Question
utils Module getInt() Add the following functions to the utils module: int getInt( const char* prompt = nullptr // User entry prompt ); This function
utils Module
getInt()
Add the following functions to the utils module:
int getInt( const char* prompt = nullptr // User entry prompt );
This function performs a fool-proof integer entry from the console.
If the prompt is not null, it is displayed before the entry as a prompt (only once).
If the user enters an invalid integer, the message"Bad integer value, try again: "is displayed until the user enters a valid integer value.
If after a valid integer value any character other than aNew Lineis entered, then the message"Enter only an integer, try again: "is displayed until the user enters only an integer value at the entry.
The entered value is returned at the end.
int getInt( int min, // minimum acceptable value int max, // maximum acceptable value const char* prompt = nullptr, // User entry prompt const char* errorMessage = nullptr, // Invalid value error message bool showRangeAtError = true // display the range if invalud value entered );
This function performs a fool-proof integer entry from the console.
This function works exactly like the previous getInt function with the following additional features.
The range of the valid entry is checked after a valid integer is received.
If the value is out of the range specified by theminandmaxarguments, the function will keep trying to get a proper value until the user enters it correctly.
After each invalid entry, the errorMessage is displayed only if the errorMessage is not null.
After each invalid entry, the range of valid entry is displayed in the following format only if the showRangeAtError argument is true:
"[MM <= value <= XX]"
- MM is replaced with the min argument value
- XX is replaced with the max argument value
getInt unit test
void getIntTester() { cout << "getInt tester:" << endl; cout << "Enter 11: "; cout << getInt() << endl << endl; cout << "Enter the following values at the prompt: " << endl; cout << "abc" << endl; cout << "9abc " << endl; cout << "9 (there is a space after 9)" << endl; cout << "9 " << endl; cout << getInt("> ") << endl << endl; cout << "Enter the following values at the prompt: " << endl; cout << "abc " << endl; cout << "9 " << endl; cout << "10 " << endl; cout << "21 " << endl; cout << "21 (there is a space after 21)" << endl; cout << "20 " << endl; getInt(10, 20, "> ", "Value must be between 10 and 20: ", false); cout << "last value entered: " << getInt(10, 20, "> ", "Invalid value, retry ") << endl; }
getIntTester output
getInt tester: Enter 11: 11 11 Enter the following values at the prompt: abc9abc 9 (there is a space after 9) 9 > abc Bad integer value, try again: 9abc Enter only an integer, try again: 9 Enter only an integer, try again: 9 9 Enter the following values at the prompt: abc 9 10 21 21 (there is a space after 21) 20 > abc Bad integer value, try again: 9 Value must be between 10 and 20: 10 > 21 Invalid value, retry [10 <= value <= 20]: 21 Enter only an integer, try again: 20 last value entered: 20
getcstr()
char* getcstr( const char* prompt = nullptr, // User entry prompt std::istream& istr = std::cin, // the Stream to read from char delimiter = ' ' // Delimiter to mark the end of data );
Prompts the user for the entry if the prompt argument is not null.
Receives an unknown size string from the istream object and allocates a dynamic Cstring to the size of the string and copies the value of the string into it. (Make sure null termination is put into account when setting the size)
In the end, it will return the dynamically allocated memory.
Challenge: Implement this without using the C++stringclass. (Make sure to notify your professor of the implementation in your final project reflection)
getcstr unit test
void getcstrTester() { char* cstr; cout << "Copy and past the following at the prompt:" << endl; cout << "If you didn't care what happened to me, " "And I didn't care for you, " "We would zig zag our way through the boredom and pain, " "Occasionally glancing up through the rain. " "Wondering which of the brothers to blame. " "And watching for pigs on the wing." << endl; cstr = getcstr("Paste here /> "); cout << cstr << endl; delete[] cstr; }
getcstrTester output
Copy and paste the following at the prompt: If you didn't care what happened to me, And I didn't care for you, We would zig zag our way through the boredom and pain, Occasionally glancing up through the rain. Wondering which of the brothers to blame. And watching for pigs on the wing. Paste here /> If you didn't care what happened to me, And I didn't care for you, We would zig zag our way through the boredom and pain, Occasionally glancing up through the rain. Wondering which of the brothers to blame. And watching for pigs on the wing. you entered: If you didn't care what happened to me, And I didn't care for you, We would zig zag our way through the boredom and pain, Occasionally glancing up through the rain. Wondering which of the brothers to blame. And watching for pigs on the wing.
Time Module
The time module is designed to:
- read and write time values.
