Answered step by step
Verified Expert Solution
Question
1 Approved Answer
very easy linked list Implementation the given code list.cpp #include #include #include using namespace std; class List; class Iterator; class Node { public: /** Constructs
very easy linked list Implementation

the given code list.cpp
#include
#include
#include
using namespace std;
class List;
class Iterator;
class Node
{
public:
/**
Constructs a node with a given data value.
@param s the data to store in this node
*/
Node(string s);
private:
string data;
Node* previous;
Node* next;
friend class List;
friend class Iterator;
};
class List
{
public:
/**
Constructs an empty list;
*/
List();
/**
Appends an element to the list.
@param data the value to append
*/
void push_back(string data);
/**
Inserts an element into the list.
@param iter the position before which to insert
@param s the value to append
*/
void insert(Iterator iter, string s);
/**
Removes an element from the list.
@param iter the position to remove
@return an iterator pointing to the element after the
erased element
*/
Iterator erase(Iterator iter);
/**
Gets the beginning position of the list.
@return an iterator pointing to the beginning of the list
*/
Iterator begin();
/**
Gets the past-the-end position of the list.
@return an iterator pointing past the end of the list
*/
Iterator end();
private:
Node* first;
Node* last;
friend class Iterator;
};
class Iterator
{
public:
/**
Constructs an iterator that does not point into any list.
*/
Iterator();
/**
Looks up the value at a position.
@return the value of the node to which the iterator points
*/
string get() const;
/**
Advances the iterator to the next node.
*/
void next();
/**
Moves the iterator to the previous node.
*/
void previous();
/**
Compares two iterators
@param b the iterator to compare with this iterator
@return true if this iterator and b are equal
*/
bool equals(Iterator b) const;
private:
Node* position;
List* container;
friend class List;
};
Node::Node(string s)
{
data = s;
previous = NULL;
next = NULL;
}
List::List()
{
first = NULL;
last = NULL;
}
void List::push_back(string data)
{
Node* new_node = new Node(data);
if (last == NULL) // List is empty
{
first = new_node;
last = new_node;
}
else
{
new_node->previous = last;
last->next = new_node;
last = new_node;
}
}
void List::insert(Iterator iter, string s)
{
if (iter.position == NULL)
{
push_back(s);
return;
}
Node* after = iter.position;
Node* before = after->previous;
Node* new_node = new Node(s);
new_node->previous = before;
new_node->next = after;
after->previous = new_node;
if (before == NULL) // Insert at beginning
first = new_node;
else
before->next = new_node;
}
Iterator List::erase(Iterator iter)
{
assert(iter.position != NULL);
Node* remove = iter.position;
Node* before = remove->previous;
Node* after = remove->next;
if (remove == first)
first = after;
else
before->next = after;
if (remove == last)
last = before;
else
after->previous = before;
delete remove;
Iterator r;
r.position = after;
r.container = this;
return r;
}
Iterator List::begin()
{
Iterator iter;
iter.position = first;
iter.container = this;
return iter;
}
Iterator List::end()
{
Iterator iter;
iter.position = NULL;
iter.container = this;
return iter;
}
Iterator::Iterator()
{
position = NULL;
container = NULL;
}
string Iterator::get() const
{
assert(position != NULL);
return position->data;
}
void Iterator::next()
{
assert(position != NULL);
position = position->next;
}
void Iterator::previous()
{
assert(position != container->first);
if (position == NULL)
position = container->last;
else
position = position->previous;
}
bool Iterator::equals(Iterator b) const
{
return position == b.position;
}
int main()
{
List staff;
Iterator pos;
staff.push_front("Tom");
staff.push_front("Dick");
staff.push_front("Harry");
staff.push_front("Juliet");
cout
for (pos = staff.begin(); !pos.equals(staff.end()); pos.next())
cout
// reverse the list
cout
staff.reverse();
for (pos = staff.begin(); !pos.equals(staff.end()); pos.next())
cout
// swap two elements
Iterator pos1, pos2;
pos1 = staff.begin();
pos2 = staff.begin();
pos2.next();
pos2.next();
staff.swap(pos1,pos2);
cout
Problem 1 (100pt): Using good coding practices, add the following methods to Linked List class List. You may use the provided list.cpp as reference. (1) void List:.reverse() to reverse the nodes in a list without creating a new list object (2) void List:: push front (string s) to add an element to the beginning of a list (3) void List: : swap(Iterator in, Iterator i2) to swap two elements The main function is given in list.cpp and you are not allowed to modify it. The out- put should be exact as follows. Compile your code and run your program to check for compile-time errors and logic errors. Instructions: (10pt) Put all your code in one cpp file, named mylist.cpp, and submit it to ccle.ucla.edu. Write your code with good coding practices. Comment on your code to make it read- able and add description of files in the beginning to show your ownership. (90pt) Compile your code and run your program to check for compile-time errors and logic errors. Your output should follow the format as follows. Remember to check your homework with PIC lab desktop if you don't code with VS 2019. You may lose the majority of points if your code doesn't compile. ******* Initial List ******* Juliet Harry Dick Tom ******* Reverse List ******* Tom Dick Harry Juliet ******* After Swapping ******* Harry Dick Tom Juliet for (pos = staff.begin(); !pos.equals(staff.end()); pos.next())
cout
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
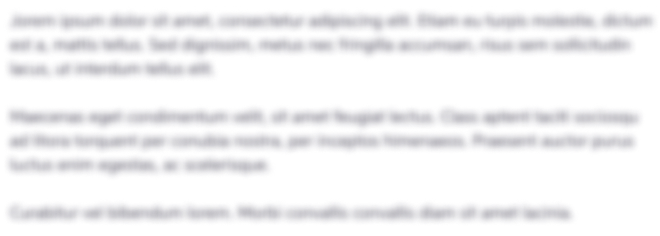
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started