//seed the pseudorandom number generator srand(time(0));
//initialize each variable with information from the user setup(crewnames, crewstatus, supplies, &captaintype, &funds, &distanceperday);
//begin the game by purchasing initial supplies printf(" Before leaving Port Marin, you should purchase some supplies. "); getsupplies(supplytypes, supplies, &funds);
//continue the voyage until the ship reaches the intended destination //if all crew members perish, the journey cannot continui while (traveled < DISTANCE && countcrew(crewstatus) > 0) { printf(" --It is day #%d.-- ", day);
//check to see if the ship has reached the next port if(traveled >= (GRENADA+CANARY) && traveled < (GRENADA + CANARY + distanceperday) ) { printf("You have arrived at Grenada, at the edge of the Carribbean Sea. "); printf("Would you like to make port? (Y/N) "); scanf(" %c", &stop);
if (stop == 'Y' || stop == 'y') getsupplies(supplytypes, supplies, &funds);
traveled = (GRENADA+CANARY) + distanceperday; } else if (traveled >= CANARY && traveled < (CANARY + distanceperday) ) { printf("You have arrived at the Canary Islands. "); printf("Would you like to make port? (Y/N) "); scanf(" %c", &stop);
if (stop == 'Y' || stop == 'y') getsupplies(supplytypes, supplies, &funds);
traveled = CANARY + distanceperday; } //if between destinations: print the daily report and process the user's action for the day else { dailyreport(crewnames, crewstatus, supplies, funds, traveled);
printf(" What would you like to do? "); printf("1 - Fish "); printf("2 - Rest "); printf("3 - Continue "); scanf("%d", &action);
if(action == 1) { supplies[0] += fish(); } else if(action == 2) { day--; rest(supplies, crewnames, crewstatus, &day); } else { traveled += distanceperday; supplies[0] = max(supplies[0] - countcrew(crewstatus) * 2, 0); event(crewnames, crewstatus, &day, supplies); } } day++; }
printf(" ");
//The final printout changes based on which condition broke the while loop if (countcrew(crewstatus) == 0) { printf("Your crew has perished in the search for treasure. :( ");
printstatus(crewnames, crewstatus); } else { printf("Your crew has made it safely to the island. ");
printstatus(crewnames, crewstatus);
if(supplies[3] >= countcrew(crewstatus)) printf("You have enough shovels to dig up the treasure! "); else printf("Unfortuantely, you will not be able to dig up the treasure. "); }
return 0; }
//Pre-conditions: none //Post-conditions: each input parameter should be assigned an initial value
//What to do in this function: Provide the starting message for the user and ask how they plan to travel. // Based on their response initialize captaintype, funds, and distanceperday // -captaintype 1 should get 1000 funds and 80 distanceperday // -captaintype 2 should get 900 funds and 90 distanceperday // -captaintype 3 should get 800 funds and 100 distanceperday
// Ask the user for their name. Place this in the first row of crewnames, representing the captain. Treat // crewnames as a 1-Dimensional array of strings. As an example: printf("%s", crewnames[0]); would print the // first string or the captains name to the screen. Then ask for the names of the other 4 crew members in a loop.
// Set the crew status to 2 for each crew member, representing healthy // Set the initial amount of supplies to be 0 for each supply void setup(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int supplies[NUMSUPPLIES], int *captaintype, int *funds, int *distanceperday) {
}
//Pre-conditions: crewstatus is an array of numerical indicators for the status of each crew member // 0 - deceased, 1 - ill, 2 - healthy //Post-conditions: returns the number of crew members that are alive
//What to do in this function: Traverse the crew status array and count how many crew members // have a status that is not 0. Return this count. int countcrew(int crewstatus[NUMCREW]) {
}
//Pre-conditions: crew names is an array of strings for the crew members // crewstatus is an array of numerical indicators for the status of each crew member // 0 - deceased, 1 - ill, 2 - healthy //Post-conditions: none
//What to do in this function: print each crew members name and their status. // You may use a status array to shorten this process: char status[3][STRLENGTH] = {"Deceased", "Ill", "Healthy"}; void printstatus(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW]) {
}
//Pre-conditions: supplytypes in an array of strings and gives the name of each supply type // supplies is an array of integers representing how many of each type the crew has // funds represents how many gold pieces the crew has to spend //Post-conditions: the user may choose to buy supplies: incrementing values in supplies and // decrementing funds
//What to do in this function: First print out the amount of gold the crew has. // Then print each supply type and the amount it costs. You may use a cost array to shorten // this process: int supplycosts[NUMSUPPLIES] = {1, 2, 20, 10}; // Based on the user's selection, ask the user how many of that supply they would like to buy. // Verify that the user has enough gold for their purchase and update the correct index of supplies. // Deduct the corresponding amount from the user's funds. void getsupplies(char supplytypes[NUMSUPPLIES][STRLENGTH], int supplies[NUMSUPPLIES], int *funds) {
