Question
Using the previous Assignment 4, set up your code to use classes. In this Assignment 5, however, all of the class methods can be included
Using the previous Assignment 4, set up your code to use classes.
In this Assignment 5, however, all of the class methods can be included as a part of the main program - without importing any external file.(Similar to Assignment 3 in having all the code in a single program, but with the new data, date calculations, and report layout from Assignment 4).All of the Python code for calculations which had been in the external functions-module will then be changed to become methods in the classes specified, in the single program that you will submit.
Set up the stock data variables as class attributes and functions as stock class methods.
Create a class for the investor as well, and add an address and phone number component for them.
Create an additional class for bonds. Bonds are similar to stocks, so set up the bond class to inherit from the stock class, but add coupon and yield attributes to it as well. Add the method necessary to read the additional two values.
Add ID fields in all the classes - for example investor_ID for the investor class and purchase_ID for the stock and bond purchase records.It is OK to use the capital letters for ID in the variable names in this special case
Instantiate all the classes to the current information Bob Smith has.
Add a single bond to Bob Smith's portfolio, with the following information:
Symbol: GT2:GOV
Purchase Price: 100.02
Current Price: 100.05
Quantity: 200
Coupon: 1.38
Yield: 1.35%
Purchase Date: 8/1/2017
Create a screen print out (report), just like you did before - in Assignment 3, but also add a bond section.Copy a screen print of this report-output to a Word document (with any terse explanations or notes as needed).
Submit the workingPython fileto Canvas - name the file as follows: LastName.FirstName.Assignment-5.pyand also submit aWord document with the report screen print- naming that file as follows: LastName.FirstName.Assignment-screen-5.doc
My assignment -4 code is
Description: This program will show the Stock Earning Summary report for multiple Stocks for Mr. Bob Smith
using nested dictionary data structure and function. It has below fields.
1.Stock symbols for all the stocks Mr. Bob owns
2.Total number of shares for each company Mr. Bob owns.
3.Based on the provided information the program will calculate the amount of money gained/lost by Mr. Bob Smith.
4.Adding new column yearly earning/loss percentage rate for all the stocks.
5.Also adding new stocks to the dictionary.
'''
# Creating 8 Dictnories of Indvidual company Stocks.Each dictnoary holds number of shares, purchase prices
# current value and purchase date of stocks for Mr. Bob Smith.
investor_stock_details = {
'GOOGL' :{
'number_of_shares' :125,
'purchase_price':772.88,
'current_value':941.53,
'purchase_date':'08/01/2015'},
'MSFT' :{
'number_of_shares' :85,
'purchase_price':56.60,
'current_value':73.04,
'purchase_date':'08/01/2015'},
'RDS-A':{
'number_of_shares' :400,
'purchase_price':49.58,
'current_value':55.74,
'purchase_date':'08/01/2015'},
'AIG' :{
'number_of_shares' :235,
'purchase_price':54.21,
'current_value':65.27,
'purchase_date':'08/01/2015'},
'FB':{
'number_of_shares' :150,
'purchase_price':124.31,
'current_value':172.45,
'purchase_date':'08/01/2015'},
'M':{
'number_of_shares' :425,
'purchase_price':30.301,
'current_value':23.98,
'purchase_date':'01/10/2017'},
'F':{
'number_of_shares' :85,
'purchase_price':12.58,
'current_value':10.95,
'purchase_date':'02/17/2017'},
'IBM':{
'number_of_shares' :80,
'purchase_price':150.37,
'current_value':145.30,
'purchase_date':'05/12/2017'}}
''' Output Report '''
#FUNCTION#3 (yearly percentage earning/loss rate for the stocks)
# moving the code to achive same result using function
# Stock Ownership Report title with table column headings,
# including current earnings/loss for stocks
# calulating the yearly percentage earning/loss rate for the stocks
import datetime
def calculate_yearly_rate_earnings(stock_current_value,stock_purchase_price,stock_purchase_date):
#function return Yearly % earning/loss
return ((((stock_purchase_price - stock_current_value) / stock_purchase_price)/(datetime.datetime.now().date() - datetime.datetime.strptime(stock_purchase_date, '%m/%d/%Y').date()).days)/365) * 100
