Question
void deleteCourse (Graph graph, int iVertex) This is invoked due to the DELETE command. This will delete a course: Because of the delete, this course
void deleteCourse(Graph graph, int iVertex)
This is invoked due to the DELETE command. This will delete a course:
Because of the delete, this course will not be associated with any successors. (This also means that any successors will no longer have it as a prerequisite.)
Because of the delete, this course will not be associated with any prereqs. (This also means that no prereqs will have this course as a successor.)
Frees all edge nodes which reference this vertex.
The course is marked deleted in the vertex array.
h file
/********************************************************************** cs2123p5.h Purpose: Defines constants: max constants error constants warning constants boolean constants Defines typedef for EdgeNode - graph edge containing preq and successor vertex Vertex - contains course information (course number, name), existence boolean, successor list first node pointer, predecessor list first node pointer GraphImp - array of vertices and a count of them Graph - pointer to an allocated GraphImp PlanImp - tbd Defines function prototypes for functions used in pgm5 (recursive and non-recursive) Defines function prototypes for functions used in pgm6 Defines WARNING macro Notes: **********************************************************************/ /*** constants ***/ #define MAX_TOKEN 50 // Maximum number of actual characters for a token #define MAX_LINE_SIZE 100 // Maximum number of character per input line #define MAX_VERTICES 60 #define OVERFLOW_BEGIN 29 // begin of overflow area // Error constants (program exit values) #define ERR_COMMAND_LINE 900 // invalid command line argument #define ERR_ALGORITHM 903 // Unexpected error in algorithm #define ERR_TOO_MANY_COURSE 1 // Too many courses #define ERR_BAD_COURSE 3 // Bad Course Data #define ERR_BAD_PREREQ 4 // Bad Prereq Data #define ERR_MISSING_SWITCH "missing switch" #define ERR_EXPECTED_SWITCH "expected switch, found" #define ERR_MISSING_ARGUMENT "missing argument for" // exitUsage control #define USAGE_ONLY 0 // user only requested usage information #define USAGE_ERR -1 // usage error, show message and usage information // boolean constants #define FALSE 0 #define TRUE 1 // EdgeNode represents one edge in a graph typedef struct EdgeNode { int iPrereqVertex; // prereq int iSuccVertex; // successor struct EdgeNode *pNextEdge; // points to next edge } EdgeNode; typedef struct Vertex { char szCourseId[8]; // Course Identifier char szCourseName[21]; // Course Full Name char szDept[4]; // Department (e.g., CS, MAT) int bExists; // pgm6 DELETE command causes this to be set to TRUE // TRUE - this vertex exists, FALSE - deleted EdgeNode * prereqList; EdgeNode * successorList; int iSemesterLevel; // Which semester should this be taken int iHashChainNext; // pgm 6 extra credit int iDistSource; // Distance from a source } Vertex; // GraphImp of a double adjacency list graph typedef struct { int iNumVertices; // Number of vertices in the vertexM array Vertex vertexM[MAX_VERTICES]; // Array of vertices int iOverflowBegin; // The subscript of the first overflow entry // in the array of vertices. // Any subscript less than this value is in // the primary area. int iFreeHead; // Subscript of a free list of entries // in the overflow portion of the // graph's vertexM array } GraphImp; typedef GraphImp *Graph; // Degree Plan typedef struct { int semesterM[5][MAX_VERTICES]; // Array which has five rows for each semester. int bIncludeM[MAX_VERTICES]; // TRUE if this course is to be included in the plan } PlanImp; typedef PlanImp * Plan; // Prototypes // Recursive functions for program 5 int maxChain(Graph graph, int iVertex); void printTraversal(Graph graph, int iCourseVertex, int indent); void printLongChains(Graph graph, int iVertex, int pathM[], int iLevel, int iLongLength); int causesCycle(Graph graph, int iPrereqVertex, int iVertex); // Non-recursive for program 5 int findCourse(Graph graph, char szCourseId[]); void insertPrereq(Graph graph, int iPrereqVertex, int iCourseVertex); void printAllInList(Graph graph); void printOne(Graph graph, int iVertex); void printSources(Graph graph); void printSinks(Graph graph); Graph newGraph(); int insertCourse(Graph graph, char szCourseId[]); // Program 6 function for delete void deleteCourse (Graph graph, int iVertex); // Program 6 functions for Plan void