Question
Using your Dog class from earlier this week, complete the following: Create a new class called DogKennel with the following: Instance field(s) - array of
Using your Dog class from earlier this week, complete the following:
Create a new class called DogKennel with the following:
-
Instance field(s) - array of Dogs + any others you may want
-
Contructor - default (no parameters) - will make a DogKennel with no dogs in it
-
Methods:
-
public void addDog(Dog d) - which adds a dog to the array
-
public int currentNumDogs() - returns number of dogs currently in kennel
-
public double averageAge() - which returns the average age of all dogs in the kennel
-
public void adoptDog(String n) - remove the dog with given name
-
Create 1 more method that uses your mutator method from the dog class somehow
Create a main test - either in the DogKennel class file or in its own file
-
Test should create a DogKennel and test each of the methods you have written
Here is the Dog Class:
public class Dog { // Instance FIelds String name, breed; int age;
// Constructors // Default Constructor public Dog() { name = ""; breed = ""; age = 0; }
// Parameterized Constructor public Dog(String n, String b, int a) { name = n; breed = b; age = a; }
// Methods // Accessor Methods // Method to return name of Dog public String getName() { return name; }
// Method to return breed of Dog public String getBreed() { return breed; }
// Method to return age of Dog public int getAge() { return age; }
// Mutator Method // Method to change breed of Dog public void changeBreed(String b) { breed = b; }
// Driver method main public static void main(String args[]) { // Instantiate the class Dog Dog dog1 = new Dog(); // Using Default Constructor Dog dog2 = new Dog("Browney", "German Shepered", 5); // Using Parameterized Constructor
// Print the current status of dogs System.out.println("Dog 1: "); System.out.println("Name: " + dog1.getName() + " Breed: " + dog1.getBreed() + " Age: " + dog1.getAge()); System.out.println("Dog 2: "); System.out.println("Name: " + dog2.getName() + " Breed: " + dog2.getBreed() + " Age: " + dog2.getAge());
// Mutate the breed of dog1 dog1.changeBreed("Pomerian");
// Print the changed dog System.out.println("Dog 1: "); System.out.println("Name: " + dog1.getName() + " Breed: " + dog1.getBreed() + " Age: " + dog1.getAge()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
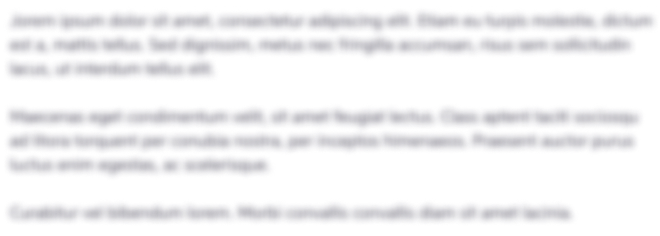
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started