Question
Visual C# chapter 9 question I am not sure how to fix my code to get it working MainForm.cs using System; using System.Collections.Generic; using System.ComponentModel;
Visual C# chapter 9 question I am not sure how to fix my code to get it working
MainForm.cs
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
namespace MainForm { public partial class MainForm : Form { private Employee employee1 = new Employee("Susan Meyers", "47899", "ccounting", "Vice President"); private Employee employee2 = new Employee("Mark Jones", "39119", "IT", "Programmer"); private Employee employee3 = new Employee("Joy Rogers", "81774", "Manufacturing", "Engineer");
private void MainForm_Load(object sender, EventArgs e) { InitializeComponent(); } }
private void btnDisplay_Click(object sender, EventArgs e) { textBoxEmployeeOne.Text = employee1.Name + "," + employee1.IdNumber + "," + employee1.Department + "," + employee1.Position;
textBoxEmployeeTwo.Text = employee2.Name + "," + employee2.IdNumber + "," + employee2.Department + "," + employee2.Position;
textBoxEmployeeThree.Text = employee3.Name + "," + employee3.IdNumber + "," + employee3.Department + "," + employee3.Position; }
private void btnExit_Click(object sender, EventArgs e) { this.Close(); } } }
Employee.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace Employee_Class {
//Employee Class Created public class Employee { public string Name { get; set; } public int IdNumber { get; set; } public string Department { get; set; } public string Position { get; set; }
//Constructor Created accecpting 4 arguments - name, id, department, position public Employee(string name, int id, string dept_name, string pos) { Name = name; IdNumber = id; Department = dept_name; Position = pos; }
//Constructor Created accecpting 4 arguments - name, id public Employee(int eid, string ename) { Name = ename; IdNumber = eid; Department = ""; Position = ""; }
//Constructor Created without any arguments public Employee() { Name = ""; Department = ""; Position = ""; IdNumber = 0; } }
designer.cs
namespace MainForm { partial class MainForm { ///
/// Required designer variable. ///
private System.ComponentModel.IContainer components = null;
///
/// Clean up any resources being used. ///
///true if managed resources should be disposed; otherwise, false. protected override void Dispose(bool disposing) { if (disposing && (components != null)) { components.Dispose(); } base.Dispose(disposing); }
#region Windows Form Designer generated code
///
/// Required method for Designer support - do not modify /// the contents of this method with the code editor. ///
private void InitializeComponent() { this.label1 = new System.Windows.Forms.Label(); this.label2 = new System.Windows.Forms.Label(); this.label3 = new System.Windows.Forms.Label(); this.textBoxEmployeeOne = new System.Windows.Forms.TextBox(); this.textBoxEmployeeTwo = new System.Windows.Forms.TextBox(); this.textBoxEmployeeThree = new System.Windows.Forms.TextBox(); this.btnDisplay = new System.Windows.Forms.Button(); this.btnExit = new System.Windows.Forms.Button(); this.label4 = new System.Windows.Forms.Label(); this.SuspendLayout(); // // label1 // this.label1.AutoSize = true; this.label1.Location = new System.Drawing.Point(341, 33); this.label1.Name = "label1"; this.label1.Size = new System.Drawing.Size(209, 32); this.label1.TabIndex = 0; this.label1.Text = "Employee Data"; // // label2 // this.label2.AutoSize = true; this.label2.Location = new System.Drawing.Point(41, 112); this.label2.Name = "label2"; this.label2.Size = new System.Drawing.Size(165, 32); this.label2.TabIndex = 1; this.label2.Text = "Employee 1"; // // label3 // this.label3.AutoSize = true; this.label3.Location = new System.Drawing.Point(41, 197); this.label3.Name = "label3"; this.label3.Size = new System.Drawing.Size(165, 32); this.label3.TabIndex = 2; this.label3.Text = "Employee 2"; // // textBoxEmployeeOne // this.textBoxEmployeeOne.Location = new System.Drawing.Point(320, 106); this.textBoxEmployeeOne.Name = "textBoxEmployeeOne"; this.textBoxEmployeeOne.Size = new System.Drawing.Size(347, 