Question
// Vehicle.java as parent class class Vehicle { //data members declared as private private String ownerName; private String address; private String phone; private String email;
// Vehicle.java as parent class class Vehicle { //data members declared as private private String ownerName; private String address; private String phone; private String email; //parameterized constructor of vechicle class public Vehicle(String ownerName, String address, String phone, String email) { this.ownerName = ownerName; this.address= address; this.phone = phone; this.email = email; } //getter and setters for data members public String getOwnerName() { return ownerName; } public void setOwnerName(String ownerName) { this.ownerName = ownerName; } public String getAddress() { return address; } public void setAddress(String address) { this.address = address; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } //toString(0 returns String type contains data member values of a vehicle class public String toString() { return "Owner's name : " + ownerName + " " + "Address : " + address + " " + "Phone : " + phone + " " + "Email : " + email; } } //Car is child class inherits from vechile class Car extends Vehicle { //datamembers intilation as private private boolean convertible; private String color; //parameterized constructor of Car class public Car(String ownerName, String address, String phone, String email, boolean convertible, String color) { //calling Vechicle class constructor using super super(ownerName, address, phone, email); this.convertible = convertible; this.color = color; } //getters and setters for convertible and getcolor data members of car public boolean getConvertible() { return convertible; } public void setConvertible(boolean convertible) { this.convertible = convertible; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } //toString() method returns String type And over rides parent class is Vehicle public String toString() { return super.toString() + " " + "Convertible : " + convertible + " " + "Color : " + color; } }//end car class /*AmericanCar inherits Car and Car inherits vechile .i.e multilevel inhertience between vechile and AmericanCar*/ class AmericanCar extends Car { //Adding two data members private boolean madeDetroit; private boolean unionShop; //parameterized constructor of American car public AmericanCar(String ownerName, String address, String phone, String email, boolean convertible, String color, boolean madeDetroit, boolean unionShop) { //calling car class parameterized constructor super(ownerName, address, phone, email, convertible, color); this.madeDetroit = madeDetroit; this.unionShop = unionShop; } //getter and setters for madeDetroit, unionShop data members public boolean getMadeDetroit() { return madeDetroit; } public void setMadeDetroit(boolean madeDetroit) { this.madeDetroit = madeDetroit; } public boolean getUnionShop() { return unionShop; } public void setUnionShop(boolean unionShop) { this.unionShop = unionShop; } //overriding super class toString() and returns adding with newly added variables public String toString() { return super.toString() + " " + "Made in Detroit : " + madeDetroit + " " + "Union shop : " + unionShop; } } //child class ForeignCar inherits from Car parent class class ForeignCar extends Car { // ForeignCar data members intilization private String manufacturerCountry; private float importDuty; //parameterized constructor for ForeignCar public ForeignCar(String ownerName, String address, String phone, String email, boolean convertible, String color, String manufacturerCountry, float importDuty) { super(ownerName, address, phone, email, convertible, color); this.manufacturerCountry = manufacturerCountry; this.importDuty = importDuty; } //getters and setters for manufacturerCountry, importDuty public String getManufacturerCountry() { return manufacturerCountry; } public void setManufacturerCountry(String manufacturerCountry) { this.manufacturerCountry = manufacturerCountry; } public float getImportDuty() { return importDuty; } public void setImportDuty(float importDuty) { this.importDuty = importDuty; } //toString() overrides parent class car public String toString() { return super.toString() + " " + "Manufacturer country : " + manufacturerCountry + " " + "Import duty : " + importDuty; } } //child class Bicycle inherits from vehicle parent class class Bicycle extends Vehicle { //initialize data member as private private int numberSpeeds; //parameter constructor of Bicycle public Bicycle(String ownerName, String address, String phone, String email, int numberSpeeds) { super(ownerName, address, phone, email); this.numberSpeeds = numberSpeeds; } //getter and setter of numberspeeds data member public int getNumberSpeeds() { return numberSpeeds; } public void setNumberSpeeds(int numberSpeeds) { this.numberSpeeds = numberSpeeds; } //overriding toString method() return String type public String toString() { return super.toString() + " " + "Number of speeds : " + numberSpeeds; } } //child class Truck inherits parent class Vechicle class Truck extends Vehicle { private float numberTons; private float truckCost; private String datePurchased; //parameter constructor of Truck public Truck(String ownerName, String address, String phone, String email, float numberTons, float truckCost, String datePurchased) { super(ownerName, address, phone, email); this.numberTons = numberTons; this.truckCost = truckCost; this.datePurchased = datePurchased; } //getters and setter for Truck data members public float getNumberTons() { return numberTons; } public void setNumberTons(float numberTons) { this.numberTons = numberTons; } public float getTruckCost() { return truckCost; } public void setTruckCost(float truckCost) { this.truckCost = truckCost; } public String getDatePurchased() { return datePurchased; } public void setDatePurchased(String datePurchased) { this.datePurchased = datePurchased; } //toString() override superclass method public String toString() { return super.toString() + " " + "Number of tons : " + numberTons + " " + "Truck cost : " + truckCost + " " + "Date purchased : " + datePurchased; } } // VehicleTest.java import java.io.