Question
We have now discussed quite a few sorts, and their performance metrics. For this assignment you are going to write an insertion sort in entirety
We have now discussed quite a few sorts, and their performance metrics. For this assignment you are going to write an insertion sort in entirety you will have available the same SortTester class that will provide the storage, comparison and swap functionality that you had on the last program. The assignment is to write an insertion sort and modify it such that instead of using a backwards linear search to find the location that the insertion elements belongs, you will use a binary search. The number of comparisons will be tracked in the SortTester to see if your comparisons are in the range that indicates you have adjusted the search. There is a bonus for this program if you can implement the algorithm in a way that maintains stability. Your swaps will be monitored and if your sort is stable, elements with the same value will not get swapped and you will receive the extra credit - you have to get full credit for all the other parts in order to be eligible for the extra credit.
main.cpp
#include SortTester.h #include SortTester.cpp #include
Step by Step Solution
There are 3 Steps involved in it
Step: 1
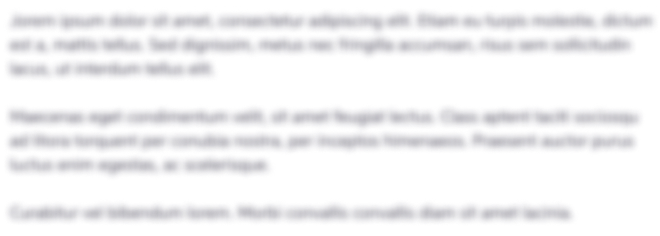
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started