Question
Visual Basic. My code is not calculating taxes for a single person (see screenshot). It will calculate for a married person but the NetPay is
Visual Basic. My code is not calculating taxes for a single person (see screenshot). It will calculate for a married person but the NetPay is incorrect See screenshot). It may be connected to the health insurance cost. My code: Private Sub BtnClear_Click(sender As Object, e As EventArgs) Handles BtnClear.Click, txtName.TextChanged, txtHoursWrk.TextChanged, txtRate.TextChanged, txtDepend.TextChanged, rbtSingle.TextChanged, rbtMarried.TextChanged, ckInsurance.TextChanged 'clearall textboxes
lblGross.Text = String.Empty lblHealth.Text = String.Empty lblTax.Text = String.Empty lblNetPay.Text = String.Empty
End Sub
Private Sub CancelKeys(sender As Object, e As KeyPressEventArgs) Handles txtRate.KeyPress, txtDepend.KeyPress, txtHoursWrk.KeyPress 'allows the text box to accept only numbers and the Backspace
If (e.KeyChar < "0" OrElse e.KeyChar > "9") AndAlso e.KeyChar <> ControlChars.Back Then 'cancel the key e.Handled = True End If End Sub
Private Function CalculateGrossPay(ByVal Pay As Decimal) As Decimal
'Calculate gross pay
Dim grossPay As Decimal Dim hourlyRate As Decimal Dim hrsWrk As Decimal
Decimal.TryParse(txtHoursWrk.Text, hrsWrk) Decimal.TryParse(txtRate.Text, hourlyRate)
If hourlyRate >= 0 And hourlyRate >= 0 And hrsWrk <= 40 Then grossPay = hourlyRate * 40 ElseIf hourlyRate >= 0 And hrsWrk > 40 Then grossPay = 40 * hourlyRate + (hrsWrk - 40) * hourlyRate * 1.5 End If
Return grossPay
End Function
Private Function CalculateInsuranceDeduction(ByVal InsuranceStatus As String) As Double 'calculate Insurance deduction
Dim healthCost As Double
If ckInsurance.Checked Then
If rbtSingle.Checked Then 'standard $60 medical $75 healthCost = 135 Else healthCost = 60
If rbtMarried.Checked Then '$ medical 150 medical healthCost = 250 Else healthCost = 100 End If End If
End If
Return healthCost End Function
Private Function CalculateTax(ByVal deduction As Decimal) As Double
Dim taxablePay As Decimal Dim tax As Decimal Dim NumberDependents As Integer Dim grossPay As Decimal
Integer.TryParse(txtDepend.Text, NumberDependents) grossPay = CalculateGrossPay(grossPay)
If rbtMarried.Checked = True Then taxablePay = grossPay - 150 - 70 * NumberDependents If ckInsurance.Checked Then taxablePay = taxablePay - 100 If taxablePay > 800 Then tax = (taxablePay - 800) * 0.35 + (800 - 500) * 0.25 + (500 - 200) * 0.15 + 200 * 0.1 ElseIf taxablePay > 500 Then tax = (taxablePay - 500) * 0.25 + (500 - 200) * 0.15 + 200 * 0.1 ElseIf taxablePay > 200 Then tax = (taxablePay - 200) * 0.15 + 200 * 0.1 Else tax = taxablePay * 0.1 End If End If Else
If rbtSingle.Checked = True Then taxablePay = grossPay - 75 - 70 * NumberDependents If ckInsurance.Checked Then taxablePay = taxablePay - 60
If taxablePay > 400 Then tax = (taxablePay - 400) * 0.35 + (400 - 250) * 0.25 + (250 - 100) * 0.15 + 100 * 0.1 ElseIf taxablePay > 250 Then tax = (taxablePay - 250) * 0.25 + (250 - 100) * 0.15 + 100 * 0.1 ElseIf taxablePay > 100 Then tax = (taxablePay - 100) * 0.15 + 100 * 0.1 Else tax = taxablePay * 0.1
End If End If End If End If Return tax
End Function Private Sub BtnCalculate_Click(sender As Object, e As EventArgs) Handles BtnCalculate.Click
Dim netPay As Decimal Dim Gross As Decimal Dim Health As Decimal Dim Tax As Decimal
Gross = CalculateGrossPay(Gross) Health = CalculateInsuranceDeduction(Health) Tax = CalculateTax(Tax)
'calculate net pay netPay = Gross - Health - Tax
' display results lblGross.Text = Gross.ToString("N2") lblHealth.Text = Health.ToString("N2") lblTax.Text = Tax.ToString("N2") lblNetPay.Text = netPay.ToString("N2")
End Sub Private Sub BtnExit_Click(sender As Object, e As EventArgs) Handles BtnExit.Click Me.Close() End Sub
End Class
The requirement Create a program that will calculate employees weekly pay as follows:
Inputs:
)Name
2)Hourly
3)Rate of Pay ($ / hour)
4)Marital Status (Married, Single)
5)# of Dependents
6)Healthcare(Yes or No)
Outputs:
1)Gross pay (Hours over 40 are paid at time and )
2)Health Insurance Amount Single Medical $60 Standard $75 Married Medical $ 100 Standard $150
3)Federal Tax, calculated as follows:
A. From gross taxable pay subtract $70 for each dependent
B. From value in A subtract appropriate value in Standard Deduction Column from table
below to give taxable gross.
