Question
Using the following Java program, modify the code so that whenever you input a number, the number goes into a TextArea (named stuff in the
Using the following Java program, modify the code so that whenever you input a number, the number goes into a TextArea (named stuff in the program). All functions that include increase, decrease, min, max, and insert must all keep the inputted numbers within the TextArea.
import java.io.*;//imports everything within io import java.util.*;//imports everything within util import java.awt.*;//imports everything within awt import java.applet.Applet; import javax.swing.*;//imports everything from swing import java.awt.event.*;//imports everything from event import java.awt.Graphics; import java.awt.Label; import java.awt.List; import java.awt.TextField;
//Key Listener is for registering different keys to different functions! public class Bubble extends Applet implements ActionListener, KeyListener{
Label data; TextArea stuff; TextField enter; Button insert; Button clear; Button inc; Button dec; Button min; Button max; Label output;
//Data int[] a = new int[10]; int[] o= new int[10]; int r = 0;
Boolean start = true; Boolean up = false; Boolean down = false; Boolean MIN = false; Boolean MAX = false;
//NOTE: 200 AND 100 ARE FOR POSITIONING... public void paint(Graphics g){ //There are multiple cases here for each button, so if the Max value button is clicked, the if case featuring MAX is recognized.
if (start){ print(g,"Data items in original order", o , 200, 100); start = false; }
if(up){ print(g,"Data items in Ascending order", a, 200, 100); up = false; }
if(down){ print(g,"Data items in Descending order ", a, 200, 100); down = false;
}
if(MIN){ dec(); printMAXMIN(g,"Minimum Value", a[r-1], 200, 100); MIN = false; }
if(MAX){ dec(); printMAXMIN(g,"Maximum Value", a[0], 200, 100); MAX = false; }
} //For max and min values public void printMAXMIN(Graphics g, String start, int x, int y, int z){
g.drawString(start,y,z); z+=15; g.drawString(String.valueOf(x),y,z); y+=20;
}
//For everything else public void print(Graphics g, String start, int x[], int y, int z){ g.drawString(start,y,z); z+=15; for(int i = 0; i < r; i++){ g.drawString(String.valueOf(x[i]), y, z); y = y + 10 + (String.valueOf(x[i]).length() * 10); if (i % 15 == 0 && i != 0){ z+=20; y=300; } }
}
//This is where I include all of my styling. public void init(){
JPanel main = new JPanel(); main.setLayout(new BorderLayout());
//here I give a set a background color for the JPanel called top. JPanel top = new JPanel(); top.setBackground(Color.GREEN); data = new Label("Please enter some data: "); top.add(data);
//Later on in the program there is an error message that pops up if the user enters something in the area of 4 digits. I allowed space for 4 digits so that this error message can pop up! enter = new TextField(4); top.add(enter); enter.addKeyListener(this);
main.add(top, BorderLayout.NORTH);
JPanel mid = new JPanel(); //Creates a BOX alignment along the x-axis mid.setLayout(new BoxLayout(mid, BoxLayout.X_AXIS)); mid.setBackground(Color.WHITE);
insert = new Button("Insert"); mid.add(insert); insert.addActionListener(this);
clear = new Button("Clear"); mid.add(clear); clear.addActionListener(this);
inc = new Button("Increasing"); mid.add(inc); inc.addActionListener(this);
dec = new Button("Decreasing"); mid.add(dec); dec.addActionListener(this);
min = new Button("Min Value"); mid.add(min); min.addActionListener(this);
max = new Button("Max Value"); mid.add(max); max.addActionListener(this);
//Adds EVERYTHING HERE to the layout. main.add(mid);
JPanel bottom = new JPanel(); bottom.setLayout(new BoxLayout(bottom, BoxLayout.X_AXIS)); bottom.setBackground(Color.WHITE); output = new Label("No Errors Yet, Thankfully!"); bottom.add(output);
main.add(bottom, BorderLayout.SOUTH);
add(main);
}
public void insert(){//adds number to array, if it can't it shows an error message. int number = Integer.valueOf(enter.getText()); if (number >= 1000 || number < 0){ JOptionPane.showMessageDialog(null,"Can't use that number!","ERROR", JOptionPane.ERROR_MESSAGE); return; } if(r < a.length){ o[r] = number; a[r] = number; r++; } }
public void inc(){ int i, k; for(int j=1; j < r; j++){ k = a[j]; for(i = (j - 1); (i >= 0) && (a[i] > k); i--){ a[i+1] = a[i]; } a[i+1] = k; } return; }
public void dec(){ int i, k; for(int j=1; j < r; j++){ k = a[j]; for(i = (j - 1); (i >= 0) && (a[i] < k); i--){ a[i+1] = a[i]; } a[i+1] = k; } return; }
//All actions performed happen as below. public void actionPerformed(ActionEvent e){
//If insert, implement insert() if(e.getSource()== insert){
try{ insert(); start = true; repaint(); enter.setText(" "); }
catch(Exception k){ output.setText("Sorry, You must enter an integer!"); enter.setText(""); }
}
//If clear, clear the data... else if(e.getSource()== clear){ o = new int[10]; a = new int[10]; r = 0; repaint(); } //If inc, implement inc(); else if(e.getSource()==inc){ inc(); up = true; repaint(); } //If dec, implement dec(); else if(e.getSource()==dec){ dec(); down = true; repaint(); }
//If min, then MIN is true and you get the minimum value. else if(e.getSource()== min){ MIN = true; repaint();
} //If max, then MAX is true and you get the maximum value. else if(e.getSource()== max){ MAX = true; repaint(); } } public void keyPressed(KeyEvent e){ //Upon pressing Enter you insert the value into the data set. if (e.getKeyCode()==KeyEvent.VK_ENTER){//enter button adds to array
try{
insert(); start = true; repaint(); enter.setText(""); }
catch(Exception k){ output.setText("Sorry, You must enter an integer!"); enter.setText(""); } }
//Upon pressing C you clear the data set else if(e.getKeyCode() == KeyEvent.VK_C) { o = new int[10]; a = new int[10]; r = 0; repaint(); }
//Upon pressing A, you increase value else if(e.getKeyCode()==KeyEvent.VK_A) { inc(); up = true; repaint(); }
//Upon pressing D, you decrease value else if(e.getKeyCode()==KeyEvent.VK_D) { dec(); down = true; repaint(); }
//Upon pressing M, you get a minimum value. else if(e.getKeyCode()==KeyEvent.VK_M) { MIN = true; repaint(); } //Upon pressing N, you get a maximum value. else if(e.getKeyCode()==KeyEvent.VK_N) { MAX = true; repaint(); } }
//Both of these needed for Key Listener. public void keyReleased(KeyEvent arg) { } public void keyTyped(KeyEvent arg0) { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
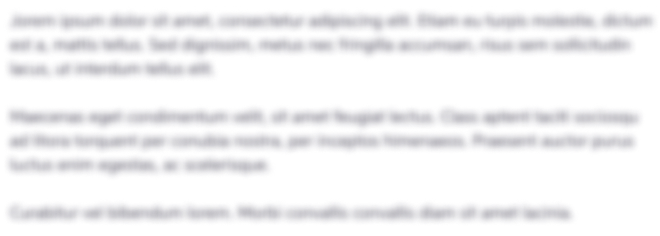
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started