Question
Using the hashtbale code provided below create a program that makes a registry for student records. Create a class that keeps students info (name, student
Using the hashtbale code provided below create a program that makes a registry for student records. Create a class that keeps students info (name, student ID, and grade). If must do the following.
It must be a persistent record, so the registry should be saved on file when exiting, and after any major changes.
It should give an option to make an new entry with the students name, ID, and grade.
It should give an option to lookup a student from their ID (this will ke the key in the hash table).
It should give an option to remove an entry by providing the student ID.
Lastly an option to print the whole registry sorted by ID.
HashTable.java
public interface HashTable
public void add(K key, V value);
public V remove(K key);
public V lookup(K key);
public Object[] getValuesList(); public V[] getSortedList(V[] list); public void printReport();
}
Hashtbl.java
import java.util.*;
public class Hashtbl
ArrayList
public Hashtbl() { for (int i = 0; i < numBuckets; i++) { bucket.add(null); } }
public int getSize() { return size; }
public boolean isEmpty() { return size == 0; }
public int additiveHashing(char[] key, int numBuckets) { int hash = 0; for (char c : key) { hash += c; } return hash % numBuckets; }
public int xorHashing(char[] key, int numBuckets) { int hash = 0; for (char c : key) { hash ^= c; } return hash % numBuckets;
} public int xorShiftHashing(char[] key, int numBuckets){ int hash = 0; for(char c : key){ hash += (c << 3) ^ (c >> 5) ^ hash; } hash = Math.abs(hash); return hash % numBuckets; }
public void add(K key, V value) { String a = key.toString(); char[] b = a.toCharArray(); //int index = additiveHashing(b, numBuckets); //int index = xorHashing(b, numBuckets); int index = xorShiftHashing(b, numBuckets); HashNode
if (head == null) { bucket.set(index, toAdd); size++; } else { while (head != null) { if (head.key.equals(key)) { head.value = value; // size++; No need to increase the size, as the key is already present in the table break; } head = head.next; } if (head == null) { head = bucket.get(index); toAdd.next = head; bucket.set(index, toAdd); size++; } } // Resizing logic if ((1.0 * size) / numBuckets > 0.7) { // do something ArrayList
public V remove(K key) { String a = key.toString(); char[] b = a.toCharArray(); //int index = additiveHashing(b, numBuckets); //int index = xorHashing(b, numBuckets); int index = xorShiftHashing(b, numBuckets); HashNode
if (head.key.equals(key)) { prev.next = head.next; size--; return head.value; } prev = head; head = head.next; } size--; return null; } }
public V lookup(K key) { String a = key.toString(); char[] b = a.toCharArray(); //int index = additiveHashing(b, numBuckets); //int index = xorHashing(b, numBuckets); int index = xorShiftHashing(b, numBuckets); HashNode public static void main(String[] args) { Hashtbl @Override public Object[] getValuesList() { // we have got total size elements Object result[] = new Object[size]; int count=0; for (int index = 0; index < numBuckets; index++) { HashNode return result; } @Override public V[] getSortedList(V[] list) { Arrays.sort(list); return list; } @Override public void printReport() { int usedBuckets = 0; int longestBucket = 0; int totalBucketLen = 0; System.out.println(" +++++++++++++++++ Printing HashTable ++++++++++++ "); for (int index = 0; index < numBuckets; index++) { System.out.print("Index " + index + ": "); HashNode HashNode.java class HashNode public HashNode(K key, V value) { this.key = key; this.value = value; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
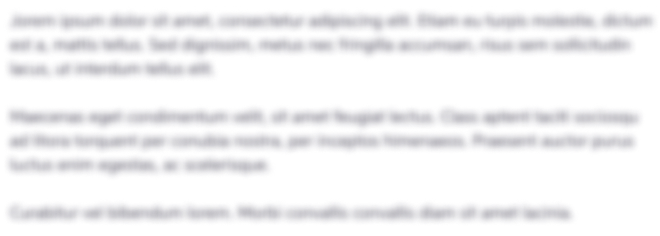
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started