C.Tax at rate of 10% appropriate amount in 10% Column from table below.
D. Tax at rate of 15% appropriate amount in 15% Column from table below.
E. Tax at rate of 25% appropriate amount in 25% Column from table below.
F. Tax at rate of 35% remaining amount (if any) in 35% Column from table below.
4) Net Pay (Gross Pay Health Care Deduction (if any)Federal Tax).
5)If any of the inputs are unreasonable, tell user with a message and force reentry of the data.
Additional Requirements:
1)Name any control that is referenced in code with a meaningful name
2)Use functions for major tasks
a.Calculate Gross Pay
b.Calculate Health Deductions
c.Calculate Tax
3) Other than constants, do NOT use global variables(e.g You need to pass variable between functions through the argument list and the return value)
.4)Comment each function and variable and major sections of code.
Assume that variable
GrossPay
is the calculated total pay for individual. All the constants are from the
table on the previous page:
If married
TaxablePay = GrossPay15070.0 * NumberDependents
if healthcare
TaxablePay = TaxablePay100
if TaxablePay > 800
Tax =(TaxablePay-800) * .35 + (800-500) * .25 + (500-200) * .15 +200 * .10
else if TaxablePay > 500
Tax = (TaxablePay-500) * .25 + (500200) * .15 +200 * .10
else if TaxablePay > 200
Tax = (TaxablePay-200) * .15 +200 * .10
else
Tax = TaxablePay * .10
else
TaxablePay = GrossPay7570.0 * NumberDependents
if healthcare
TaxablePay = TaxablePay60if TaxablePay > 400
Tax = (TaxablePay-400) * .35 + (400-250) * .25 + (250-100) * .15 +100 * .10
else if TaxablePay > 250Tax = (TaxablePay-250) * .25 + (250100) * .15 +100 * .10
else if TaxablePay > 100
Tax = (TaxablePay-100) * .15 +100 * .10
else
Tax = TaxablePay * .10
xample
s
:
1.A married employee with 45 hours at $20.00/hour, 2 dependents and health care:
A.Gross Pay:40 *20.00 + 5 *20.00* 1.5= $950.00
B.Minus Standard Deduction of $150.00 = 800.00
C.Minus Medical Deduction of $100.00= $700.00
D.Minus 2 dependents (@$70) = $560.00
E.Tax at 10% Bracket= 200 * .10 = $20.00
F. Taxat 15% Bracket($500$200)= 300 * .15 = $45.00
G.Tax at 25% Bracket($560$500)=$60.00* .25= $15.00
H.Total Tax = $20 + $45 + $15.00= $80.00
I.Net Pay = 950.00-$100.00 (Medical)-$80.00(Tax) = $770.00
2. A single employee with 45 hours at $20.00/hour, 1 dependent and no health care:
A.Gross Pay: 40* 20.00 + 5 * 20.00 * 1.5 = $950.00
B.Minus Standard Deduction of $75.00 = 875.00
C.Minus 1 dependent (@$70) = $805.00
D.Tax at 10% Bracket = 100 * .10 = $10.00
E.Tax at 15% Bracket ($250$100) =$150.00* .15 = $22.5
F.Tax at 25% Bracket ($400$250) = $150.00 *.25 = $37.5
G.Tax at 35% Bracket ($805-$400) = $405 * .35 =$141.75H.
Total Tax = $10 + $22.50+ $37.50 + 141.75= $211.75I.
Net Pay = 950.00-$100.00 (Medical)-$80.00 (Tax) = $770.00
Step by Step Solution
There are 3 Steps involved in it
Step: 1
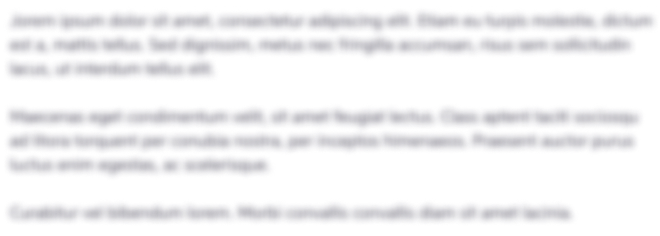
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started