Question
Using your existing Vehicle.java , Car.java , and Truck.java classes. Create a new class called Inventory.java that will keep track of all the cars and
Using your existing Vehicle.java, Car.java, and Truck.java classes. Create a new class called Inventory.java that will keep track of all the cars and trucks inventory. The Inventory class will maintain a list of vehicles that are available for sale. Available operations are as below.
- Constructor
- void add(Vehicle)
- void remove(Vehicle)
- Vehicle findCheapestVehicle()
- Vehicle findMostExpensiveVehicle()
- void printAveragePriceOfAllVehicles()
You can use the List provided by the Java API: List Interface and ArrayList class (implements List) Take a look at the provided methods and decide which ones could be of use to you.
Write JUnit tests to test the Inventory.java class. Follow the guidelines as discussed in class:
- Use AAA (Arrange-Act-Assert) style of testing
- Have good code coverage (test at the minimum every method excluding simple getters and setters, every branches inside a method, test with boundary values, etc)
- Have happy cases (at a minimum), and failure cases when a failure can happen
- You will need to rely on a helper method to test the printAveragePriceOfAllVehicles() method
public class Vehicle { private String make; private String model; private boolean is4WD; private double price; private int mpg; private int year; public Vehicle(String make, String model, boolean is4WD, double price, int mpg, int year) { this.make = make; this.model = model; this.is4WD = is4WD; this.price = price; this.mpg = mpg; this.year = year; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public String getMake() { return make; } public void setMake(String make) { this.make = make; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public boolean isIs4WD() { return is4WD; } public void setIs4WD(boolean is4WD) { this.is4WD = is4WD; } public double getPrice() { return price; } public void setPrice(int price) { this.price = price; } public int getMpg() { return mpg; } public void setMpg(int mpg) { this.mpg = mpg; } public void printVehicle() { System.out.println(year + " " + make + " " + model); if (is4WD) System.out.println("4WD"); else System.out.println("Not 4WD"); System.out.println("$" + price); System.out.println(mpg + "MPG"); }
public class Truck extends Vehicle { private boolean hasSideStep; private int towCapacity; public Truck(String make, String model, int year, boolean is4WD, double price, int mpg, boolean hasSideStep, int towCapacity) { super(make, model, is4WD, price, mpg, year); this.hasSideStep = hasSideStep; this.towCapacity = towCapacity; } public boolean isHasSideStep() { return hasSideStep; } public void setHasSideStep(boolean hasSideStep) { this.hasSideStep = hasSideStep; } public int getTowCapacity() { return towCapacity; } public void setTowCapacity(int towCapacity) { this.towCapacity = towCapacity; } @Override public void printVehicle(){ super.printVehicle(); if(hasSideStep) System.out.println("This Truck has side step."); else System.out.println("This Truck does not have side step."); System.out.println("Tows up to " + towCapacity + " tons"); } }
public class Car extends Vehicle { private boolean isConvertable; public Car(String make, String model, boolean is4WD, double price, int mpg,int year, boolean isConvertable) { super(make, model, is4WD, price, mpg, year); this.isConvertable = isConvertable; } public boolean isConvertable() { return isConvertable; } public void setConvertable(boolean convertable) { isConvertable = convertable; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
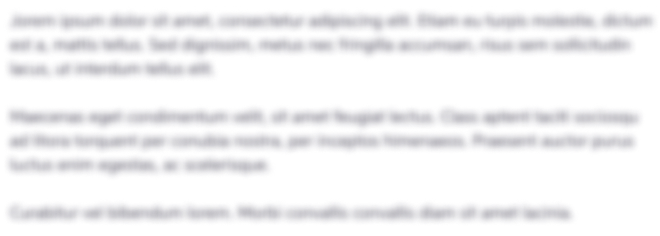
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started