Question
Using the given enumerated types and structures, write the source code (C Language) that will firstly fill the deck with all unique 52 playing cards,
Using the given enumerated types and structures, write the source code (C Language) that will firstly fill the deck with all unique 52 playing cards, and then show the complete deck in the console, one card per line.
Hint: Best practice method is by using a function that will fill the deck, another function to display an individual playing card and a function to display the deck, using that previous function that displays an individual card.
#include
typedef enum CARDSUITE {DIAMONDS, CLUBS, HEARTS, SPADES} CardSuit; typedef enum CARDNUMBER {TWO=2, THREE, FOUR, FIVE, SIX, SEVEN, EIGHT, NINE, TEN, JACK, QUEEN, KING, ACE} CardNumber;
typedef struct PLAYCARD { CardNumber number; CardSuit color; } PlayCard;
#define NR_CARDS_IN_DECK 52
typedef struct DECK { PlayCard card[NR_CARDS_IN_DECK]; } Deck;
void showPlayingCard(PlayCard card); void fillDeck(Deck * p_cardDeck); void showDeck(Deck * p_cardDeck);
//A function to display the given playing card. Both values //from the struct must be displayed. void showPlayingCard(PlayCard card) { char cardColor[10] = " "; char cardValue[10] = " ";
switch (card.color) { case DIAMONDS: strcpy(cardColor, "Diamonds"); break;
case CLUBS: strcpy(cardColor, "Clubs"); break;
case HEARTS: strcpy(cardColor, "Hearts"); break;
case SPADES: strcpy(cardColor, "Spades"); break; }
switch (card.number) { case TWO: strcpy(cardValue, " 2"); break; case THREE: strcpy(cardValue, " 3"); break; case FOUR: strcpy(cardValue, " 4"); break; case FIVE: strcpy(cardValue, " 5"); break; case SIX: strcpy(cardValue, " 6"); break; case SEVEN: strcpy(cardValue, " 7"); break; case EIGHT: strcpy(cardValue, " 8"); break; case NINE: strcpy(cardValue, " 9"); break; case TEN: strcpy(cardValue, "10"); break; case JACK: strcpy(cardValue, "JACK"); break; case QUEEN: strcpy(cardValue, "QUEEN"); break; case KING: strcpy(cardValue, "KING"); break; case ACE: strcpy(cardValue, "ACE"); break; }
printf("The selected card has color : %s ", cardColor); printf("It's value is : %s ", cardValue); }
void fillDeck(Deck * p_cardDeck) {
/* Needs to be created */ /*A function to fill the individual play cards in a deck.*/
}
void showDeck(Deck * p_cardDeck) {
/* Needs to be created */ /*A function to display the cards in a deck.*/
}
int main() { /* seed the pseudo-random number generator */ srand(time(NULL));
/* declare the deck of cards */ Deck cardDeck;
/* fill the deck of cards with unique cards */ fillDeck(&cardDeck);
/* show the deck of cards */ showDeck(&cardDeck);
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
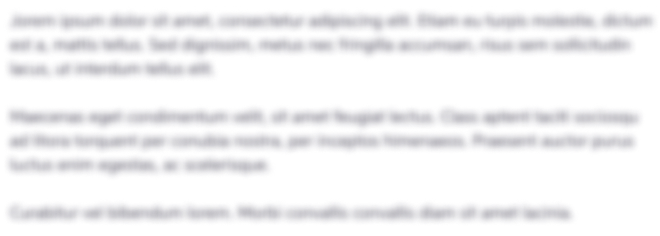
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started