Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Using the provided skeleton code, please write a c program that has the following output / / Small stack implementation using linked list #include #include
Using the provided skeleton code, please write a c program that has the following outputSmall stack implementation using linked list
#include
#include
#define EMPTY assuming we will not have in the stack
Struct used to form a stack of integers.
struct stack
int data;
struct stack next;
;
int pushstruct stack front int num;
int popstruct stack front;
int emptystruct stack front;
int topstruct stack front;
void initstruct stack front;
void printStackstruct stack front;
int main
int choice, data;
struct stack stack; stack is the root of our stack
int tempval;
init; call the init function appropriately
printfMenu: push pop, print exit.
Enter your choice: ;
scanfd &choice;
whilechoice
switchchoice
case :
printfEnter data: ;
scanfd &data;
ifpush&stack data
printfpush failed
;
else
printStackstack;
break; break from case
case :
data pop&stack;
ifdata EMPTY
printfd popped
data;
printStackstack;
else
printfempty stack
;
break;
case :
printStackstack;
printfMenu: push pop, print exit.
Enter your choice: ;
scanfd &choice;
return ;
void initstruct stack front
write the init functin to initialze the front to NULL
void printStackstruct stack front
iffront NULL
printfempty stack";
else
printfstack: ;
write the loop to print the stack. Numbers should be printed in space
separated
while
complete this part
printf
;
Precondition: front points to the top of the stack.
Postcondition: a new node storing num will be pushed on top of the
stack if memory is available. In this case a is
returned. If no memory is found, no push is executed
and a is returned.
int pushstruct stack front int num
complete the push function
Precondition: front points to the top of a stack
Postcondition: Return the top front most data from the stack and
remove the current front and upate the front
return EMPTY if stack is empty.
int popstruct stack front
complete the pop function
Precondition: front points to the top of a stack
Postcondition: returns true if the stack is empty, false otherwise.
int emptystruct stack front
if front NULL
return ;
else
return ;
Precondition: front points to the top of a stack
Postcondition: returns the value stored at the top of the stack if the
stack is nonempty, or otherwise.
int peekstruct stack front
if front NULL
return frontdata;
else
return ;
Example inputoutput the red color texts are part of the input:
Menu: push pop, print exit.
Enter your choice:
Enter data:
stack:
Menu: push pop, print exit.
Enter your choice:
Enter data:
stack:
Menu: push pop, print exit.
Enter your choice:
Enter data:
stack:
Menu: push pop, print exit.
Enter your choice:
popped
stack:
Menu: push pop, print exit.
Enter your choice:
popped
stack:
Menu: push pop, print exit.
Enter your choice:
popped
empty stack
Menu: push pop, print exit.
Enter your choice:
empty stack
Menu: push pop, print exit.
Enter your choice:
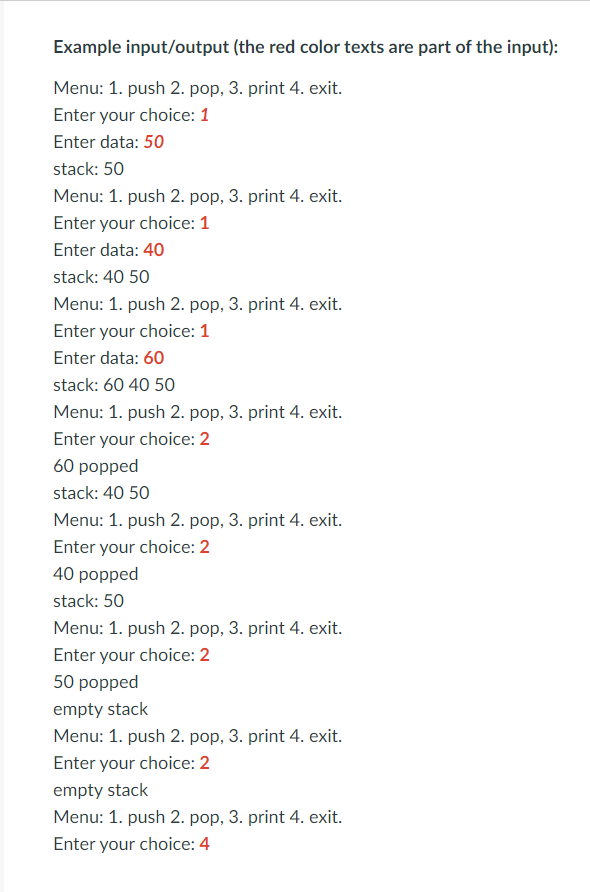
Step by Step Solution
There are 3 Steps involved in it
Step: 1
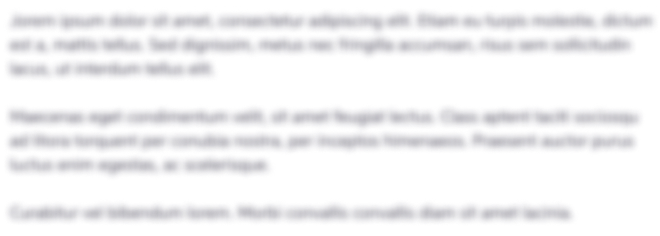
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started