doPlan(Graph graph, Plan plan); void setLevel(Graph g, Plan plan, int iVertex, int iLev); Plan newPlan(); // hash function for extra credit int hash(Graph g, char SzCourseId[]); void printHash(Graph g); void printChain(Graph g, int iVertex); // functions in most programs, but require modifications void processCommandSwitches(int argc, char *argv[], char **ppszCommandFileName); void exitUsage(int iArg, char *pszMessage, char *pszDiagnosticInfo); // Utility routines provided by Larry void ErrExit(int iexitRC, char szFmt[], ...); char * getToken(char *pszInputTxt, char szToken[], int iTokenSize); /* WARNING macro Parameters: I szFmt - a printf format I ... - a variable number of parameters corresponding to szFmt's format codes. Results: Prints "WARNING" and the value(s) specified by the szFmt. Notes: Since this generates multiple C statements, we surround them with a dummy do while(0) which only executes once. Notice that the dummy do while isn't ended with a ";" since the user of the macro naturally specifies a ";". Example: if (x != 0) WARNING("X must be blah blah"); else { // normal processing .... } If we used {} in the macro definition instead of the dummy do while(0), the generated code would have a bad ";": if (x != 0) { printf("\tWARNING: "); printf("X must be blah blah"); printf(" "); } ; // yuck, bad ";" causing the compiler to not understand the else else { // normal processing .... } */ #define WARNING(szFmt, ...) do { \ printf("\tWARNING: "); \ printf(szFmt, __VA_ARGS__); \ printf(" "); \ } while (0)
/********************************************
Input file
COURSE CS1083 Intro I COURSE CS1713 Intro II PREREQ CS1083 COURSE CS2123 Data Structures PREREQ CS1713 PRTONE CS2123 PRTONE CS1713 COURSE MAT1214 Calculus I COURSE MAT1224 Calculus II PREREQ MAT1214 COURSE MAT2233 Discrete Math PREREQ MAT1214 PREREQ CS1713 COURSE MAT3333 Math Found PREREQ MAT1224 PREREQ CS1713 COURSE CS3343 Analysis of Algo PREREQ CS2123 PREREQ MAT3333 PREREQ MAT2233 * * show some chains * PRTSUCC CS1083 MAXCHAIN CS1083 PRTLONGS CS1083 COURSE CS3423 Sys Pgm PREREQ CS2123 COURSE CS3433 Princ of Security PREREQ CS3423 COURSE CS3443 App Pgm PREREQ CS2123 COURSE CS3843 Comp Org PREREQ CS2123 COURSE CS3723 Pgm Lang PREREQ CS3443 PREREQ MAT2233 COURSE CS3733 Operating Systems PREREQ CS3423 PREREQ CS3443 PREREQ CS3843 PRTALL PRTSUCC CS1713 MAXCHAIN CS1713 PRTLONGS CS1713 PRTSUCC MAT1214 MAXCHAIN MAT1214 PRTLONGS MAT1214 * * Insert all the other courses * COURSE CS3743 Database Systems PREREQ CS3423 PREREQ CS3753 COURSE CS3753 Intro Data Sci PREREQ CS2123 PREREQ MAT2233 PREREQ MAT3333 COURSE CS3773 Software Engineering PREREQ CS3443 COURSE CS3793 Artificial Intel PREREQ CS3343 COURSE CS3853 Computer Arch PREREQ CS3843 PREREQ CS3423 COURSE CS3873 Networks PREREQ CS3843 COURSE CS4223 Bioinformatics PREREQ CS3753 COURSE CS4233 Computational Bio PREREQ CS3753 COURSE CS4353 Unix & Net Security PREREQ CS3433 COURSE CS4363 Cryptography PREREQ CS3343 PREREQ CS3433 COURSE CS4373 Data Mining PREREQ CS3743 COURSE CS4393 User Interfaces PREREQ CS3443 COURSE CS4413 Web PREREQ CS3423 COURSE CS4633 Simulation PREREQ CS3343 COURSE CS4633 Mobile Tech PREREQ CS3733 COURSE CS4663 Dist Cloud Security PREREQ CS3733 COURSE CS4673 Cyber Operations PREREQ CS4353 COURSE CS4713 Compiler Construct PREREQ CS3723 PREREQ CS3843 COURSE CS4723 Sw Valid & QA PREREQ CS3773 COURSE CS4733 Project Mgt PREREQ CS3773 COURSE CS4743 Enterprise Sw Eng PREREQ CS3773 COURSE CS4783 Adv Sw Eng PREREQ CS3773 COURSE CS4823 Parallel Programming PREREQ CS3343 PREREQ CS3423 COURSE CS4843 Cloud Computing PREREQ CS3423 PREREQ CS3853 COURSE CS4973 Big Data PREREQ CS3743 * * End of all the courses * * * cycle stuff * PRTSUCC CS3443 COURSE CS3443 Appl Pgm PREREQ MAT1214 PREREQ CS4713 * * Final Output from program 5 * PRTSUCC CS1083 PRTSUCC MAT1214 PRTSOURCES PRTSINKS PRTALL MAXCHAIN CS2123 PRTLONGS CS2123 * * Program 6 * PLAN CS1083 PLAN CS1713 PLAN CS2123 PLAN MAT2233 PLAN CS3733 PLAN MAT3333 PLAN CS3343 PLAN CS3423 PLAN CS3443 PLAN CS3723 PLAN CS3843 PLAN CS3853 PLAN MAT1214 PLAN MAT1224 * * Add the Data Concentration - CS3753 CS3743 CS4223 CS4233 CS4373 CS4973 * * PLAN CS3753 PLAN CS3743 PLAN CS4223 PLAN CS4233 PLAN CS4373 PLAN CS4973 * Get the plan * DOPLAN HALT * * Delete CS3853 * PRTONE CS3853 DELETE CS3853 PRTONE CS3853 PRTALL
Step by Step Solution
There are 3 Steps involved in it
Step: 1
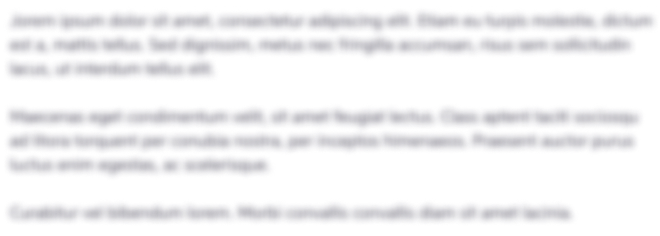
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started