Question
WE MUST NOT USE MAP.ENTRY Create a Class called CharacterCounter that provides char counts for any text file (file used as constructor parameter). Use a
WE MUST NOT USE MAP.ENTRY Create a Class called CharacterCounter that provides char counts for any text file (file used as constructor parameter). Use a custom data structure CharInt.java that implements Comparable and Comparator, to provide the correct sorting as described below (i.e. TreeMap is not sorted correctly). SUBMIT: CharacterCounter.java CharInt.java A good place to start is with the example from the text (counting words) Vocabulary3.zipView in a new window and/or complete Exercise 11.15 in our text (counting int occurrences) for some good starting examples. In the case of Exercise 11.15, however, we can get a TreeMap, which is sorted alphabetically, so the first three elements could be a,b,c regardless of their counts. We desire the sorting to be by the count of those characters in the text file! One easy way to do this is to write a CharInt Class with two fields, Character and Integer, which implements Comparable to sort via count instead of char. When you have a Set (or Map) with a Character key, it will be alphabetical, and we require sorting by count, thus the need for this "custom" CharInt Class. Requirements: DO NOT use Map.Entry nor the entrySet() method (***below) Keep with the Map concept for toString() output like { c=i } where c is the Character and i is the Integer count of c Ignore case for alphabetic characters, report just a-z (meaning a-z are the same as A-Z). Other characters such as tabs, returns, line feeds, spaces (there will be many spaces) also count. Use the constructor to read a data file (see testing code below) Provide overloaded (char or int or default) getCounts method that returns a Collection of character counts (see test code below: char returns count of that char, int returns highest of that many or lowest when negative, default() returns the entire Collection). Test code below: Collection defined: use any List, Set, or Map that makes this easiest for you. Scanner data = new Scanner(new File("moby.txt")); CharacterCounter working = new CharacterCounter(data); // reads data from file, all the code to read file System.out.println(working.getCounts(3)); // returns highest 3 counts e.g. =199250, e=115002, t=86488... // I counted 199250 space characters in moby.txt file, yes 'e' is the most common char as Wikipedia predicts. System.out.println(working.getCounts('a')); // returns int count of char 'a' (zero if not present) System.out.println(working.getCounts(-3)); // returns a Collection of lowest 3 counts, e.g. $=2, [=2, ]=2 System.out.println(working.getCounts()); // returns the ENTIRE Collection like a Map (like e=9, etc.) crazy long... Hints: Use a small test file (like 10 characters) where you know the answers for initial testing. Especially because that's how your instructor will grade these.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
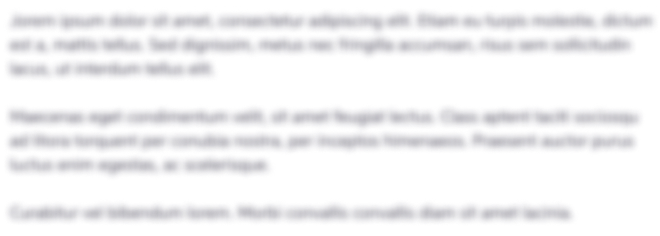
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started