38); this.textBoxEmployeeOne.TabIndex = 3; // // textBoxEmployeeTwo // this.textBoxEmployeeTwo.Location = new System.Drawing.Point(320, 191); this.textBoxEmployeeTwo.Name = "textBoxEmployeeTwo"; this.textBoxEmployeeTwo.Size = new System.Drawing.Size(347, 38); this.textBoxEmployeeTwo.TabIndex = 4; // // textBoxEmployeeThree // this.textBoxEmployeeThree.Location = new System.Drawing.Point(320, 276); this.textBoxEmployeeThree.Name = "textBoxEmployeeThree"; this.textBoxEmployeeThree.Size = new System.Drawing.Size(347, 38); this.textBoxEmployeeThree.TabIndex = 5; // // btnDisplay // this.btnDisplay.Location = new System.Drawing.Point(205, 379); this.btnDisplay.Name = "btnDisplay"; this.btnDisplay.Size = new System.Drawing.Size(153, 59); this.btnDisplay.TabIndex = 6; this.btnDisplay.Text = "Display"; this.btnDisplay.UseVisualStyleBackColor = true; this.btnDisplay.Click += new System.EventHandler(this.btnDisplay_Click); // // btnExit // this.btnExit.Location = new System.Drawing.Point(504, 379); this.btnExit.Name = "btnExit"; this.btnExit.Size = new System.Drawing.Size(117, 59); this.btnExit.TabIndex = 7; this.btnExit.Text = "Exit"; this.btnExit.UseVisualStyleBackColor = true; this.btnExit.Click += new System.EventHandler(this.btnExit_Click); // // label4 // this.label4.AutoSize = true; this.label4.Location = new System.Drawing.Point(41, 282); this.label4.Name = "label4"; this.label4.Size = new System.Drawing.Size(165, 32); this.label4.TabIndex = 8; this.label4.Text = "Employee 3"; // // MainForm // this.AutoScaleDimensions = new System.Drawing.SizeF(240F, 240F); this.AutoScaleMode = System.Windows.Forms.AutoScaleMode.Dpi; this.AutoSize = true; this.ClientSize = new System.Drawing.Size(800, 450); this.Controls.Add(this.label4); this.Controls.Add(this.btnExit); this.Controls.Add(this.btnDisplay); this.Controls.Add(this.textBoxEmployeeThree); this.Controls.Add(this.textBoxEmployeeTwo); this.Controls.Add(this.textBoxEmployeeOne); this.Controls.Add(this.label3); this.Controls.Add(this.label2); this.Controls.Add(this.label1); this.Name = "MainForm"; this.Text = "9-4 PP"; this.Load += new System.EventHandler(this.MainForm_Load); this.ResumeLayout(false); this.PerformLayout();
}
#endregion
private System.Windows.Forms.Label label1; private System.Windows.Forms.Label label2; private System.Windows.Forms.Label label3; private System.Windows.Forms.TextBox textBoxEmployeeOne; private System.Windows.Forms.TextBox textBoxEmployeeTwo; private System.Windows.Forms.TextBox textBoxEmployeeThree; private System.Windows.Forms.Button btnDisplay; private System.Windows.Forms.Button btnExit; private System.Windows.Forms.Label label4; } }
4. Employee Class Wirite a class named Employee that has the following properties Name-The Name property holds the employee's name. IdNumber-The IdNumber property holds the employee's ID number. Department-The Department property holds the name of the department in which the employee works. Position-The Position property holds the employee's job title. The class should have the following overloaded constructors: A constructor that accepts the following values as arguments and assigns them to the appropriate properties: employee's name, employee's ID number, depart- ment, and position A constructor that accepts the following values as arguments and assigns them to the appropriate properties: employee's name and ID number. The Depart- ment and Position properties should be assigned an empty string () A parameterless constructor that assigns empty strings () to the Name, Department, and Position properties, and 0 to the IdNumber property In an application, create three Employee objects to hold the following data: Name Susan Meyers Mark Jones Joy Rogers ID Number Department Position 47899 39119 81774 Vice President Accounting IT Manufacturing Engineer Programmer The each employee on the screen. application should store this data in the three objects and display the data for
Step by Step Solution
There are 3 Steps involved in it
Step: 1
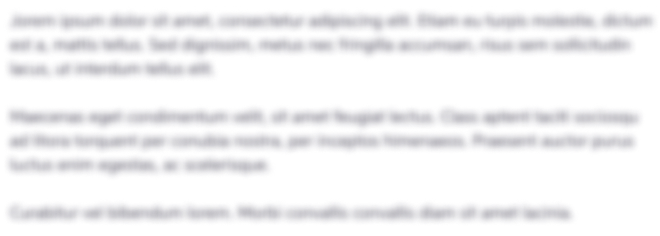
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started