Question
Using the information above your program must do the following: Display a welcome message. Prompt the user for their cats weight in pounds (convert weight
Using the information above your program must do the following:
Display a welcome message.
Prompt the user for their cats weight in pounds (convert weight from pounds to kilogram by dividing by 2.205).
Prompt the user for the number of chocolate pieces their cat ate. Compute the weight of chocolate ingested by the pet. Assume that each chocolate piece is 6 grams in weight. (This will be useful later on.)
Calculate the amount of theobromine and caffeine (in mg) in the chocolate ingested if the chocolate type is dark chocolate. Dark chocolate contains 4.59 mg theobromine and 0.705 mg caffeine, for every gram of chocolate.
Use a named constant to represent amount of theobromine in each gram of dark chocolate. For example:
public static double THEO_IN_DARK_CHOC = 4.59; //mg per g of choc
Multiply this named constant with the actual amount of chocolate ingested (from previous step), to get amount of theobromine ingested by cat.
For example: double theoIfDark = THEO_IN_DARK_CHOC * chocWeight; //mg per g of choc
Use a named constant to represent amount of caffeine in each gram of milk chocolate.
For example: public static double THEO_IN_MILK_CHOC = 2.05; //mg per g of choc
Multiply this named constant with the actual amount of chocolate ingested, to get amount of caffeine ingested by cat.
For example: double caffIfDark = CAFF_IN_DARK_CHOC * chocWeight; //mg per g of choc
Calculate the total toxin dose in mg/kg. We do this by dividing the sum of amounts of theobromine and caffeine ingested by cat (calculated in previous step) by the weight of the cat (in kg).
For example: double toxinDoseIfDark = (theoIfDark + caffIfDark) / wtKgs; //mg per kg of pet weight
Repeat the previous two steps to find total toxin dose in case the chocolate is a milk chocolate. Milk chocolate typically contains 2.05 mg theobromine and 0.21 mg caffeine, for every gram of chocolate.
Display the total toxin values in the amount of chocolate eaten by cat, for both cases if the chocolate is dark chocolate, and if it is milk chocolate.
If the total toxin dose is greater than 55 mg for either of the two types of chocolate, display that the cat has ingested a lethal quantity.
If the total toxin dose is 15 mg or less for both types of chocolate, display that the cat will be okay.
In step 3 we assumed that each piece of chocolate is 6 grams or 0.21 oz. Compute and display to user how much chocolate is unaccounted for, in a 4.4 oz or 125 gram bar of chocolate. (Hint: 125/6 = 20, and 125 % 6 = 5, therefore, there are 20 pieces of 6g weight each, and 5g of chocolate is left unaccounted.)
Do not hard code the values 20 and 5. Let the code do the division and modulo operations.
Ask user for weight of chocolate (in grams) and weight of each piece (in grams). Then compute and display how many pieces of chocolate there are in the bar, and how much chocolate is used up in connecting the pieces (the unaccounted amount).
Display a thank you message for using the program: Thank you for using (your name)s Cat Chocolate Toxicity Calculator!
Make sure your program uses the correct data types:
Use int data types for the number of chocolate pieces.
Use double data types for the amount of theobromine and caffeine amounts and doses.
Use named constants for the occurrence rate of theobromine and caffeine in dark chocolate, and separate named constants for milk chocolate.
Use int data types for weight of chocolate (in grams) and weight of each piece (in grams), when computing number of pieces in chocolate bar, and amount left over.
Example Input and Output
When you test your program the output should look like the following examples, except that your name should be used in place of mine.
Example Run 1:
Welcome to Kriti Chauhan's Cat Chocolate Toxicity Calculator
Please enter your cat's weight in pounds
10
How many pieces of chocolate did your cat eat?
3
Toxin dose for your cat, for each chocolate type is:
dark chocolate: 21.02 mg/kg
milk chocolate: 8.97 mg/kg
In a 125g bar of chocolate, there are 20 pieces, with 5g of chocolate left over.
Enter weight of chocolate bar (in g):
43
Enter weight of each piece of chocolate (in g):
5
In a 43g bar of chocolate, there are 8 pieces, with 3g of chocolate left over.
Thank you for using Kriti Chauhan's cat chocolate toxicity calculator
------------------------------------------------------------------------
Note that in the above example that 10, 3, 43, and 5 are user input and the rest of the information is output by the program.
Example Run 2:
Welcome to Kriti Chauhan's Cat Chocolate Toxicity Calculator
Please enter your cat's weight in pounds
10
How many pieces of chocolate did your cat eat?
8
Toxin dose for your cat, for each chocolate type is:
dark chocolate: 56.04 mg/kg
milk chocolate: 23.92 mg/kg
CAUTION!! Your cat may have ingested a lethal amount of chocolate!
In a 125g bar of chocolate, there are 20 pieces, with 5g of chocolate left over.
Enter weight of chocolate bar (in g):
100
Enter weight of each piece of chocolate (in g):
7
In a 100g bar of chocolate, there are 14 pieces, with 2g of chocolate left over.
Thank you for using Kriti Chauhan's cat chocolate toxicity calculator
------------------------------------------------------------------------
In this example the user input was 10, 8, 100, and 7. Notice that this changes the resulting output. There is a CAUTION statement that wasnt there in the previous output.
Example Run 3:
Welcome to Kriti Chauhan's Cat Chocolate Toxicity Calculator
Please enter your cat's weight in pounds
10
How many pieces of chocolate did your cat eat?
2
Toxin dose for your cat, for each chocolate type is:
dark chocolate: 14.01 mg/kg
milk chocolate: 5.98 mg/kg
Your cat will be okay!
In a 125g bar of chocolate, there are 20 pieces, with 5g of chocolate left over.
Enter weight of chocolate bar (in g):
50
Enter weight of each piece of chocolate (in g):
5
In a 50g bar of chocolate, there are 10 pieces, with 0g of chocolate left over.
Thank you for using Kriti Chauhan's cat chocolate toxicity calculator
------------------------------------------------------------------------
In this example the user input was 10, 2, 50, and 5. Notice that this changes the resulting output. The statement Your cate will be okay! appears in the output.
Notes and Comments
Upload your Java source file to the dropbox named Assignment 2. The name of the source file must be your last name followed by Assign2 with the extension .java. For example, mine would be ChauhanAssign2.java.
Make sure to include comments with your name, a description of what the program does, the course (CSCI 1010), and the assignment name (Assignment 2) at the beginning of your source file.
Make sure you only hand in the source file for your assignment, not the class file, or any other files that NetBeans creates.
Your programs must compile without errors in order to be graded. Once your program compiles make sure to test it using at least two different test cases to see if it meets the requirements of the assignment.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
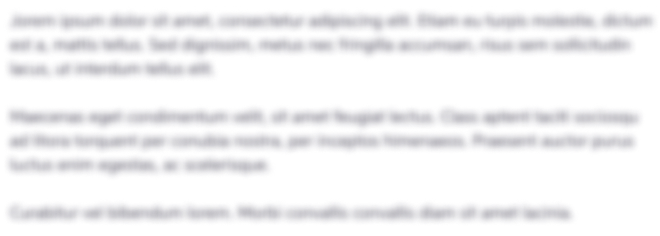
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started