Question
Utilizing the following Java code, I need to make modifications // Contact.java public class Contact { /*basic attributes*/ private String name; private String address; private
Utilizing the following Java code, I need to make modifications
// Contact.java
public class Contact {
/*basic attributes*/
private String name;
private String address;
private String phone;
private String email;
/*getters and setters*/
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
/*returns a string containing all data fields*/
public String toString() {
return name + "\t" + address + "\t" + phone + "\t" + email;
}
}
// ContactBook.java
import java.util.Arrays;
public class ContactBook {
/**
* Array of contacts
*/
Contact[] entries;
/**
* method to add a contact to the list
*/
public void add(Contact c) {
if (entries == null) {
/**
* first entry
*/
entries = new Contact[1];
entries[0] = c;
} else {
/**
* Taking a copy of the array with one size bigger, and assigning to
* the list
*/
entries = Arrays.copyOf(entries, entries.length + 1);
/**
* setting the contact at the current last index
*/
entries[entries.length - 1] = c;
}
System.out.println("Contact added!");
}
public void update(int pos, Contact c) {
if (pos >= 0 && pos < entries.length) {
/**
* valid position, adding contact to the specified index
*/
entries[pos] = c;
System.out.println("Contact updated!");
} else {
System.out.println("Invalid position");
}
}
public void remove(int pos) {
if (pos >= 0 && pos < entries.length) {
/**
* valid position
*/
for (int i = pos; i < entries.length - 1; i++) {
/**
* Shifting elements in the right side to occupy the vacant space
*/
entries[pos] = entries[pos + 1];
}
/**
* Resizing (copying and assigning) the array to have one less length
*/
entries = Arrays.copyOf(entries, entries.length - 1);
System.out.println("Contact updated!");
} else {
System.out.println("Specified index doesn't exist!");
}
}
/**
* returns a String representation of the contact book
*/
public String toString() {
String data = "";
for (int i = 0; i < entries.length; i++) {
data += i + ") " + entries[i].toString() + " ";
}
return data;
}
}
// ContactApp.java
import java.util.Scanner;
public class ContactApp {
static ContactBook contactBook;
static Scanner scanner;
public static void main(String[] args) {
/**
* Initializing the contact book and the scanner for reading input
*/
contactBook = new ContactBook();
scanner = new Scanner(System.in);
int choice = 0;
/**
* Loops until user wish to quit
*/
while (choice != 5) {
/**
* getting the choice
*/
choice = displayMenuAndReturnChoice();
switch (choice) {
case 1: /*Adding a contact*/
Contact c = getContactInput();
contactBook.add(c);
break;
case 2:/*updating a contact in position*/
c = getContactInput();
System.out.println("Enter the position to be updated: ");
int pos = Integer.parseInt(scanner.nextLine());
contactBook.update(pos, c);
break;
case 3:/*Removing a contact*/
System.out
.println("Enter the position of contact to be removed: ");
pos = Integer.parseInt(scanner.nextLine());
contactBook.remove(pos);
break;
case 4:/*Displaying all contacts*/
System.out.println(contactBook);
break;
case 5:
System.out.println("Bye!");
break;
}
}
}
/**
* method to display the menu and returns the choice
*
* @return - a valid choice between 1-5
*/
static int displayMenuAndReturnChoice() {
System.out.println("1. Add a contact");
System.out.println("2. Update a contact");
System.out.println("3. Remove a contact");
System.out.println("4. List all contacts");
System.out.println("5. Exit");
System.out.println("## Enter your choice: ");
try {
int choice = Integer.parseInt(scanner.nextLine());
if (choice >= 1 && choice <= 5) {
return choice;
} else {
System.out.println("Invalid choice, try again");
return displayMenuAndReturnChoice();
}
} catch (Exception e) {
System.out.println("Invalid input, try again...");
return displayMenuAndReturnChoice();
}
}
/**
* method to prompt the user to enter details of a contact, create a Contact
* object and returns it (this helper method can be used by add/update
* operations)
*/
private static Contact getContactInput() {
Contact c = new Contact();
System.out.println("Enter name: ");
String name = scanner.nextLine();
System.out.println("Enter address: ");
String address = scanner.nextLine();
System.out.println("Enter phone: ");
String phone = scanner.nextLine();
System.out.println("Enter email: ");
String email = scanner.nextLine();
c.setName(name);
c.setPhone(phone);
c.setAddress(address);
c.setEmail(email);
return c;
}
}
- Create the ContactFileManager class with two methods a. writeContacts, which takes a String (the filename to write to) and an array of Contact objects as parameters and writes the Contact data to the file with the given name. This method may throw an IOException. b. readContacts, which take a String (the filename to read from) as a parameter, reads an array of Contacts objects from that file, and returns that array. This method may throw an IOException or a ClassNotFoundException.
Modify the existing Contact and ContactBook classes accordingly a. Contact must implement Serializable. b. ContactBook must include the two new methods. i. Add a private instance variable named fileMan which is a ContactFileManager object. ii. Add two public void methods which each take a Scanner object as a parameter a. save, which takes a String as a parameter. The String is the name of the file to save all of the Contact data to. Note that this method should not throw any exceptions. If saving fails this should display Unable to save. as well as the message of the associated exception b. load, which takes a String as a parameter. The String is the name of the file to load all Contact data from (previously loaded entries if any will be lost). Note that this method should not throw any exceptions. If loading fails this should display Unable to load. as well as the message of the associated exception. - Modify the existing ContactApp class to add two new options to the menu. It should now match the sample below and function appropriately, asking the user for a file name whenever they choose to save or load the contact book.
1) List All Contacts 2) Add a Contact 3) Update a Contact 4) Remove a Contact 5) Save Contact Book 6) Load Contact Book 7) Exit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
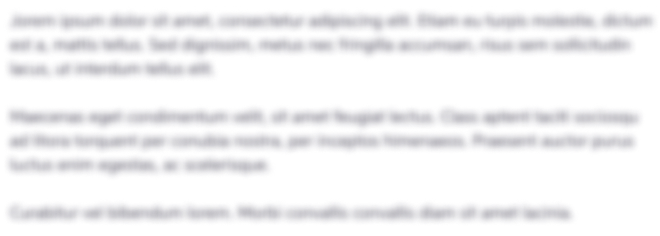
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started