- measure the passage of time by doing basic arithmetic operations
The time module only holds the time in minutes but will display and read the time in the following format:
HH:MM
For example, when the Time object holds the value 125, it will display02:05. Likewise if the time13:55is read by the Time object from a stream,835is stored in the object (i.e. 13x60+55). Note that since the Time object is also used for the passage of time, there is no limit to the number of hours and minutes and they may pass 24 and 60 if needed.
Note:125:15is a valid time that means125hours and15minutes also0:96is a valid entry and it is displayed as:01:36that is1hour and36minutes
Complete the implementation of the Time class with the following mandatory specs:
namespace sdds { class Time { unsigned int m_minutes; public: Time& setToNow(); Time(unsigned int minutes = 0); std::ostream& write(std::ostream& ostr) const; std::istream& read(std::istream& istr); Time& operator-=(const Time& D); Time operator-(const Time& D)const; Time& operator+=(const Time& D); Time operator+(const Time& D)const; Time& operator=(unsigned int val); Time& operator *= (unsigned int val); Time& operator /= (unsigned int val); Time operator *(unsigned int val)const; Time operator /(unsigned int val)const; operator unsigned int()const; operator int()const; }; Time& setToNow();
setToNow, sets theTimeto the current time usingsdds::getTime()(available inutilsmodule) and then returns the reference of the current object.
Note that if thesdds::debugis set to true, thegetTime()function will receive the time from the user instead. This will be used for debugging purposes and when submitting your work through the submitter program.
Time::Time
Time(unsigned int min = 0);
Constructs the Time by setting the number of minutes held in the object or set the time to zero by default.
Time::write
std::ostream& write(std::ostream& ostr) const;
Writes the time into a stream in HH:MM format padding the spaces with zero if the numbers are single digit (examples 03:02, 16:55 234:06 )
Time::read
std::istream& read(std::istream& istr);
Reads the time from a stream in H:M format. It makes sure that the two integers (hours and minute) are greater than zero and separated by ":", otherwise it will set theistreamobject to afailure state.
This function does not react to any invalid data, instead, it will work exactly how istream works;It will put the istream in a failure state if anything goes wrongby following these steps:
- reads the integer for the hours usingistrand if the value is negative, it setsthe istream object to a failure state.
- reads one character and makes sure it is':'. If it is not':', it will setthe istream object to a failure state.
- reads the integer for the minutes usingistrand if the value is negative, itsets the istream object to a failure state.
setting istream to a fail state
To set the istream to a fail state manually call the following method of istream:
setstate(ios::failbit);
Note: Do not clear or flush theistreamobject since this method complies with theistreamstandards. The caller of this function may check the state of theistreamobject to make sure that the read was successful if needed.
Time basic arithmetic operations
All the implemented basic arithmetic operations onTimeare done exactly as it is defined in math except for the subtraction:
Time::operator-=
Design the subtraction in theTimeas if you are turning a 24-hour clock backwards:
Time& operator-= (const Time& D);
Calculates the time difference between the current time and the incoming argument TimeDand the returns the reference of the left operand object. Note that the difference can never be a negative value:
23:00 -= 9:00 will be14:00.
18:00 -= 16:00 will be2:00.
1:00 -= 22:00 will be3:00. ((1:00+ 24:00) -22:00)
Also:
1:00 -= 46:00 will be3:00. ((1:00+ 24:00 + 24:00) -46:00)
See the illustration below:
Time::operator-
Time operator-(const Time& D)const;
Works exactly like the operator-= but without side-effect. This operator will not modify the left operand and returns aTimeobject that is the result of the calculation.
Time::operator+=
Time& operator+=(const Time& D);
Add the minute value of the right operand to the value of the left operand and then returns the reference of the left operand.
Time::operator+
Time operator+(const Time& D)const;
Creates a Time object with the minute value that is the sum of the minute values of the left and right operands and then returns it.
Time::operator=
Time& operator=(unsigned int val);
Sets the minute value of the left operand to the value of the right operand and then returns the reference of the left operand.
Time::operator*=
Time& operator *= (unsigned int val);
Multiplies the minutes' value of the left operand by the value of the right operand and then returns the reference of the left operand.
Time::operator*
Time operator *(unsigned int val)const;
Creates aTimeobject with the minutes value being the product of the minutes' value of the left operand by the value of the right operand and returns the object.
Time::operator/=
Time& operator /= (unsigned int val);
Divides the minutes' value of the left operand by the value of the right operand and then returns the reference of the left operand.
Time::operator/
Time operator /(unsigned int val)const;
Creates aTimeobject with the minutes value being the division of the minutes' value of the left operand by the value of the right operand and returns the object.
Time::operator int
operator int()const;
When the time is cast to an integer, it will return the number of minutes as an integer.
