Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using the java code provided below: public class Cashier { public static final double FIVER_VALUE = 5.0; public static final double TOONIE_VALUE = 2.0; public
Using the java code provided below:
public class Cashier { public static final double FIVER_VALUE = 5.0; public static final double TOONIE_VALUE = 2.0; public static final double LOONIE_VALUE = 1.0; public static final double QUARTER_VALUE = 0.25; public static final double DIME_VALUE = 0.1; public static final double NICKEL_VALUE = 0.05; public static final double PENNY_VALUE = 0.01; int fivers,loonies, toonies, quarters, dimes, nickels, pennies; double cashInside, cashLimit, purchase; public Cashier(double cashInside, double cashLimit){ // populate cashier with internal cash and a purchase limit // most cashiers have to have a minimum amount of cash in them (predetermined) // cash is measured in dollars for division purposes this.cashInside = cashInside; this.cashLimit = cashLimit; this.fivers = 5; this.loonies = 10; this.toonies = 10; this.quarters = 20; this.dimes = 20; this.nickels = 50; this.pennies = 50; cashInRegister(); } public void cashInRegister(){ System.out.printf("%Use print formatting to properly display the data as shown in expected output% ", this.fivers, this.toonies, this.loonies, this.quarters, this.dimes, this.nickels, this.pennies); } public void purchase(double itemPrice){ // update purchase total } private double totalCash(int fivers, int toonies,int loonies,int quarters,int dimes,int nickels,int pennies){ // create singular cash value from the multiple coin and bill types given return cash; } public void checkout(int fivers, int toonies,int loonies,int quarters,int dimes,int nickels,int pennies){ double cashGiven = // get total cash from coins and bills given double change = // create probable change amount to be returned by comparing cash given to total purchase amount if(/* if there is enough money given for the purchase and enough money in the register*/){ System.out.println("Successfull purchase of items"); // update the cash inside the cashier object if(/*if cash given is more than the total purchase amount*/){ System.out.println("Your change is: " /* add previous change value*/); // calculate exact coin value regarding the total change amount previously calculated } else{ System.out.println("No change given."); } // the purchase has been made and can be reset to 0 } else if(/* if there is not enough in register*/){ System.out.println("Not enough money in register for items"); } else if(/* if there is not enough money given for the total purchase */){ System.out.println("Not enough money from customer for items"); } } private boolean enoughMoney(double totalPurchase, double cashGiven){ // determine if the cash given is enough for the total purchase } private boolean enoughInRegister(double change){ if(/* find the money available in the register after removing the minimum cash needed inside*/ >= /* compare against the change needed to give out*/){ return true; } else{ return false; } } private void giveChange(double change){ // find all possible five dollar bills that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on five dollar bills // use the remainder operation to update the change to the remainder after diving the total change value by the fiver value // find all possible toonies that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on toonies // use the remainder operation to update the change to the remainder after diving the total change value by the toonie value // find all possible loonies that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on loonies // use the remainder operation to update the change to the remainder after diving the total change value by the loonie value // find all possible quarters that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on quarters // use the remainder operation to update the change to the remainder after diving the total change value by the quarter value // find all possible dimes that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on dimes // use the remainder operation to update the change to the remainder after diving the total change value by the dime value // find all possible nickels that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on quarters // use the remainder operation to update the change to the remainder after diving the total change value by the quarter value // find all possible pennies that can be found in the change, HINT: you may need to do some typecasting // update the internal register's count on pennies // use the remainder operation to update the change to the remainder after diving the total change value by the pennies value System.out.printf("% use proper print formatting context to fit returned change values based on the expected output %", fivers, toonies, loonies, quarters, dimes, nickels, pennies); } public static void main(String[] arguments){ Cashier cashier = new Cashier(49.37, 20); cashier.purchase(6.25); cashier.checkout(1, 2, 1, 0, 0, 0, 0); cashier.cashInRegister(); cashier.purchase(6.25); cashier.purchase(6.25); cashier.checkout(1, 2, 1, 0, 0, 0, 0); cashier.cashInRegister(); cashier.purchase(25); cashier.purchase(25); cashier.checkout(60, 0, 0, 0, 0, 0, 0); cashier.cashInRegister(); } }In this task, you are to create a Cashier object and make attempts to purchase different items that invoke different scenarios. The cashier has: 5 fivers, 1 toonies, 101oonies, 20 quarters, 20 dines, 50 nickels, 58 pennies Successfull purchase of items Your change is: 2.75 You exact change is: fivers, l toonies, loonies, 3 quarters, dines, nickels, pernies The cashier has: 5 fivers, 9 toonies, 101oonies, 17 quarters, 20 dines, 5e nickels, 58 pennies ot enough money from customer for item The cashier has: 5 fivers, 9 toonies, 1 loonies, 17 quarters, 20 dines, 5e nickels, 58 pennies ot enough money in register for items The cashier has:5 fivers,9 toonies,10 Loonies, 17 quarters, 20 dines, 50 nickels,58 pennies BUILD SUCCESSFUL (total time:0 seconds) In this task, you are to create a Cashier object and make attempts to purchase different items that invoke different scenarios. The cashier has: 5 fivers, 1 toonies, 101oonies, 20 quarters, 20 dines, 50 nickels, 58 pennies Successfull purchase of items Your change is: 2.75 You exact change is: fivers, l toonies, loonies, 3 quarters, dines, nickels, pernies The cashier has: 5 fivers, 9 toonies, 101oonies, 17 quarters, 20 dines, 5e nickels, 58 pennies ot enough money from customer for item The cashier has: 5 fivers, 9 toonies, 1 loonies, 17 quarters, 20 dines, 5e nickels, 58 pennies ot enough money in register for items The cashier has:5 fivers,9 toonies,10 Loonies, 17 quarters, 20 dines, 50 nickels,58 pennies BUILD SUCCESSFUL (total time:0 seconds)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
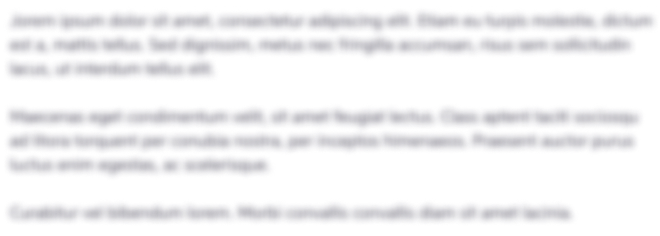
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started