}
//Pre-conditions: crew names is an array of strings for the crew members // crewstatus is an array of numerical indicators for the status of each crew member // 0 - deceased, 1 - ill, 2 - healthy // supplies is an array of integers representing how many of each type the crew has // funds represents how many gold pieces the crew has to spend // traveled represents the total number of miles the ship has traveled from the beginning //Post-conditions: none
//What to do in this function: Print a daily status report by telling the user how many miles have been // traveled. Then, print the status of the crew by calling printstatus. Print the funds and amount // of food that the ship has. Then, let the user know how far they are from their next destination. void dailyreport(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int supplies[NUMSUPPLIES], int funds, int traveled) {
}
//Pre-conditions: a and b are both integers //Post-conditions: the larger value will be returned int max(int a, int b) {
}
//Pre-conditions: a and b are both integers //Post-conditions: the smaller value will be returned int min(int a, int b) {
}
//Pre-conditions: supplies is an array of integers representing how many of each type the crew has // crew names is an array of strings for the crew members // crewstatus is an array of numerical indicators for the status of each crew member // 0 - deceased, 1 - ill, 2 - healthy // days represents the current day //Post-conditions: the user will select a number of days to rest for. update days to reflect this number // there is a small chance an ill crew member will recover during rest days
//What to do in this function: ask the user how many days they would like to rest for. // Updates days to indicate that that many days has now passed. Deduct 2 pounds of food // for each crew member for each day rested.
// Generate a pseudorandom value that will be either 0 or 1. Generate a second value // between 0 and the number of original crew members. If the first number is a 1, // the crew member in the index of the second number may recover if they are sick. // If they are healthy or deceased, nothing happens. If the first number is a 0, // nothing happens. void rest(int supplies[NUMSUPPLIES], char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int *days) {
}
//Pre-conditions: none //Post-conditions: returns the number of pounds of fish gained by the ship
//What to do in this function: Generate a pseudorandom value between 0 and 3, inclusive. // Multiply this number by 50 and tell the user how many fish were caught. Return // this value. int fish() {
}
//Pre-conditions: crew names is an array of strings for the crew members // crewstatus is an array of numerical indicators for the status of each crew member // 0 - deceased, 1 - ill, 2 - healthy // days represents the current day // supplies is an array of integers representing how many of each type the crew has //Post-conditions: the status of a crew member or supplies may be affected by a random event, // some events cause the ship to be delayed a certain number of days
//What to do in this function: Generate a pseudorandom number between 0 and 9, inclusive. If the user // has run out of food, tell the user they have no food and increase your number by 2. This number cannot // exceed 9.
// Numbers 0, 1, and 2 do not correspond with any events. // Numbers 3 and 4 correspond with "positive events" // on a 3 the user will gain between 1 and 4 ship parts determined randomly // on a 4 the user will gain between 10 and 60 pounds of food determined randomly // both events take 1 day // Number 5 means the user will lose between 5 and 55 pounds of food // this event takes 1 day // Number 6 means the ship is stuck in fog, which takes 1 day // Number 7 means the ship is in a storm, which takes 2 days // Number 8 means something on the ship has broken // check the number of extra ship parts the crew has // if they have at least one ship part, they can replace it and continue // this takes 1 day // if they have no extra parts, they must stop and repair // this takes 3 days // Number 9 indicates sickness. Generate a random number between 0 and the original // number of crew members. Check the status of that crew member: if they are healthy, // they now fall ill. If they are already ill, they now perish. If they are already // deceased, nothing happens.
// For any days spent in this way, deduct 2 pounds of food per crew member per day. void event(char crewnames[NUMCREW][STRLENGTH], int crewstatus[NUMCREW], int *days, int supplies[NUMSUPPLIES]) {
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
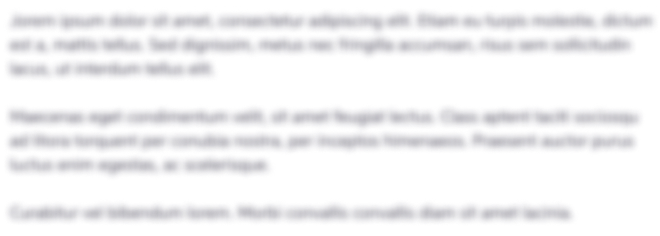
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started