*; import java.util.*; public class VehicleTest { public static void main(String[] args) throws IOException { //creating arraylist for vechicle class ArrayList vehicles = new ArrayList(); FileInputStream in = new FileInputStream("vehicles.txt"); BufferedReader br = new BufferedReader(new InputStreamReader(in)); String line; String ownerName, address, phone, email, color, manufacturerCountry, datePurchased; boolean convertible, madeDetroit, unionShop; float importDuty, numberTons, truckCost; int numberSpeeds; try { while((line = br.readLine()) != null) { ownerName = br.readLine(); address = br.readLine(); phone = br.readLine(); email = br.readLine(); if (line.equals("vehicle")) { vehicles.add(new Vehicle(ownerName, address, phone, email)); } else if (line.equals("car")) { convertible = Boolean.parseBoolean(br.readLine()); color = br.readLine(); vehicles.add(new Car(ownerName, address, phone, email, convertible, color)); } else if (line.equals("american car")) { convertible = Boolean.parseBoolean(br.readLine()); color = br.readLine(); madeDetroit = Boolean.parseBoolean(br.readLine()); unionShop = Boolean.parseBoolean(br.readLine()); vehicles.add(new AmericanCar(ownerName, address, phone, email, convertible, color, madeDetroit, unionShop)); } else if (line.equals("foreign car")) { convertible = Boolean.parseBoolean(br.readLine()); color = br.readLine(); manufacturerCountry = br.readLine(); importDuty = Float.parseFloat(br.readLine()); vehicles.add(new ForeignCar(ownerName, address, phone, email, convertible, color, manufacturerCountry, importDuty)); } else if (line.equals("bicycle")) { numberSpeeds = Integer.parseInt(br.readLine()); vehicles.add(new Bicycle(ownerName, address, phone, email, numberSpeeds)); } else if (line.equals("truck")) { numberTons = Float.parseFloat(br.readLine()); truckCost = Float.parseFloat(br.readLine()); datePurchased = br.readLine(); vehicles.add(new Truck(ownerName, address, phone, email, numberTons, truckCost, datePurchased)); } br.readLine(); } } catch(Exception e) { System.out.println(e); return; } finally { br.close(); in.close(); } // convert to an array Vehicle[] va = new Vehicle[vehicles.size()]; va = vehicles.toArray(va); br = new BufferedReader(new InputStreamReader(System.in)); while (true) { System.out.println(" Menu"); System.out.println(" ---- "); System.out.println("1. Print all records. "); System.out.println("2. Sort records by email address. "); System.out.println("3. Print number of records. "); System.out.println("4. Print bicycle and truck records. "); System.out.println("5. Print vehicles in area code 987. "); int choice = 0; try { do { System.out.print("Your choice 1-5 or stop to close: "); String input = br.readLine(); if (input.toLowerCase().equals("stop")) return; choice = Integer.parseInt(input); } while(choice < 1 || choice > 5); } catch(Exception e) { System.out.println(e); } System.out.println(); switch(choice) { case 1: printAll(va); break; case 2: sort(va, true); break; case 3: printNumberRecords(va); break; case 4: printBicyclesAndTrucks(va); break; case 5: printAreaCode987(va); break; } } } private static void printAll(Vehicle[] va) { for(int i = 0; i < va.length; i++) { System.out.println("Vehicle type : " + va[i].getClass().getName()); System.out.println(va[i].toString()); System.out.println(); } } private static void sort(Vehicle[] va, boolean print) { Vehicle temp; for(int i = 0;i < va.length - 1;i++) { for(int j = i + 1; j < va.length;j++) { if(va[j].getEmail().compareTo(va[i].getEmail()) < 0) { temp = va[j]; va[j] = va[i]; va[i] = temp; } } } if (print) printAll(va); } private static void printNumberRecords(Vehicle[] va) { System.out.println("The number of records is " + va.length); System.out.println(); } private static void printBicyclesAndTrucks(Vehicle[] va) { sort(va, false); // make sure sorted first for(int i = 0; i < va.length; i++) { String typeName = va[i].getClass().getName(); if (typeName.equals("Bicycle") || typeName.equals("Truck")) { System.out.println("Vehicle type : " + typeName); System.out.println(va[i].toString()); System.out.println(); } } } private static void printAreaCode987(Vehicle[] va) { for(int i = 0; i < va.length; i++) { if (va[i].getPhone().startsWith("(987)", 0)) { System.out.println("Vehicle type : " + va[i].getClass().getName()); System.out.println(va[i].toString()); System.out.println(); } } } }. this code does not compile can someone please help me with that?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
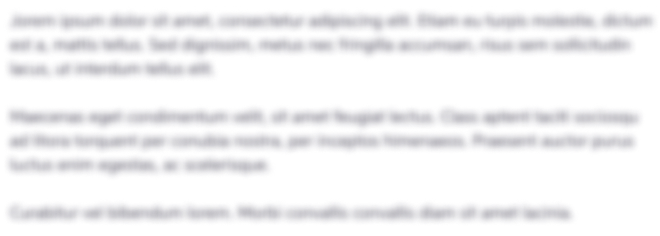
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started