# Years owned for first 5 stocks till date is total days owned/365 i.e.(1730/365) i.e 4.74
# Rest of 3 stocks have different purchase dates so function will calculate number of days owned dynamically
# Printing headers
print('Stock Ownership for Bob Smith')
print('---------------------------------------- ')
print('STOCKSHARE#EARNINGS/LOSSYEARLY % EARNING/LOSS')
print('-----------------------------------------------------------------------------')
# For loop to output the data on each company to the report's detail lines
for company in investor_stock_details:
#passing dictonary values to function to calculate Yearly % earning/loss
result=calculate_yearly_rate_earnings(float(investor_stock_details[company]['purchase_price']),float(investor_stock_details[company]['current_value']),investor_stock_details[company]['purchase_date'])
# The stock company name,number of shares
# output total earning/loss as per price change for a stock times the number of shares owned and
# limited the string to 8 places as per report requirement
# Output the string, using spaces for column alignment
# concatenate calculated Yearly % earning/loss(result) and limited the string to 4 places as per report requirement
print(company +'\t\t'+ str(investor_stock_details[company]['number_of_shares']) + \
'\t\t'+'$' +str((investor_stock_details[company]['current_value'] -investor_stock_details[company]['purchase_price']) * investor_stock_details[company]['number_of_shares'])[0:8]+\
'\t\t'+str(result)[0:4] + ' '+ '%')
#FUNCTION#2-calculate percentage yeild/loss
# moving the code and adding function
# Stock Ownership Report title with table column headings,
# including current earnings per stock
#calulating the yearly earning/loss rate for the stocks
def calculate_earnings_percentage(stock_current_value,stock_purchase_price):
#function return% earning/loss
return (((stock_purchase_price - stock_current_value) / stock_purchase_price)* 100)
#print(' Stock Ownership for Bob Smith')
#print('----------------------------- ')
#print('STOCKSHARE#EARNINGS/LOSS %')
#print('-----------------------------------------------')
#For loop to output the data on each company to the report's detail lines
for company in investor_stock_details:
#passing dictonary values to function to calculate % earning/loss
result=str(calculate_earnings_percentage (float(investor_stock_details[company]['purchase_price']),float(investor_stock_details[company]['current_value'])))
# The stock company name,number of shares
# outpu percentage earning/loss as per price change for a stock and limited the string to 5 places as per report requirement
# Output the string, using spaces for column alignment
# print(company + '\t\t' + str(investor_stock_details[company]['number_of_shares']) + \
#'\t\t' + result[0:5]+' %')
#FUNCTION#1 calculate total earnings
# moving the code and adding function
# Stock Ownership Report title with table column headings,
# including current earnings per stock
#calulating the yearly earning/loss rate for the stocks
def calculate_earnings_loss(number_of_shares,stock_purchase_price,stock_current_value):
#function returntotal earning/loss for a stock holding
return (stock_current_value - stock_purchase_price) * number_of_shares
#print(' Stock Ownership for Bob Smith')
#print('----------------------------- ')
#print('STOCKSHARE#EARNINGS/LOSS $')
#print('-----------------------------------------------')
#For loop to output the data on each company to the report's detail lines
for company in investor_stock_details:
#passing dictonary values to function to calculate total earning/loss
result=str(calculate_earnings_loss(int(investor_stock_details[company]['number_of_shares']),float(investor_stock_details[company]['purchase_price']),float(investor_stock_details[company]['current_value'])))
# The stock company name,number of shares
# output total earning/loss as per price change for a stock times number of shares
# limited the result string to 5 places as per report requirement
# Output the string, using spaces for column alignment
#print(company + '\t\t' + str(stocks[company]['number_of_shares']) + \
#'\t\t' +'$'+ result[0:8])
Step by Step Solution
There are 3 Steps involved in it
Step: 1
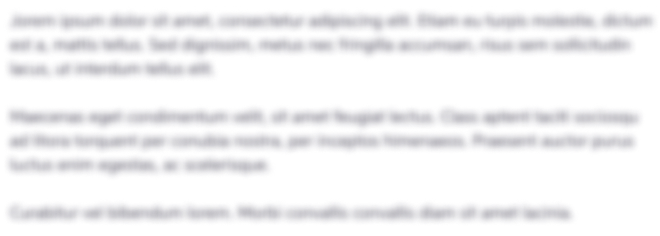
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started