Time::operator unsigned int
operator unsigned int()const;
When the time is cast to an unsigned integer, it will return the number of minutes.
operator<<
Overload the insertion operator to be able to insert a Time object into an ostream object
operator>>
Overload the extraction operator to be able to extract data from an istream object into the Time object
Time unit test
void timeTester() { Time D(1385u), C(65u), E; cout << "E: " << E << endl; cout << "D: " << D << endl; cout << "C: " << C << endl; cout << " D C D-=C" << endl; cout << D << " -= " << C << " = "; cout << (D -= C) << endl << endl; cout << " C D C-=D" << endl; cout << C << " -= " << D << " = "; cout << (C -= D) << endl << endl; cout << " C D C+=D" << endl; cout << C << " += " << D << " = "; cout << (C += D) << endl << endl; cout << " C = 245u" << endl; cout << "C: " << (C = 245u) << endl << endl; cout << " D = 2760u" << endl; cout << "D: " << (D = 2760u) << endl << endl; cout << " E = C + D" << endl; E = C + D; cout << E << " = " << C << " + " << D << endl << endl; cout << " E = C - D" << endl; E = C - D; cout << E << " = " << C << " - " << D << endl << endl; cout << "C: " << C << endl; cout << " C *= 2u;" << endl << "C: "; cout << (C *= 2u) << endl; cout << " C *= 12u" << endl << "C: "; cout << (C *= 12u) << endl << endl; cout << " C = 245u" << endl; cout << "C: " << (C = 245u) << endl << endl; cout << " E = C * 2u" << endl; E = C * 2u; cout << E << " = " << C << " * 2u" << endl << endl; cout << " E = C * 12u" << endl; E = C * 12u; cout << E << " = " << C << " * 12u" << endl << endl; cout << "C: " << C << endl; cout << " C /= 2u;" << endl << "C: "; cout << (C /= 2u) << endl; cout << " C = 245u" << endl; cout << "C: " << (C = 245u) << endl << endl; cout << " E = C / 2u" << endl; E = C / 2u; cout << E << " = " << C << " / 2u" << endl << endl; cout << "E: " << E << endl; cout << "D: " << D << endl; cout << "C: " << C << endl << endl; cout << "Enter the following values at the prompt:" << endl; cout << "aa:bb" << endl; cout << "12,12 " << endl; cout << "-12:12 " << endl; cout << "12:-12" << endl; cout << "12:12" << endl; cout << "Please enter the time (HH:MM): "; bool done; do { done = true; cin >> E; if (cin.fail()) { cin.clear(); cin.ignore(1000, ' '); done = false; cout << "Bad time entry, retry (HH:MM): "; } } while (!done); cout << "you entered: " << E << endl << endl; cout << "Enter 100:100 at the prompt: " << endl; sdds::debug = true; E.setToNow(); cout << E << endl; sdds::debug = false; cout << "The actual system time is: " << Time().setToNow() << endl; }
Time unit test output
E: 00:00 D: 23:05 C: 01:05 D C D-=C 23:05 -= 01:05 = 22:00 C D C-=D 01:05 -= 22:00 = 03:05 C D C+=D 03:05 += 22:00 = 25:05 C = 245u C: 04:05 D = 2760u D: 46:00 E = C + D 50:05 = 04:05 + 46:00 E = C - D 06:05 = 04:05 - 46:00 C: 04:05 C *= 2u; C: 08:10 C *= 12u C: 98:00 C = 245u C: 04:05 E = C * 2u 08:10 = 04:05 * 2u E = C * 12u 49:00 = 04:05 * 12u C: 04:05 C /= 2u; C: 02:02 C = 245u C: 04:05 E = C / 2u 02:02 = 04:05 / 2u E: 02:02 D: 46:00 C: 04:05 Enter the following values at the prompt: aa:bb12,12 -12:12 12:-12 12:12 Please enter the time (HH:MM): aa:bb Bad time entry, retry (HH:MM): 12,12 Bad time entry, retry (HH:MM): -12:12 Bad time entry, retry (HH:MM): 12:-12 Bad time entry, retry (HH:MM): 12:12 you entered: 12:12 Enter 100:100 at the prompt: Enter current time: 100:100 101:40 The actual system time is: 11:46
Step by Step Solution
There are 3 Steps involved in it
Step: 1
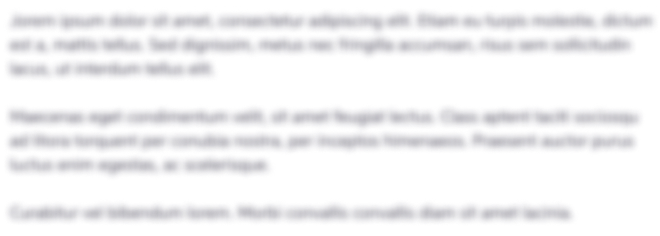
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started