Question
Using the header.h, input.dat, and main.c files below, answer the following questions. Question 1: This program currently uses a library call to get the length
Using the header.h, input.dat, and main.c files below, answer the following questions.
Question 1: This program currently uses a library call to get the length of a string. For example: len = (int) strlen( (const char *)lineBuffer); Replace this call with your own function that is equivalent. The protoype is: int myStrlen( (const char *) stringPtr); Add this function at the end of this file. Replace the call to strlen() with calls to myStrlen(). Question 2: What gets displayed by this program if we changed line3 of input.dat to have just one number (300) ? Describe what happens and explain what you see.
******************************************************************
// header.h //
#ifndef __header_h
#define __header_h
#include
#include
#include
#include
#include
#define NOERROR 0
#define ERROR -1
#define CHAR_ERROR 0xFF
#define MAX_FILENAME_SIZE 128
#define MAX_LINE_SIZE 1024
#endif
*************************************************************************************
Input.dat
1 2 3 4
5 6 7 8
100 200
300 400
This is a line of text
-1
Final Line....
********************************************************************************
// Program : main.c
// Explanation: Shows illustrative examples of fileIO
// Program Parameters: fileName : The name of the file to read
#include "header.h"
#include
int testScanf(char *fileName);
int main(int argc, char *argv[])
{
int rc = NOERROR;
int opCode = 0;
//holds file names in string format. /Init to default file names, program arguments overide defaults
char fileName[MAX_FILENAME_SIZE] = "input.dat";
//The program can support 0, 1, or 2 arguments...Check if the first arg is provided
if ( argc != 2)
{
printf("[%s] Need a fileName ",argv[0]);
rc = ERROR;
return rc;
}
sscanf(argv[1], "%s", fileName);
printf("[%s] Entered with %i arguments, fileName:%s ", argv[0],argc,fileName);
rc = testScanf(fileName);
printf ("[%s] testScanf returns: %i ",argv[0],rc);
if (rc >=0)
rc = NOERROR;
return rc;
}
// routine: int testScanf (char *fileName)
// explanation: reads fileName and illustrates the use of fscanf and sscanf
// inputs: char *fileName: string holding the filename
// outputs: returns an ERROR or NOERROR.
int testScanf (char *fileName)
{
int rc = NOERROR;
FILE *inFile = NULL;
char lineBuffer[MAX_LINE_SIZE];
char *tmpPtr = NULL;
int number1 = 0;
int number2 = 0;
int len = 0;
int lineCount= 0;
int charCount=0;
inFile = fopen(fileName, "r");
if (inFile == NULL) {
fprintf(stderr, "ERROR: Can not open %s ", fileName);
rc = ERROR;
return rc;
}
printf("testScanf: file:%s, rc:%i, read the first number found..... ", fileName, rc);
// Let's get the first line, place it in the lineBuffer
tmpPtr = fgets(lineBuffer, MAX_LINE_SIZE, inFile);
if (tmpPtr == NULL) {
fprintf(stderr," Error from fgets : errno:%i ",errno);
rc = ERROR;
return rc;
}
len = (int) strlen( (const char *)lineBuffer);
charCount+=len;
lineCount++;
printf("line1(%i): %s ",len,lineBuffer);
tmpPtr = fgets(lineBuffer, MAX_LINE_SIZE, inFile);
len = (int) strlen( (const char *)lineBuffer);
charCount+=len;
lineCount++;
printf("line2(%i): %s ",len,lineBuffer);
//Now, read in the first number and the second from the third line
rc = fscanf(inFile, "%d %d", &number1, &number2);
if (rc == EOF) {
fprintf(stderr," Error from fscanf : errno:%i ",errno);
rc = ERROR;
return rc;
}
if (rc != 2) {
fprintf(stderr," WARNING: did not read %i varaiables ?? ",rc);
}
printf(" number of variables read from fscanf:%i, \tnumber1:%i \tnumber2:%i ",rc, number1, number2);
//Next, read the next line
tmpPtr = fgets(lineBuffer, MAX_LINE_SIZE, inFile);
if (tmpPtr == NULL) {
fprintf(stderr," Error from fgets : errno:%i ",errno);
rc = ERROR;
return rc;
}
// fgets returns a line of size 1. In fact, it is the newline. fscanf (and scanf) advances the stream only
// until it is able to get all the variables. newline allows fscanf to complete number2 but keeps the
// newline in the stream.Which is then read on our fgets....So really....this is still reading line 3
len = (int) strlen( (const char *)lineBuffer);
charCount+=len;
lineCount++;
printf("line3(%i): %s ",len,lineBuffer);
// To accommodate, we can check if all we read was a newline
if (len > 0) {
if ( *lineBuffer == ' ')
{
tmpPtr = fgets(lineBuffer, MAX_LINE_SIZE, inFile);
len = (int) strlen( (const char *)lineBuffer);
charCount+=len;
lineCount++;
printf("next line(%i): %s ",len,lineBuffer);
}
}
//THe fourth line is in lineBuffer- if this is input.dat it holds: two numbers: 300 400
//fscanf is used when the input stream is from a FILE.
//scanf is used when the input stream is stdin (we used this in our calculator programs)
//sscanf is used when the input comes from a string (i.e, string scanf )
// NOTE: scanf family functions do the same thing : convert character digits from the selected
// input stream into numbers.
rc = sscanf(lineBuffer, "%d %d", &number1, &number2);
if (rc == EOF) {
fprintf(stderr," Error from fscanf : errno:%i ",errno);
rc = ERROR;
return rc;
}
if (rc != 2) {
fprintf(stderr," WARNING: did not read %i varaiables ?? ",rc);
}
printf(" number of variables read from scanf:%i, \tnumber1:%i \tnumber2:%i ",rc, number1, number2);
printf("testScanf: exit with lineCount:%i, total number characters: %i ", lineCount, charCount);
if (inFile != NULL)
fclose (inFile);
return rc;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
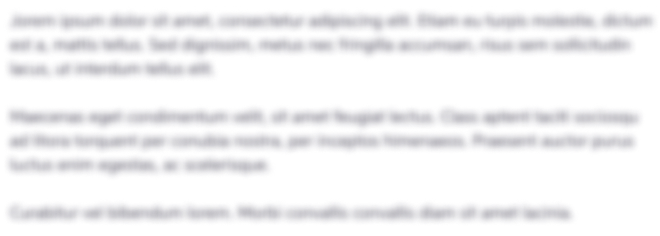
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started