Question
Video Store example CH5 Page 327 Data Structures Using C++ D.S MALIK videoListType uses the help of unorderedLinkedList which is provided in the files. You
Video Store example CH5 Page 327 Data Structures Using C++ D.S MALIK
videoListType uses the help of unorderedLinkedList which is provided in the files. You are asked to implement it with the help of STL library and update the given member functions for the operations such as check video availability, check-in and check-out of videos, search and other member functions accordingly.
videoListType.h //copy code
videoListTypeImp.cpp //copy code
unorderedLinkedList.h //copy code
#ifndef H_UnorderedLinkedList
#define H_UnorderedLinkedList
#include "linkedList.h"
using namespace std;
template
class unorderedLinkedList: public linkedListType
{
public:
bool search(const Type& searchItem) const;
//Function to determine whether searchItem is in the list.
//Postcondition: Returns true if searchItem is in the list,
// otherwise the value false is returned.
void insertFirst(const Type& newItem);
//Function to insert newItem at the beginning of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the beginning of the list, last points to
// the last node, and count is incremented by 1.
//
void insertLast(const Type& newItem);
//Function to insert newItem at the end of the list.
//Postcondition: first points to the new list, newItem is
// inserted at the end of the list, last points to the
// last node, and count is incremented by 1.
void deleteNode(const Type& deleteItem);
//Function to delete deleteItem from the list.
//Postcondition: If found, the node containing deleteItem
// is deleted from the list. first points to the first
// node, last points to the last node of the updated
// list, and count is decremented by 1.
};
template
bool unorderedLinkedList
search(const Type& searchItem) const
{
nodeType
bool found = false;
current = linkedListType
/ode in the list
while (current != NULL && !found) //search the list
if (current->info == searchItem) //searchItem is found
found = true;
else
current = current->link; //make current point to
//the next node
return found;
}//end search
template
void unorderedLinkedList
{
nodeType
newNode = new nodeType
newNode->info = newItem; //store the new item in the node
newNode->link = linkedListType
linkedListType
//actual first node
linkedListType
if (linkedListType
//the last node in the list
linkedListType
}//end insertFirst
template
void unorderedLinkedList
{
nodeType
newNode = new nodeType
newNode->info = newItem; //store the new item in the node
newNode->link = NULL; //set the link field of newNode
//to NULL
if (linkedListType
//both the first and last node
{
linkedListType
linkedListType
linkedListType
}
else //the list is not empty, insert newNode after last
{
linkedListType
linkedListType
//last node in the list
linkedListType
}
}//end insertLast
template
void unorderedLinkedList
{
nodeType
nodeType
bool found;
if (linkedListType
cout
else
{
if (linkedListType
{
current = linkedListType
linkedListType
linkedListType
if (linkedListType
linkedListType
delete current;
}
else //search the list for the node with the given info
{
found = false;
trailCurrent = linkedListType
//to the first node
current = linkedListType
//the second node
while (current != NULL && !found)
{
if (current->info != deleteItem)
{
trailCurrent = current;
current = current-> link;
}
else
found = true;
}//end while
if (found) //Case 3; if found, delete the node
{
trailCurrent->link = current->link;
linkedListType
if (linkedListType
//was the last node
linkedListType
//of last
delete current; //delete the node from the list
}
else
cout
}//end else
}//end else
}//end deleteNode
#endif
1 video ListType.h 4 5 6 7 8 #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
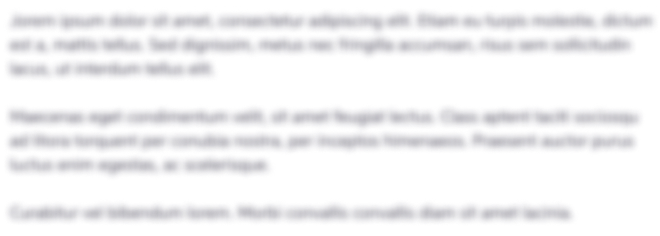
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started